使用Python进行写操作时,去掉标点符号的方法有多种,如使用正则表达式、string库、手动遍历字符等。其中,使用正则表达式是最为高效和常用的方式。正则表达式不仅能够高效地匹配和替换字符,还可以灵活地处理复杂的字符串操作。接下来,我们将详细介绍如何在Python写操作中去掉标点符号,并提供一些示例代码。
一、使用正则表达式去掉标点符号
正则表达式(Regular Expressions)是用于匹配字符串中字符组合的强大工具。在Python中,可以使用re
模块来处理正则表达式。以下是使用正则表达式去掉标点符号的详细步骤:
1. 导入正则表达式模块
首先需要导入re
模块:
import re
2. 定义一个去掉标点符号的函数
接下来,我们定义一个函数,用于去掉字符串中的标点符号:
def remove_punctuation(text):
# 正则表达式模式,匹配所有标点符号
pattern = r'[^\w\s]'
# 使用re.sub()函数替换标点符号
clean_text = re.sub(pattern, '', text)
return clean_text
3. 测试函数
测试一下这个函数是否能够正确去掉标点符号:
text = "Hello, world! This is a test."
clean_text = remove_punctuation(text)
print(clean_text) # 输出:Hello world This is a test
二、使用string库去掉标点符号
Python的string
库中包含了一个常量string.punctuation
,它包含了所有的标点符号。我们可以利用这个常量来去掉字符串中的标点符号。
1. 导入string库
import string
2. 定义一个去掉标点符号的函数
def remove_punctuation(text):
# 创建一个翻译表,将所有标点符号映射为空字符
translator = str.maketrans('', '', string.punctuation)
clean_text = text.translate(translator)
return clean_text
3. 测试函数
text = "Hello, world! This is a test."
clean_text = remove_punctuation(text)
print(clean_text) # 输出:Hello world This is a test
三、手动遍历字符去掉标点符号
虽然正则表达式和string
库的方法更为高效,但有时我们可能需要手动遍历字符串中的每个字符,去掉标点符号。以下是这种方法的实现:
1. 定义一个去掉标点符号的函数
def remove_punctuation(text):
# 定义标点符号集合
punctuation = '''!()-[]{};:'"\,<>./?@#$%^&*_~'''
clean_text = ""
for char in text:
if char not in punctuation:
clean_text += char
return clean_text
2. 测试函数
text = "Hello, world! This is a test."
clean_text = remove_punctuation(text)
print(clean_text) # 输出:Hello world This is a test
四、在写操作中去掉标点符号
在实际应用中,去掉标点符号的操作往往与文件读写操作结合在一起。以下是一个示例,展示如何从文件中读取文本,去掉标点符号后,再写入另一个文件。
1. 定义一个函数,去掉文件内容中的标点符号
import re
def remove_punctuation_from_file(input_file, output_file):
with open(input_file, 'r') as file:
text = file.read()
clean_text = re.sub(r'[^\w\s]', '', text)
with open(output_file, 'w') as file:
file.write(clean_text)
2. 测试函数
假设我们有一个名为input.txt
的文件,内容如下:
Hello, world! This is a test.
Let's see if this works.
我们希望将处理后的内容写入output.txt
文件中:
remove_punctuation_from_file('input.txt', 'output.txt')
运行上述代码后,output.txt
的内容将变为:
Hello world This is a test
Lets see if this works
五、总结
在Python写操作中去掉标点符号的方法有多种,常用的有使用正则表达式、string库和手动遍历字符。其中,正则表达式方法最为高效和灵活,适用于处理复杂的字符串操作。使用string库的方法也很简洁,而手动遍历字符的方法适用于特定需求的场景。在实际应用中,我们可以将这些方法与文件读写操作结合起来,实现对文件内容的处理。希望本文能帮助你在Python编程中更好地处理标点符号问题。
相关问答FAQs:
在Python中,如何有效地去掉字符串中的所有标点符号?
要去掉字符串中的标点符号,可以使用str.translate()
方法结合str.maketrans()
函数。以下是一个简单的示例:
import string
text = "Hello, world! This is an example."
# 创建一个翻译表,将所有标点符号映射到None
translator = str.maketrans('', '', string.punctuation)
cleaned_text = text.translate(translator)
print(cleaned_text) # 输出:Hello world This is an example
这种方法可以高效地去除文本中的所有标点符号,适用于大多数情况。
使用正则表达式去掉标点符号的方式有哪些?
正则表达式是处理文本的强大工具,可以方便地去掉标点符号。使用re
模块的re.sub()
函数可以实现这一点。示例如下:
import re
text = "Hello, world! This is an example."
cleaned_text = re.sub(r'[^\w\s]', '', text)
print(cleaned_text) # 输出:Hello world This is an example
在这个例子中,[^\w\s]
表示匹配所有非字母数字和非空格的字符,从而有效去掉所有标点符号。
在处理文件时,如何去掉读取内容中的标点符号?
当需要处理文件内容时,可以先读取文件内容,再使用上述方法去掉标点符号。以下是一个示例:
import string
with open('example.txt', 'r') as file:
text = file.read()
translator = str.maketrans('', '', string.punctuation)
cleaned_text = text.translate(translator)
with open('cleaned_example.txt', 'w') as file:
file.write(cleaned_text)
这种方式能够有效清洗文件中的文本,使其更适合后续的数据处理或分析。
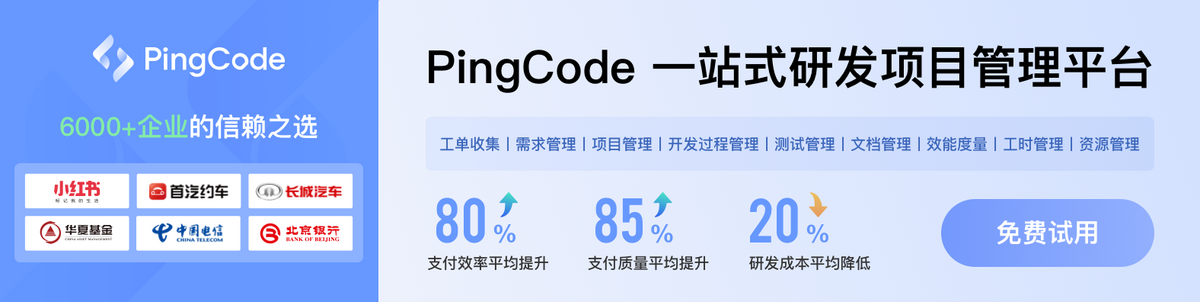