在 Python 中判断 SQL 的表是否存在可以通过多种方法来实现,主要方法有:使用系统表查询、使用信息模式(INFORMATION_SCHEMA)、执行特定的 SQL 语句。 其中,使用信息模式(INFORMATION_SCHEMA)是最为通用和标准的方法。接下来将详细介绍如何在 Python 中使用这些方法来判断 SQL 表是否存在。
一、使用系统表查询
系统表查询是根据数据库系统表(如 MySQL 的 INFORMATION_SCHEMA.TABLES
)中的信息来判断表是否存在。这种方法适用于大多数关系型数据库,包括 MySQL、PostgreSQL、SQL Server 等。
1、MySQL
在 MySQL 中,您可以查询 INFORMATION_SCHEMA.TABLES
来检查表是否存在。以下是一个示例代码:
import pymysql
def check_table_exists(connection, table_name):
with connection.cursor() as cursor:
cursor.execute("""
SELECT COUNT(*)
FROM information_schema.tables
WHERE table_schema = DATABASE()
AND table_name = %s
""", (table_name,))
return cursor.fetchone()[0] > 0
connection = pymysql.connect(
host='localhost',
user='your_username',
password='your_password',
database='your_database'
)
table_exists = check_table_exists(connection, 'your_table')
print("Table exists:", table_exists)
2、PostgreSQL
在 PostgreSQL 中,您可以查询 pg_catalog.pg_tables
来检查表是否存在。以下是一个示例代码:
import psycopg2
def check_table_exists(connection, table_name):
with connection.cursor() as cursor:
cursor.execute("""
SELECT EXISTS (
SELECT 1
FROM pg_catalog.pg_tables
WHERE tablename = %s
)
""", (table_name,))
return cursor.fetchone()[0]
connection = psycopg2.connect(
host='localhost',
user='your_username',
password='your_password',
dbname='your_database'
)
table_exists = check_table_exists(connection, 'your_table')
print("Table exists:", table_exists)
二、使用信息模式(INFORMATION_SCHEMA)
INFORMATION_SCHEMA
是一个包含了所有数据库对象信息的模式。您可以在其中查询表、列、索引等信息来判断表是否存在。
1、使用 INFORMATION_SCHEMA.TABLES
import mysql.connector
def check_table_exists(connection, table_name):
cursor = connection.cursor()
cursor.execute("""
SELECT COUNT(*)
FROM INFORMATION_SCHEMA.TABLES
WHERE TABLE_SCHEMA = %s
AND TABLE_NAME = %s
""", (connection.database, table_name))
return cursor.fetchone()[0] > 0
connection = mysql.connector.connect(
host='localhost',
user='your_username',
password='your_password',
database='your_database'
)
table_exists = check_table_exists(connection, 'your_table')
print("Table exists:", table_exists)
三、执行特定的 SQL 语句
您还可以使用 SHOW TABLES
或 SELECT
语句来判断表是否存在。这种方法虽然不如前两种方法标准,但也非常有效。
1、MySQL 中的 SHOW TABLES
import pymysql
def check_table_exists(connection, table_name):
with connection.cursor() as cursor:
cursor.execute("SHOW TABLES LIKE %s", (table_name,))
return cursor.fetchone() is not None
connection = pymysql.connect(
host='localhost',
user='your_username',
password='your_password',
database='your_database'
)
table_exists = check_table_exists(connection, 'your_table')
print("Table exists:", table_exists)
2、PostgreSQL 中的 SELECT
import psycopg2
def check_table_exists(connection, table_name):
with connection.cursor() as cursor:
cursor.execute("""
SELECT to_regclass(%s)
""", (table_name,))
return cursor.fetchone()[0] is not None
connection = psycopg2.connect(
host='localhost',
user='your_username',
password='your_password',
dbname='your_database'
)
table_exists = check_table_exists(connection, 'your_table')
print("Table exists:", table_exists)
四、结合 ORM 框架
如果您使用 ORM 框架(如 SQLAlchemy),可以利用 ORM 提供的方法来判断表是否存在。
1、使用 SQLAlchemy
from sqlalchemy import create_engine, inspect
engine = create_engine('mysql+pymysql://your_username:your_password@localhost/your_database')
inspector = inspect(engine)
def check_table_exists(table_name):
return inspector.has_table(table_name)
table_exists = check_table_exists('your_table')
print("Table exists:", table_exists)
结论
在 Python 中判断 SQL 表是否存在,可以通过查询系统表、使用 INFORMATION_SCHEMA
或执行特定 SQL 语句来实现。此外,利用 ORM 框架提供的方法也是一种简便的方法。无论采用哪种方法,都需要根据具体的数据库类型和应用场景来选择最合适的解决方案。通过这些方法,您可以确保数据库操作的安全性和可靠性,从而避免因表不存在而引发的错误。
相关问答FAQs:
如何使用Python连接数据库并判断SQL表是否存在?
要判断SQL表是否存在,首先需要使用Python中的数据库连接库,例如sqlite3
、mysql-connector
或pyodbc
等。连接到数据库后,可以执行相应的SQL查询语句来检查表的存在性。例如,在SQLite中可以使用SELECT name FROM sqlite_master WHERE type='table' AND name='your_table_name';
来查询。
在Python中检查表存在性时,有哪些常见的错误处理方式?
在执行表存在性检查时,可能会遇到数据库连接失败或SQL语法错误等问题。使用try-except
语句来捕捉这些异常是一个良好的做法。通过捕获特定的异常类型,可以为用户提供更明确的错误信息,并采取相应的补救措施。
判断表是否存在后,可以进行哪些操作?
一旦确认表存在,可以进行多种操作,例如查询数据、插入新记录或更新现有记录。如果表不存在,根据业务需求,可以选择创建该表或返回相应的提示信息。使用Python的ORM框架如SQLAlchemy,可以简化这些操作,提供更高效的代码管理。
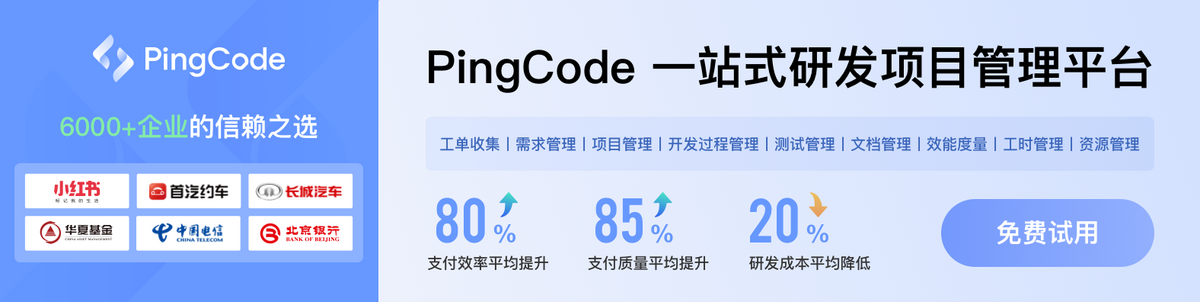