复制文件中的一行时,可以使用Python中的文件操作功能来读取文件、找到需要复制的行,并将其写入目标文件。
在处理文件操作时,Python 提供了多种方法,包括使用内置的 open
函数及其相关方法,或者使用更高级的文件处理库如 shutil
。在这篇文章中,我们将详细介绍如何使用这些工具来完成复制文件中的一行这一任务。
一、使用内置的文件操作函数
1. 读取文件内容
要复制文件中的某一行,我们首先需要读取文件内容。Python 提供了 open
函数,可以方便地打开文件并读取其内容。
def read_file(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
return lines
2. 选择需要复制的行
读取文件内容后,我们可以通过行号来选择需要复制的行。例如,如果我们想复制文件中的第3行:
def get_specific_line(lines, line_number):
return lines[line_number - 1] # 注意行号从1开始,索引从0开始
3. 写入目标文件
我们可以使用 open
函数以写入模式打开目标文件,并将选择的行写入其中:
def write_to_file(file_path, line):
with open(file_path, 'a') as file: # 以追加模式打开文件
file.write(line + '\n')
4. 完整代码示例
将上述步骤结合起来,形成一个完整的函数:
def copy_line_from_file(source_file, target_file, line_number):
lines = read_file(source_file)
specific_line = get_specific_line(lines, line_number)
write_to_file(target_file, specific_line)
示例使用
source_file_path = 'source.txt'
target_file_path = 'target.txt'
line_number_to_copy = 3
copy_line_from_file(source_file_path, target_file_path, line_number_to_copy)
二、使用 shutil
库
shutil
是一个高级文件操作库,提供了更多的文件处理功能。虽然 shutil
主要用于文件复制和移动,但也可以通过结合基本的文件操作来实现复制文件中的某一行。
import shutil
def copy_specific_line_with_shutil(source_file, target_file, line_number):
with open(source_file, 'r') as src, open(target_file, 'a') as tgt:
for current_line_number, line in enumerate(src, start=1):
if current_line_number == line_number:
tgt.write(line)
break
示例使用
source_file_path = 'source.txt'
target_file_path = 'target.txt'
line_number_to_copy = 3
copy_specific_line_with_shutil(source_file_path, target_file_path, line_number_to_copy)
三、错误处理和边界检查
在实际应用中,我们需要考虑文件是否存在、行号是否有效等问题。以下是一个更加健壮的实现,包括错误处理和边界检查:
def copy_line_with_error_handling(source_file, target_file, line_number):
try:
with open(source_file, 'r') as src:
lines = src.readlines()
if line_number < 1 or line_number > len(lines):
raise ValueError("Invalid line number.")
specific_line = lines[line_number - 1]
with open(target_file, 'a') as tgt:
tgt.write(specific_line + '\n')
except FileNotFoundError:
print(f"Error: The file {source_file} does not exist.")
except ValueError as ve:
print(f"Error: {ve}")
示例使用
source_file_path = 'source.txt'
target_file_path = 'target.txt'
line_number_to_copy = 3
copy_line_with_error_handling(source_file_path, target_file_path, line_number_to_copy)
四、总结
复制文件中的一行在Python中可以通过多种方法实现,本文介绍了使用内置文件操作函数和 shutil
库的方法。通过这些步骤,我们可以轻松地读取文件、选择特定行并将其写入目标文件。在实际应用中,加入错误处理和边界检查可以提高代码的健壮性,确保程序在各种情况下都能正常运行。
无论是简单的文件操作还是复杂的文件处理任务,Python 都提供了丰富的工具和方法,帮助我们高效地完成工作。希望这篇文章能为你在处理文件操作时提供有用的参考。
相关问答FAQs:
如何在Python中复制特定行到另一个文件?
要在Python中复制特定行,可以使用文件操作方法读取源文件的内容,然后将所需行写入目标文件。你可以使用with open
语句来确保文件在操作后被正确关闭。示例代码如下:
with open('source.txt', 'r') as source_file:
lines = source_file.readlines()
with open('destination.txt', 'w') as dest_file:
dest_file.write(lines[line_number]) # line_number是你想复制的行的索引
这样可以将指定的行从源文件复制到目标文件。
如何选择性地复制多行?
如果你需要复制多行,可以在读取源文件时使用列表切片。假设你想复制从第2行到第4行,可以使用以下代码:
with open('source.txt', 'r') as source_file:
lines = source_file.readlines()
with open('destination.txt', 'w') as dest_file:
dest_file.writelines(lines[1:4]) # 复制第2到第4行
此方法会将指定范围内的多行写入目标文件。
如果文件很大,如何提高复制效率?
处理大文件时,可以逐行读取并写入,从而避免一次性加载整个文件到内存中。使用以下代码可以提高效率:
with open('source.txt', 'r') as source_file, open('destination.txt', 'w') as dest_file:
for current_line_number, line in enumerate(source_file):
if current_line_number in {1, 2, 3}: # 替换成你想复制的行号
dest_file.write(line)
这种方式适合处理大文件,因为它只在内存中保留一行数据。
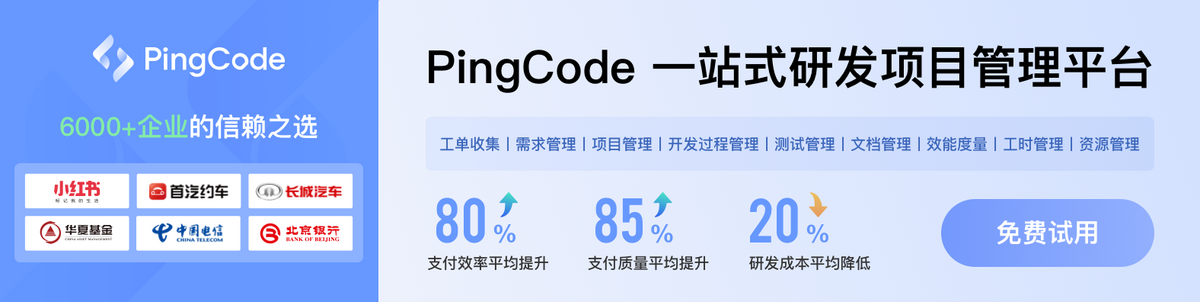