Python3 如何建立新文件:使用 open() 函数、指定模式、处理文件路径、处理异常
在Python3中,建立新文件的最简单方法是使用 open()
函数并指定模式。使用 open() 函数、指定模式、处理文件路径、处理异常 是创建新文件的关键步骤。我们将详细介绍如何实现这一过程,并确保代码在各种场景下的健壮性。
一、使用 open() 函数
open()
函数是Python内置的函数,用于打开文件。它可以创建新文件或打开现有文件进行读写。最常用的参数包括文件名和模式。
file = open('example.txt', 'w')
file.close()
在这个示例中,'w'
模式表示写入模式。如果文件不存在,Python会自动创建它。
二、指定模式
模式参数决定了文件的读写方式。常见的模式有:
'r'
:只读模式'w'
:写入模式(如果文件存在,会覆盖文件)'a'
:追加模式(如果文件存在,写入内容会附加到文件末尾)'x'
:创建模式(如果文件存在,会引发异常)'b'
:二进制模式't'
:文本模式(默认)
with open('example.txt', 'w') as file:
file.write("Hello, World!")
使用 with
语句可以确保文件在使用完后自动关闭,这是一种更好的实践。
三、处理文件路径
在实际应用中,文件路径可能不仅仅是当前目录下的文件,而是需要处理不同的路径。可以使用 os
模块来处理路径。
import os
path = os.path.join('folder', 'example.txt')
with open(path, 'w') as file:
file.write("Hello, World!")
四、处理异常
在文件操作过程中,可能会遇到各种异常,例如文件不存在、没有权限等。因此,使用 try...except
结构来捕获和处理异常是很重要的。
try:
with open('example.txt', 'w') as file:
file.write("Hello, World!")
except IOError as e:
print(f"An error occurred: {e}")
五、不同模式下的文件创建与操作
1、写入模式 ('w')
写入模式是最常用的文件创建方式。如果文件不存在,'w'
模式会创建文件;如果文件存在,会覆盖文件内容。
with open('write_mode.txt', 'w') as file:
file.write("This is written in write mode.")
2、追加模式 ('a')
追加模式用于在文件末尾添加内容。如果文件不存在,'a'
模式也会创建文件。
with open('append_mode.txt', 'a') as file:
file.write("\nThis is appended text.")
3、创建模式 ('x')
创建模式用于确保文件不存在时才创建文件。如果文件存在,会引发 FileExistsError
。
try:
with open('create_mode.txt', 'x') as file:
file.write("This file is created using 'x' mode.")
except FileExistsError:
print("File already exists.")
六、文件路径的处理与优化
在处理文件路径时,可以使用相对路径或绝对路径。为了确保代码的可移植性,最好使用相对路径和 os.path
模块。
1、相对路径
相对路径是相对于当前工作目录的路径。使用 os.path.join
可以构建跨平台的路径。
import os
relative_path = os.path.join('folder', 'example.txt')
with open(relative_path, 'w') as file:
file.write("This is a file with a relative path.")
2、绝对路径
绝对路径是从根目录开始的完整路径。使用 os.path.abspath
可以获取绝对路径。
import os
absolute_path = os.path.abspath('example.txt')
with open(absolute_path, 'w') as file:
file.write("This is a file with an absolute path.")
七、异常处理的最佳实践
在处理文件操作时,可能会遇到各种异常,如文件不存在、权限不足等。使用 try...except
结构可以捕获和处理这些异常,确保代码的健壮性。
try:
with open('example.txt', 'w') as file:
file.write("Hello, World!")
except IOError as e:
print(f"An error occurred: {e}")
1、捕获特定异常
可以捕获特定的异常类型,如 FileNotFoundError
和 PermissionError
,以便进行更具体的错误处理。
try:
with open('example.txt', 'w') as file:
file.write("Hello, World!")
except FileNotFoundError:
print("File not found.")
except PermissionError:
print("Permission denied.")
except IOError as e:
print(f"An error occurred: {e}")
八、文件操作的高级技巧
1、读写二进制文件
除了文本文件,Python还可以读写二进制文件。使用 'b'
模式即可。
with open('binary_file.bin', 'wb') as file:
file.write(b'\x00\x01\x02\x03')
2、使用 pathlib 模块
pathlib
是Python 3.4引入的模块,用于面向对象地处理文件系统路径。
from pathlib import Path
path = Path('example.txt')
path.write_text("Hello, World!")
3、临时文件
有时需要创建临时文件,tempfile
模块可以帮助处理这些情况。
import tempfile
with tempfile.NamedTemporaryFile(delete=False) as temp_file:
temp_file.write(b'Temporary file content.')
temp_file_name = temp_file.name
print(f"Temporary file created at: {temp_file_name}")
九、跨平台文件操作
为了确保代码在不同操作系统上都能正常运行,使用 os.path
和 pathlib
模块处理路径是最佳实践。
1、使用 os.path
os.path
提供了许多有用的函数来处理文件路径,如 os.path.join
、os.path.exists
和 os.path.isfile
。
import os
path = os.path.join('folder', 'example.txt')
if not os.path.exists(path):
with open(path, 'w') as file:
file.write("This is a cross-platform file path.")
2、使用 pathlib
pathlib
提供了更简洁的语法来处理文件路径。
from pathlib import Path
path = Path('folder') / 'example.txt'
if not path.exists():
path.write_text("This is a cross-platform file path using pathlib.")
十、总结
在Python3中,创建新文件是一个常见且基本的操作。通过使用 open()
函数、指定适当的模式、处理文件路径和异常,可以确保文件操作的正确性和健壮性。此外,掌握高级技巧和跨平台操作可以进一步提升代码的灵活性和可移植性。无论是在简单的脚本还是复杂的项目中,这些技巧都是不可或缺的。
相关问答FAQs:
如何在Python 3中创建新文件?
在Python 3中,可以使用内置的open()
函数来创建新文件。通过指定文件名和模式为'w'
(写入模式),如果文件不存在则会创建一个新文件。例如:
with open('new_file.txt', 'w') as file:
file.write('Hello, World!')
这段代码会创建一个名为new_file.txt
的新文件,并在其中写入“Hello, World!”。
创建文件时如何避免覆盖已有文件?
为了避免覆盖现有文件,可以使用'x'
模式。该模式会在文件已存在时引发FileExistsError
。示例代码如下:
try:
with open('existing_file.txt', 'x') as file:
file.write('This will not overwrite existing file.')
except FileExistsError:
print("文件已存在,无法创建新文件。")
通过这种方式,可以确保文件的安全性。
如何在特定目录中创建新文件?
要在特定目录中创建文件,只需在文件名之前添加路径。例如,如果希望在/home/user/documents/
目录下创建文件,可以这样写:
with open('/home/user/documents/new_file.txt', 'w') as file:
file.write('这是在特定目录下创建的文件。')
确保指定的目录存在,否则会引发FileNotFoundError
。
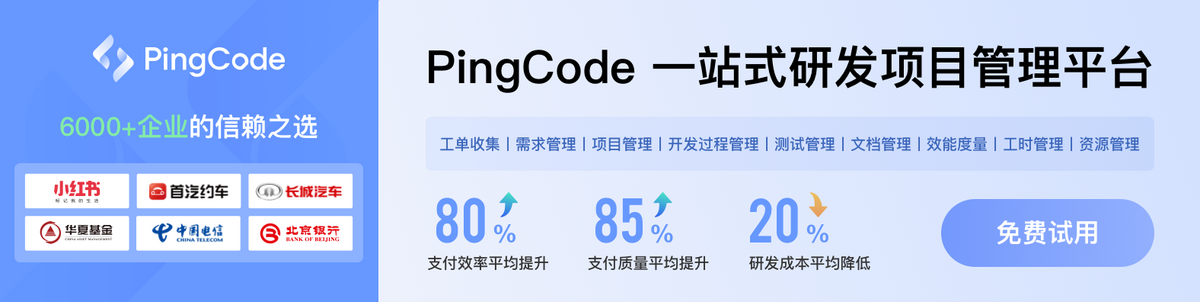