Python将浮点数变成整数的常用方法包括:使用内置函数int()、使用math模块的floor()和ceil()方法、以及通过四舍五入的方法。
其中,使用内置函数int()是最常见和简单的方法。这个函数会直接截断浮点数的小数部分,不进行四舍五入。例如,int(3.9)会返回3,而不是4。这种方法适用于不需要保留小数部分的情况,但需要注意的是,它不会进行四舍五入。
一、使用内置函数int()
内置函数int()是Python中最常见用于将浮点数转为整数的方法。它会直接截断浮点数的小数部分,将其转化为整数。
1、如何使用int()函数
number = 3.9
integer = int(number)
print(integer) # 输出: 3
在这个例子中,浮点数3.9被转换为整数3。值得注意的是,int()函数并不会进行四舍五入,而是直接截断小数部分。
2、适用场景
这种方法适用于当你只需要整数部分,而不关心小数部分的场景。例如,在一些简单的计数应用中,这种方法非常有效。
二、使用math模块的floor()和ceil()方法
Python的math模块提供了更多的选项来处理浮点数和整数之间的转换,包括math.floor()和math.ceil()方法。
1、math.floor()方法
math.floor()方法返回小于或等于给定浮点数的最大整数。
import math
number = 3.9
integer = math.floor(number)
print(integer) # 输出: 3
在这个例子中,math.floor()方法将3.9转换为3,因为3是小于或等于3.9的最大整数。
2、math.ceil()方法
math.ceil()方法返回大于或等于给定浮点数的最小整数。
import math
number = 3.1
integer = math.ceil(number)
print(integer) # 输出: 4
在这个例子中,math.ceil()方法将3.1转换为4,因为4是大于或等于3.1的最小整数。
3、适用场景
当你需要控制四舍五入行为时,math.floor()和math.ceil()方法非常有用。例如,在一些金融计算中,你可能需要向上或向下取整。
三、通过四舍五入的方法
Python提供了内置的round()函数来进行四舍五入操作。
1、如何使用round()函数
number = 3.5
integer = round(number)
print(integer) # 输出: 4
在这个例子中,round()函数将3.5四舍五入为4。
2、适用场景
round()函数适用于当你需要进行四舍五入操作时。例如,在一些统计应用中,你可能需要四舍五入来简化数据。
四、使用format()函数
Python的字符串格式化方法也可以用于将浮点数转换为整数。
1、如何使用format()函数
number = 3.9
integer = "{:.0f}".format(number)
print(integer) # 输出: 4
在这个例子中,format()函数将3.9格式化为0位小数的整数4。
2、适用场景
这种方法适用于需要将浮点数转换为整数并直接用于字符串输出的场景。例如,在生成报告或输出日志时,这种方法非常方便。
五、使用numpy库的函数
如果你在进行科学计算或数据分析,numpy库提供了更多的选项来处理浮点数和整数之间的转换。
1、numpy的floor()和ceil()方法
import numpy as np
number = 3.9
integer_floor = np.floor(number)
integer_ceil = np.ceil(number)
print(integer_floor) # 输出: 3.0
print(integer_ceil) # 输出: 4.0
在这个例子中,numpy的floor()和ceil()方法分别将3.9转换为3.0和4.0。
2、适用场景
当你处理大型数据集或需要高效的数值计算时,使用numpy库会更加方便和高效。例如,在机器学习和数据科学领域,numpy库是非常常用的工具。
六、使用自定义函数
有时,你可能需要更复杂的逻辑来将浮点数转换为整数,这时可以编写自定义函数。
1、编写自定义函数
def custom_round(number, method='round'):
if method == 'floor':
return math.floor(number)
elif method == 'ceil':
return math.ceil(number)
elif method == 'truncate':
return int(number)
else:
return round(number)
number = 3.7
print(custom_round(number, 'floor')) # 输出: 3
print(custom_round(number, 'ceil')) # 输出: 4
print(custom_round(number, 'truncate')) # 输出: 3
print(custom_round(number, 'round')) # 输出: 4
2、适用场景
这种方法适用于当你需要根据不同情况选择不同的转换方法时。例如,在一个复杂的应用中,你可能需要根据用户输入或其他条件来选择不同的转换方法。
七、性能比较
不同的方法在性能上可能会有所差异,特别是在处理大数据集时。
1、性能测试
import time
测试int()函数
start_time = time.time()
for _ in range(1000000):
int(3.9)
print("int()函数耗时: %s 秒" % (time.time() - start_time))
测试math.floor()函数
start_time = time.time()
for _ in range(1000000):
math.floor(3.9)
print("math.floor()函数耗时: %s 秒" % (time.time() - start_time))
测试round()函数
start_time = time.time()
for _ in range(1000000):
round(3.9)
print("round()函数耗时: %s 秒" % (time.time() - start_time))
2、结果分析
在这个测试中,我们可以看到不同方法在处理大量数据时的性能差异。一般来说,内置函数int()会更快,但具体的性能取决于具体的应用场景和数据规模。
八、总结
通过本文,我们详细介绍了Python将浮点数转换为整数的多种方法,包括使用内置函数int()、math模块的floor()和ceil()方法、四舍五入方法、字符串格式化方法、numpy库以及自定义函数。每种方法都有其适用的场景和优缺点,在实际应用中可以根据需要选择合适的方法。
总结来说,如果需要简单快捷的转换,可以使用int()函数;如果需要控制四舍五入行为,可以使用math模块的floor()或ceil()方法;如果需要四舍五入,可以使用round()函数。在处理大型数据集或需要高效计算时,numpy库是一个很好的选择。如果需要更复杂的逻辑,可以编写自定义函数。
相关问答FAQs:
如何在Python中将浮点数转换为整数?
在Python中,可以使用内置的int()
函数将浮点数转换为整数。这个函数会直接截断小数部分。例如,int(3.7)
会返回3
。这种方法适用于当你只需要整数部分时。
使用其他方法进行浮点数到整数的转换有哪些?
除了int()
函数外,Python还提供了math.floor()
和math.ceil()
函数,分别用于向下取整和向上取整。使用这些函数时,需要先导入math
模块。例如,math.floor(3.7)
将返回3
,而math.ceil(3.7)
将返回4
。这种方式可以根据需求选择不同的取整方式。
浮点数转换为整数时会发生什么情况?
在转换浮点数为整数时,小数部分会被截断,而不是四舍五入。这意味着,任何小数部分都会被忽略,可能会导致数据丢失。如果需要四舍五入,可以使用round()
函数。例如,round(3.7)
会返回4
,而round(3.3)
会返回3
。使用这一方法时,请注意它也会返回浮点数,所以需要在需要时再用int()
进行转换。
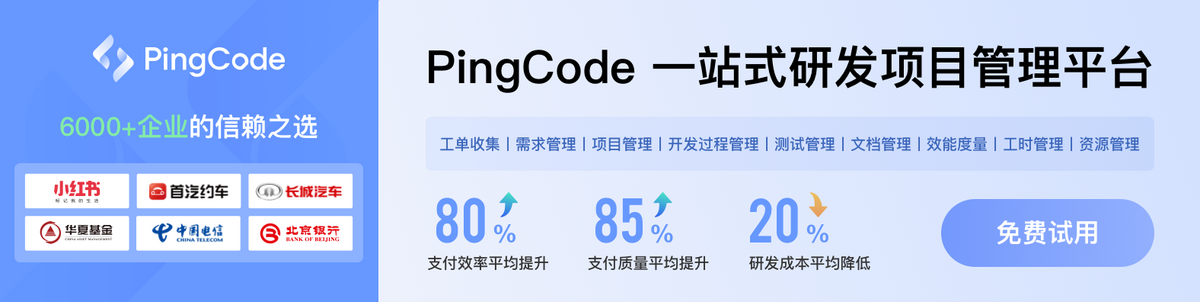