在Python中,有几种方法可以让程序只执行一次:使用锁文件、设置全局变量、使用数据库或缓存来记录执行状态。这些方法各有优劣,最常用且简单的方法是使用锁文件。 锁文件是一种标记文件,用于在文件系统中记录某个操作是否已经执行过。以下将详细介绍如何使用锁文件来实现Python程序只执行一次。
一、锁文件
使用锁文件是让Python程序只执行一次的常见方法之一。锁文件是一种简单的标记机制,通过创建一个特定文件来记录程序是否已经执行过。
1. 创建锁文件
在程序开始时检查锁文件是否存在,如果不存在则创建并执行程序逻辑;如果存在则跳过执行。
import os
lock_file = "program.lock"
if not os.path.exists(lock_file):
with open(lock_file, 'w') as f:
f.write("This program has been executed.")
# 执行程序逻辑
print("Program is running...")
else:
print("Program has already been executed.")
2. 清理锁文件
在某些情况下,你可能希望在特定条件下删除锁文件,以便程序可以再次执行。
import os
lock_file = "program.lock"
if not os.path.exists(lock_file):
with open(lock_file, 'w') as f:
f.write("This program has been executed.")
# 执行程序逻辑
print("Program is running...")
# 条件满足时清理锁文件
if some_condition:
os.remove(lock_file)
else:
print("Program has already been executed.")
二、全局变量
另一种方法是使用全局变量来记录程序的执行状态。虽然这种方法适用于简单的脚本,但在多进程或多线程环境中可能不太可靠。
1. 使用全局变量记录执行状态
has_run = False
def run_once():
global has_run
if not has_run:
# 执行程序逻辑
print("Program is running...")
has_run = True
else:
print("Program has already been executed.")
run_once()
run_once()
2. 多线程环境中的使用
在多线程环境中,可以使用线程锁来确保全局变量的修改是线程安全的。
import threading
has_run = False
lock = threading.Lock()
def run_once():
global has_run
with lock:
if not has_run:
# 执行程序逻辑
print("Program is running...")
has_run = True
else:
print("Program has already been executed.")
threads = [threading.Thread(target=run_once) for _ in range(10)]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
三、数据库或缓存
对于更复杂的应用,可以使用数据库或缓存来记录程序的执行状态。这种方法适用于需要在分布式环境中确保唯一执行的情况。
1. 使用SQLite数据库
SQLite是一种轻量级的嵌入式数据库,适合用于记录程序的执行状态。
import sqlite3
def create_table():
conn = sqlite3.connect('program.db')
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS execution_status (id INTEGER PRIMARY KEY, has_run BOOLEAN)''')
conn.commit()
conn.close()
def check_status():
conn = sqlite3.connect('program.db')
c = conn.cursor()
c.execute('SELECT has_run FROM execution_status WHERE id = 1')
result = c.fetchone()
conn.close()
return result
def update_status():
conn = sqlite3.connect('program.db')
c = conn.cursor()
c.execute('INSERT OR REPLACE INTO execution_status (id, has_run) VALUES (1, 1)')
conn.commit()
conn.close()
create_table()
status = check_status()
if status is None or not status[0]:
# 执行程序逻辑
print("Program is running...")
update_status()
else:
print("Program has already been executed.")
2. 使用Redis缓存
Redis是一种高性能的内存数据库,可以用于记录程序的执行状态。
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
if not r.get('has_run'):
# 执行程序逻辑
print("Program is running...")
r.set('has_run', '1')
else:
print("Program has already been executed.")
四、总结
在本文中,我们探讨了几种让Python程序只执行一次的方法,包括使用锁文件、全局变量、数据库和缓存。每种方法都有其适用的场景和优缺点。
锁文件是一种简单且常用的方法,适合于单机环境;全局变量适用于简单脚本,但在多线程或多进程环境中需要额外的处理;数据库和缓存方法适用于更复杂和分布式的应用环境。
选择合适的方法取决于具体的应用场景和需求。希望这篇文章对你有所帮助!
相关问答FAQs:
如何确保我的Python脚本在多次运行时只执行一次?
为了确保Python程序只执行一次,可以使用锁文件的机制。创建一个特定的文件作为标识,如果文件存在,则程序不再执行;如果不存在,则创建该文件并继续执行。完成后,记得在程序结束时删除该锁文件,这样下次运行时可以正常执行。
在多线程环境中,如何确保Python程序的单次执行?
在多线程环境中,可以使用线程锁(threading.Lock)来确保同一时间只有一个线程可以执行程序的关键部分。通过在代码块前后加锁,确保在执行期间其他线程无法进入,从而达到只执行一次的效果。
是否有第三方库可以帮助实现Python程序的单次执行?
是的,可以使用一些第三方库,如singleton-decorator
或者pyngrok
,它们可以帮助你轻松实现单例模式,确保Python程序只执行一次。这些库提供了简单的装饰器,可以直接应用于你的主函数,使得程序在多次调用时不会重复执行。
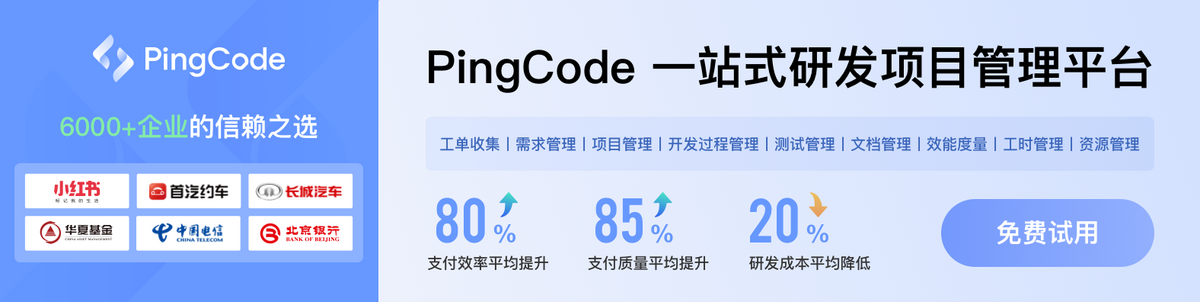