Python Qt 如何将很多张图片平铺
在使用Python和Qt进行应用开发时,将很多张图片平铺是一个常见需求,尤其在图像浏览器或相册应用中。为实现这一功能,主要有以下几个步骤:创建一个包含图片的窗口、使用QGridLayout布局管理器、优化图片加载和显示。本文将详细介绍这些步骤,并分享一些实用的技巧和经验。
一、创建一个包含图片的窗口
为了展示图片,我们首先需要创建一个主窗口,并在其中放置一个容器来显示图片。我们可以使用QMainWindow
类来创建主窗口,并使用QWidget
作为图片展示的容器。以下是一个简单的示例代码:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget, QVBoxLayout
class ImageWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle('Image Grid Viewer')
self.setGeometry(100, 100, 800, 600)
self.central_widget = QWidget()
self.setCentralWidget(self.central_widget)
self.layout = QVBoxLayout()
self.central_widget.setLayout(self.layout)
if __name__ == '__main__':
app = QApplication(sys.argv)
window = ImageWindow()
window.show()
sys.exit(app.exec_())
二、使用QGridLayout布局管理器
为了将多张图片平铺,我们可以使用QGridLayout
布局管理器,它允许我们按照网格形式排列控件。我们可以在布局管理器中添加QLabel
控件,并在每个标签中显示一张图片。
from PyQt5.QtWidgets import QLabel, QGridLayout
from PyQt5.QtGui import QPixmap
class ImageWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle('Image Grid Viewer')
self.setGeometry(100, 100, 800, 600)
self.central_widget = QWidget()
self.setCentralWidget(self.central_widget)
self.layout = QGridLayout()
self.central_widget.setLayout(self.layout)
self.load_images()
def load_images(self):
image_paths = ['image1.jpg', 'image2.jpg', 'image3.jpg', 'image4.jpg'] # 替换为实际的图片路径
rows = 2
cols = 2
for i, image_path in enumerate(image_paths):
row = i // cols
col = i % cols
label = QLabel()
pixmap = QPixmap(image_path)
label.setPixmap(pixmap)
self.layout.addWidget(label, row, col)
三、优化图片加载和显示
为了提高应用的性能和用户体验,我们需要优化图片的加载和显示。以下是一些常见的优化技巧:
-
缩放图片:在加载图片时,我们可以先缩放图片以适应标签的大小,这样可以减少内存使用并提高显示速度。
-
异步加载:如果图片数量较多,可以考虑使用多线程或异步加载图片,以避免阻塞主线程。
-
懒加载:仅在图片即将显示时才加载图片,这样可以减少内存使用并提高初始加载速度。
-
使用缓存:对于经常访问的图片,可以使用缓存机制,以减少重复加载的开销。
以下是一个示例代码,展示了如何应用这些优化技巧:
from PyQt5.QtCore import Qt, QThread, pyqtSignal
from PyQt5.QtWidgets import QScrollArea
class ImageLoaderThread(QThread):
image_loaded = pyqtSignal(int, QPixmap)
def __init__(self, image_paths):
super().__init__()
self.image_paths = image_paths
def run(self):
for i, image_path in enumerate(self.image_paths):
pixmap = QPixmap(image_path).scaled(100, 100, Qt.KeepAspectRatio)
self.image_loaded.emit(i, pixmap)
class ImageWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle('Image Grid Viewer')
self.setGeometry(100, 100, 800, 600)
self.central_widget = QWidget()
self.setCentralWidget(self.central_widget)
self.scroll_area = QScrollArea()
self.scroll_area.setWidgetResizable(True)
self.layout = QGridLayout()
self.container = QWidget()
self.container.setLayout(self.layout)
self.scroll_area.setWidget(self.container)
self.central_layout = QVBoxLayout()
self.central_layout.addWidget(self.scroll_area)
self.central_widget.setLayout(self.central_layout)
self.image_paths = ['image1.jpg', 'image2.jpg', 'image3.jpg', 'image4.jpg'] # 替换为实际的图片路径
self.labels = []
self.init_ui()
self.load_images()
def init_ui(self):
rows = 2
cols = 2
for i in range(len(self.image_paths)):
label = QLabel()
label.setFixedSize(100, 100)
self.layout.addWidget(label, i // cols, i % cols)
self.labels.append(label)
def load_images(self):
self.loader_thread = ImageLoaderThread(self.image_paths)
self.loader_thread.image_loaded.connect(self.update_image)
self.loader_thread.start()
def update_image(self, index, pixmap):
self.labels[index].setPixmap(pixmap)
四、总结
将多张图片平铺展示在Python Qt应用中涉及创建一个包含图片的窗口、使用QGridLayout布局管理器、以及优化图片加载和显示。通过合理的布局管理和优化技巧,可以提高应用的性能和用户体验。
- 创建一个包含图片的窗口:使用
QMainWindow
和QWidget
创建主窗口和图片展示容器。 - 使用QGridLayout布局管理器:通过
QGridLayout
布局管理器,将图片按照网格形式排列。 - 优化图片加载和显示:通过缩放图片、异步加载、懒加载和缓存等技巧,提高应用性能和用户体验。
希望本文的内容能帮助你在Python Qt应用中实现多张图片的平铺展示。如果你有其他问题或需要进一步的帮助,请随时提出。
相关问答FAQs:
如何在Python Qt中实现图片的平铺显示?
在Python Qt中,可以使用QGridLayout或QVBoxLayout等布局管理器来实现图片的平铺显示。首先,加载每张图片,并将其转换为QPixmap对象。接着,将QLabel与每个QPixmap关联,并将这些QLabel添加到布局中,最后将布局设置到窗口或widget中。
有哪些库可以帮助我在Python Qt中处理图片?
在Python Qt中,PyQt和PySide是最常用的库,它们都提供了丰富的功能来处理图像。可以使用PIL(Pillow)库进行图像处理,如调整大小、格式转换等,然后再将处理后的图像加载到Qt界面中。
如何优化图片加载速度以提高用户体验?
为了提升用户体验,可以采用懒加载(Lazy Loading)技术,仅在用户需要查看某个图片时才进行加载。此外,考虑使用较小的图片文件或在加载时调整图片的分辨率,这样可以减少内存占用和加载时间。此外,使用QPixmap而不是QImage可以提高显示性能,因为QPixmap是专为显示优化的。
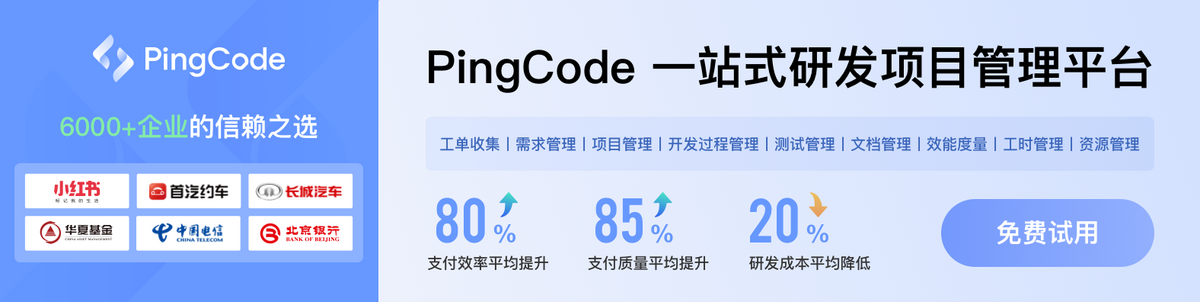