在Python中提取包含关键字的文本,可以通过多种方法实现,如正则表达式、字符串方法、自然语言处理工具包(如NLTK)等。 其中,正则表达式 是最常用且高效的方法之一,因为它提供了强大的模式匹配功能。
为了更详细地解释使用正则表达式提取包含关键字的文本,假设我们需要从一段文本中提取所有包含“Python”这个关键字的句子。我们可以利用Python的re
模块,通过匹配模式来定位这些句子。
一、正则表达式的基础
正则表达式是一种用于模式匹配的强大工具。Python 提供了内置的 re
模块来处理正则表达式。首先,我们需要了解一些基本的正则表达式符号和操作。
1、常用的正则表达式符号
.
:匹配任意单个字符(除换行符外)。*
:匹配前面的字符零次或多次。+
:匹配前面的字符一次或多次。?
:匹配前面的字符零次或一次。[]
:匹配方括号中的任意一个字符。^
:匹配字符串的开头。- `在Python中提取包含关键字的文本,可以通过多种方法实现,如正则表达式、字符串方法、自然语言处理工具包(如NLTK)等。 其中,正则表达式 是最常用且高效的方法之一,因为它提供了强大的模式匹配功能。
为了更详细地解释使用正则表达式提取包含关键字的文本,假设我们需要从一段文本中提取所有包含“Python”这个关键字的句子。我们可以利用Python的re
模块,通过匹配模式来定位这些句子。
一、正则表达式的基础
正则表达式是一种用于模式匹配的强大工具。Python 提供了内置的 re
模块来处理正则表达式。首先,我们需要了解一些基本的正则表达式符号和操作。
1、常用的正则表达式符号
.
:匹配任意单个字符(除换行符外)。*
:匹配前面的字符零次或多次。+
:匹配前面的字符一次或多次。?
:匹配前面的字符零次或一次。[]
:匹配方括号中的任意一个字符。^
:匹配字符串的开头。- :匹配字符串的结尾。
\d
:匹配一个数字字符。\w
:匹配一个字母或数字字符。\s
:匹配一个空白字符。
2、基本的正则表达式操作
re.search(pattern, string)
:在字符串中搜索模式,返回第一个匹配的对象。re.findall(pattern, string)
:返回字符串中所有非重叠匹配的列表。re.sub(pattern, repl, string)
:替换字符串中所有匹配的模式。
二、使用正则表达式提取关键字文本
在了解了基础的正则表达式操作后,我们可以使用它们来提取包含关键字的文本。假设我们有如下文本:
text = """
Python is an interpreted, high-level, general-purpose programming language.
Created by Guido van Rossum and first released in 1991, Python's design philosophy emphasizes code readability with its notable use of significant whitespace.
Its language constructs and object-oriented approach aim to help programmers write clear, logical code for small and large-scale projects.
"""
我们希望提取所有包含“Python”关键字的句子。可以使用如下代码:
import re
text = """
Python is an interpreted, high-level, general-purpose programming language.
Created by Guido van Rossum and first released in 1991, Python's design philosophy emphasizes code readability with its notable use of significant whitespace.
Its language constructs and object-oriented approach aim to help programmers write clear, logical code for small and large-scale projects.
"""
定义正则表达式模式
pattern = r'[^.]*\bPython\b[^.]*\.'
使用findall方法提取包含关键字的句子
sentences_with_keyword = re.findall(pattern, text)
for sentence in sentences_with_keyword:
print(sentence)
以上代码将输出包含关键字“Python”的句子。解释如下:
\bPython\b
:匹配单词边界中的“Python”。[^.]*
:匹配除句号外的任意字符零次或多次。\.
:匹配句号。
三、使用字符串方法提取关键字文本
除了正则表达式,Python的字符串方法也能实现相似的功能。假设我们仍然使用上面的文本,我们可以利用字符串的split
方法和列表推导来提取包含关键字的句子。
text = """
Python is an interpreted, high-level, general-purpose programming language.
Created by Guido van Rossum and first released in 1991, Python's design philosophy emphasizes code readability with its notable use of significant whitespace.
Its language constructs and object-oriented approach aim to help programmers write clear, logical code for small and large-scale projects.
"""
将文本按句号分割
sentences = text.split('.')
提取包含关键字的句子
sentences_with_keyword = [sentence.strip() + '.' for sentence in sentences if 'Python' in sentence]
for sentence in sentences_with_keyword:
print(sentence)
这种方法虽然较为直接,但对复杂的文本结构处理不如正则表达式灵活。
四、使用自然语言处理工具包(NLTK)
自然语言处理工具包(NLTK)是Python中非常流行的文本处理库。它提供了丰富的工具来处理和分析人类语言数据。我们可以利用NLTK来实现关键字文本的提取。
1、安装NLTK
首先,安装NLTK库:
pip install nltk
2、使用NLTK提取关键字文本
import nltk
from nltk.tokenize import sent_tokenize
下载punkt模型
nltk.download('punkt')
text = """
Python is an interpreted, high-level, general-purpose programming language.
Created by Guido van Rossum and first released in 1991, Python's design philosophy emphasizes code readability with its notable use of significant whitespace.
Its language constructs and object-oriented approach aim to help programmers write clear, logical code for small and large-scale projects.
"""
使用NLTK的sent_tokenize方法将文本分割为句子
sentences = sent_tokenize(text)
提取包含关键字的句子
sentences_with_keyword = [sentence for sentence in sentences if 'Python' in sentence]
for sentence in sentences_with_keyword:
print(sentence)
NLTK的sent_tokenize
方法能够准确地将文本分割为句子,即使句子结构复杂。
五、综合应用与实践
在实际应用中,我们通常需要处理更复杂的文本数据,可能包含多种格式和结构。为了提高文本处理的准确性和效率,可以结合多种方法来提取关键字文本。例如,我们可以先使用正则表达式对文本进行初步清洗,然后利用NLTK进行更精细的处理。
1、初步清洗文本
import re
text = """
Python is an interpreted, high-level, general-purpose programming language.
Created by Guido van Rossum and first released in 1991, Python's design philosophy emphasizes code readability with its notable use of significant whitespace.
Its language constructs and object-oriented approach aim to help programmers write clear, logical code for small and large-scale projects.
"""
清洗文本,移除多余的空白和特殊字符
cleaned_text = re.sub(r'\s+', ' ', text).strip()
print(cleaned_text)
2、精细处理文本
import nltk
from nltk.tokenize import sent_tokenize
下载punkt模型
nltk.download('punkt')
使用NLTK将清洗后的文本分割为句子
sentences = sent_tokenize(cleaned_text)
提取包含关键字的句子
sentences_with_keyword = [sentence for sentence in sentences if 'Python' in sentence]
for sentence in sentences_with_keyword:
print(sentence)
通过结合正则表达式和NLTK,我们可以更高效地提取包含关键字的文本,适应各种复杂的文本处理需求。
综上所述,Python中提取包含关键字的文本有多种方法,包括正则表达式、字符串方法和自然语言处理工具包(如NLTK)。根据具体需求选择合适的方法,可以大大提高文本处理的效率和准确性。
相关问答FAQs:
如何在Python中提取特定关键字的文本?
在Python中,可以使用字符串的find()
方法或正则表达式来查找和提取包含特定关键字的文本。通过遍历文本或使用re
模块,可以轻松识别并提取包含目标关键字的句子或段落。示例代码如下:
import re
text = "这是一个示例文本,其中包含关键字。"
keyword = "关键字"
# 使用正则表达式提取
matches = re.findall(r'([^.]*?{}[^.]*\.)'.format(keyword), text)
print(matches)
我可以使用哪些Python库来帮助提取关键字相关的文本?
有多个Python库可以帮助提取相关文本。re
模块用于正则表达式匹配,nltk
和spaCy
等自然语言处理库能够进行更复杂的文本分析和处理。此外,pandas
库在处理表格数据时也非常有用,可以通过条件筛选来提取特定关键字的相关行。
在提取文本时,如何提高关键字匹配的准确性?
为了提高关键字匹配的准确性,可以考虑使用大小写不敏感的匹配、去掉标点符号,或者使用词干提取和词形还原技术。利用nltk
或spaCy
库,可以在提取前对文本进行预处理,如分词、去除停用词等,从而提高关键字的匹配效果。
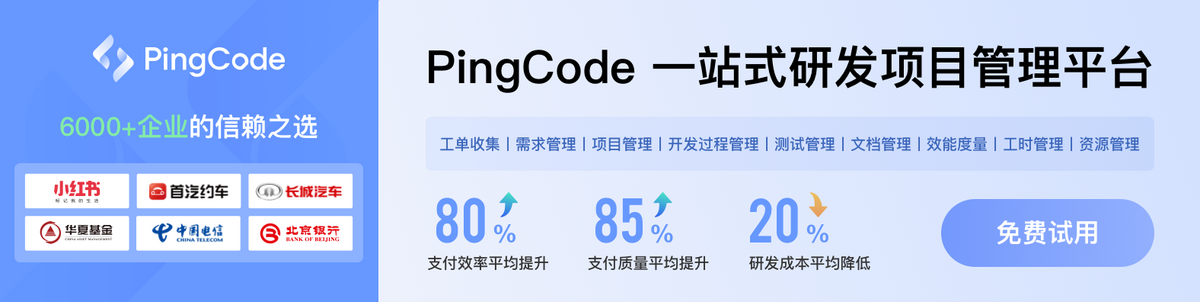