如何用Python做一个地图导航
实现Python地图导航的步骤包括:使用地图API、数据处理、路径规划、用户界面开发。在这几个步骤中,使用地图API是关键,因为它可以提供地图数据和导航功能。下面将详细介绍如何使用Python做一个地图导航系统。
一、使用地图API
使用地图API是实现地图导航的第一步。API可以提供地图数据、地理编码、路径规划等功能。常见的地图API包括谷歌地图API、Mapbox API、OpenStreetMap等。
使用谷歌地图API
谷歌地图API是一个强大的工具,可以为开发者提供丰富的地图服务。首先,你需要申请一个API密钥,这可以通过谷歌云平台完成。申请API密钥后,你可以使用谷歌地图API进行地理编码、逆地理编码和路径规划。
import googlemaps
初始化谷歌地图API
gmaps = googlemaps.Client(key='你的API密钥')
地理编码
geocode_result = gmaps.geocode('1600 Amphitheatre Parkway, Mountain View, CA')
逆地理编码
reverse_geocode_result = gmaps.reverse_geocode((37.4219999,-122.0840575))
路径规划
directions_result = gmaps.directions("Sydney Town Hall",
"Parramatta, NSW",
mode="transit")
二、数据处理
在使用地图API获取数据之后,下一步是处理这些数据。数据处理的目的是将地图API提供的原始数据转换成适合展示和分析的格式。
处理地理编码和逆地理编码数据
地理编码将地址转换为地理坐标,逆地理编码将地理坐标转换为地址。处理这些数据可以帮助我们获取和展示地理信息。
def get_coordinates(address):
# 地理编码
geocode_result = gmaps.geocode(address)
if geocode_result:
location = geocode_result[0]['geometry']['location']
return location['lat'], location['lng']
else:
return None
def get_address(lat, lng):
# 逆地理编码
reverse_geocode_result = gmaps.reverse_geocode((lat, lng))
if reverse_geocode_result:
address = reverse_geocode_result[0]['formatted_address']
return address
else:
return None
三、路径规划
路径规划是地图导航的核心功能。通过路径规划,我们可以找到从起点到终点的最佳路径。谷歌地图API提供了多种路径规划模式,包括步行、驾车、骑行和公共交通。
使用谷歌地图API进行路径规划
def get_directions(origin, destination, mode='driving'):
# 路径规划
directions_result = gmaps.directions(origin,
destination,
mode=mode)
if directions_result:
steps = directions_result[0]['legs'][0]['steps']
path = []
for step in steps:
path.append({
'distance': step['distance']['text'],
'duration': step['duration']['text'],
'instructions': step['html_instructions'],
'start_location': step['start_location'],
'end_location': step['end_location']
})
return path
else:
return None
示例
origin = '1600 Amphitheatre Parkway, Mountain View, CA'
destination = '1 Infinite Loop, Cupertino, CA'
path = get_directions(origin, destination)
for step in path:
print(step['instructions'])
四、用户界面开发
为了让用户能够方便地使用地图导航功能,我们需要开发一个用户界面。用户界面可以使用网页、桌面应用或移动应用等多种形式。这里我们将介绍如何使用Python和Flask框架开发一个简单的网页用户界面。
使用Flask框架开发网页用户界面
Flask是一个轻量级的Python web框架,非常适合快速开发和部署应用。
- 安装Flask
pip install flask
- 创建Flask应用
from flask import Flask, request, render_template
import googlemaps
app = Flask(__name__)
gmaps = googlemaps.Client(key='你的API密钥')
@app.route('/')
def index():
return render_template('index.html')
@app.route('/directions', methods=['POST'])
def directions():
origin = request.form['origin']
destination = request.form['destination']
mode = request.form['mode']
path = get_directions(origin, destination, mode)
return render_template('directions.html', path=path)
def get_directions(origin, destination, mode='driving'):
directions_result = gmaps.directions(origin, destination, mode=mode)
if directions_result:
steps = directions_result[0]['legs'][0]['steps']
path = []
for step in steps:
path.append({
'distance': step['distance']['text'],
'duration': step['duration']['text'],
'instructions': step['html_instructions'],
'start_location': step['start_location'],
'end_location': step['end_location']
})
return path
else:
return None
if __name__ == '__main__':
app.run(debug=True)
- 创建HTML模板
在templates
目录下创建index.html
和directions.html
两个文件。
index.html
<!DOCTYPE html>
<html>
<head>
<title>地图导航</title>
</head>
<body>
<h1>地图导航</h1>
<form action="/directions" method="post">
<label for="origin">起点:</label>
<input type="text" id="origin" name="origin"><br>
<label for="destination">终点:</label>
<input type="text" id="destination" name="destination"><br>
<label for="mode">模式:</label>
<select id="mode" name="mode">
<option value="driving">驾车</option>
<option value="walking">步行</option>
<option value="bicycling">骑行</option>
<option value="transit">公共交通</option>
</select><br>
<input type="submit" value="获取路径">
</form>
</body>
</html>
directions.html
<!DOCTYPE html>
<html>
<head>
<title>路径结果</title>
</head>
<body>
<h1>路径结果</h1>
<ul>
{% for step in path %}
<li>{{ step.instructions }} ({{ step.distance }}, {{ step.duration }})</li>
{% endfor %}
</ul>
<a href="/">返回</a>
</body>
</html>
五、优化和扩展
除了基本的地图导航功能,我们还可以对系统进行优化和扩展,以提供更好的用户体验和更多的功能。
缓存和优化性能
为了提高系统性能,我们可以使用缓存技术缓存经常访问的数据。例如,可以使用Redis或Memcached缓存地理编码和路径规划结果,以减少API调用次数。
增加更多功能
可以增加更多功能,如实时交通信息、兴趣点搜索、路线保存和分享等。例如,可以使用谷歌地图API提供的实时交通信息来优化路径规划,避免拥堵。
移动应用开发
除了网页用户界面,还可以开发移动应用。可以使用React Native或Flutter等跨平台框架开发移动应用,并集成地图导航功能。
import googlemaps
初始化谷歌地图API
gmaps = googlemaps.Client(key='你的API密钥')
地理编码
geocode_result = gmaps.geocode('1600 Amphitheatre Parkway, Mountain View, CA')
逆地理编码
reverse_geocode_result = gmaps.reverse_geocode((37.4219999,-122.0840575))
路径规划
directions_result = gmaps.directions("Sydney Town Hall",
"Parramatta, NSW",
mode="transit")
总结
通过本文的介绍,我们详细讲解了如何使用Python做一个地图导航系统。我们从使用地图API开始,介绍了数据处理、路径规划和用户界面开发的具体步骤。最后,我们还探讨了系统优化和扩展的可能性。希望这篇文章能够帮助你理解和实现Python地图导航系统。
相关问答FAQs:
如何选择合适的地图API来实现导航功能?
在使用Python进行地图导航开发时,选择合适的地图API至关重要。常见的API包括Google Maps API、OpenStreetMap、Mapbox等。Google Maps API提供全面的服务,包括实时交通信息和路线优化,而OpenStreetMap则是一个开源选择,适合需要自定义地图样式的项目。确保根据项目需求、预算和技术支持来做出选择。
我需要掌握哪些Python库才能实现地图导航功能?
为了实现地图导航功能,可以使用一些强大的Python库,比如Folium、Geopy和Matplotlib。Folium可用于生成交互式地图,Geopy则帮助进行地理编码和地址解析,Matplotlib则可以用于数据可视化和图形呈现。此外,利用requests库与API进行交互,获取地图数据和导航信息也是必不可少的。
如何在Python中处理用户输入的导航请求?
处理用户输入的导航请求涉及到解析用户提供的起点和终点信息。可以通过简单的命令行输入或创建图形用户界面来接受用户的输入。使用正则表达式可以帮助提取地址信息,然后通过调用地图API进行地址解析和路线计算。确保对用户的输入进行必要的验证,以提高导航的准确性和用户体验。
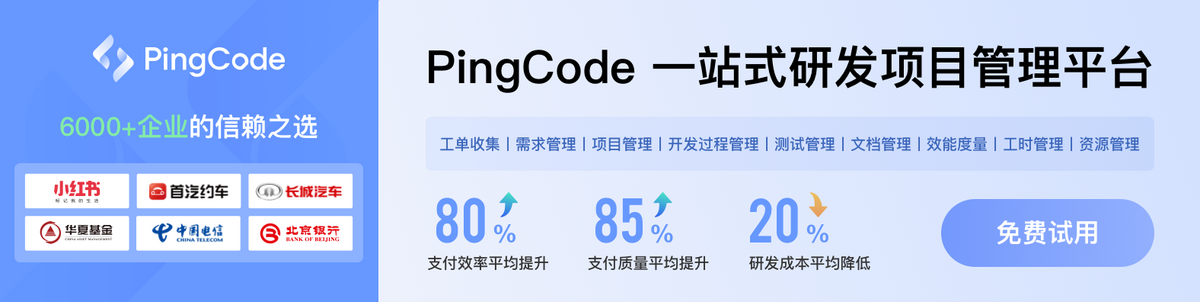