Python编写一个定时死机程序的方法主要包括:使用线程、定时器、操作系统命令。 其中,使用Python的threading
模块可以设置定时器,结合操作系统命令实现电脑死机效果。这里我们将详细介绍如何使用Python编写一个定时死机程序。
一、使用threading
模块设置定时器
Python的threading
模块提供了一个简便的方法来创建定时器。定时器是一个线程,在经过指定的时间间隔后执行某个函数。可以使用它来在特定时间后执行死机命令。
1、导入必要的模块
在编写定时死机程序之前,需要导入一些必要的模块。主要模块包括threading
、os
和time
模块。threading
模块用于创建定时器,os
模块用于执行操作系统命令,time
模块用于处理时间相关操作。
import threading
import os
import time
2、定义死机函数
在设置定时器之前,需要定义一个死机函数。这个函数将包含执行死机操作的代码。在Windows系统上,可以使用os.system
函数执行shutdown
命令来实现死机效果。
def crash_computer():
os.system("shutdown /s /f /t 0")
在这个函数中,shutdown /s /f /t 0
命令将立即关闭计算机。/s
选项表示关闭计算机,/f
选项表示强制关闭,/t 0
表示立即关闭。
3、创建定时器
在定义了死机函数之后,可以使用threading.Timer
类创建一个定时器。定时器将在指定的时间间隔后执行死机函数。
def set_timer(seconds):
timer = threading.Timer(seconds, crash_computer)
timer.start()
在这个函数中,threading.Timer
类创建一个定时器,seconds
参数表示定时器的时间间隔,单位为秒。定时器将在经过指定的时间间隔后执行crash_computer
函数。
二、主程序
在定义了定时器之后,需要编写主程序来接受用户输入的时间间隔,并设置定时器。在主程序中,可以使用input
函数接受用户输入的时间间隔,并调用set_timer
函数设置定时器。
if __name__ == "__main__":
try:
seconds = int(input("Enter the time (in seconds) after which the computer will crash: "))
set_timer(seconds)
print(f"The computer will crash in {seconds} seconds.")
except ValueError:
print("Invalid input. Please enter a valid number.")
在这个主程序中,input
函数接受用户输入的时间间隔,并将其转换为整数。然后调用set_timer
函数设置定时器。如果用户输入的不是有效的数字,程序将打印一条错误消息。
三、跨平台实现
上面的方法主要适用于Windows系统,如果需要在其他操作系统(如Linux或MacOS)上实现类似的功能,可以使用不同的命令。
1、Linux系统
在Linux系统上,可以使用os.system
函数执行shutdown
命令来实现死机效果。
def crash_computer():
os.system("shutdown now")
2、MacOS系统
在MacOS系统上,可以使用os.system
函数执行sudo shutdown -h now
命令来实现死机效果。
def crash_computer():
os.system("sudo shutdown -h now")
四、注意事项
在编写定时死机程序时,需要注意以下几点:
1、安全性
编写和执行这样的程序可能会带来一定的风险,特别是在生产环境或重要数据存在的计算机上。因此,建议在测试环境或虚拟机中运行该程序,并确保已经备份重要数据。
2、权限
执行关闭计算机的命令通常需要管理员权限。在Windows系统上,可能需要以管理员身份运行Python脚本;在Linux和MacOS系统上,可能需要使用sudo
命令并输入管理员密码。
3、用户体验
定时死机程序可能会对用户体验造成负面影响。因此,在实际应用中,应避免使用这样的程序,除非有明确的需求和充分的理由。
五、扩展功能
除了定时死机功能,还可以扩展程序的功能。例如,添加一个取消定时器的功能,或者在死机前显示一个警告消息。
1、取消定时器
可以通过保存定时器对象并调用其cancel
方法来取消定时器。
def set_timer(seconds):
timer = threading.Timer(seconds, crash_computer)
timer.start()
return timer
if __name__ == "__main__":
try:
seconds = int(input("Enter the time (in seconds) after which the computer will crash: "))
timer = set_timer(seconds)
print(f"The computer will crash in {seconds} seconds.")
cancel = input("Do you want to cancel the timer? (yes/no): ")
if cancel.lower() == 'yes':
timer.cancel()
print("The timer has been cancelled.")
except ValueError:
print("Invalid input. Please enter a valid number.")
2、显示警告消息
可以在死机前显示一个警告消息,提示用户计算机即将关闭。
def crash_computer():
os.system("shutdown /s /f /t 60")
print("The computer will shut down in 60 seconds.")
def set_timer(seconds):
timer = threading.Timer(seconds, crash_computer)
timer.start()
return timer
if __name__ == "__main__":
try:
seconds = int(input("Enter the time (in seconds) after which the computer will crash: "))
timer = set_timer(seconds)
print(f"The computer will crash in {seconds} seconds.")
cancel = input("Do you want to cancel the timer? (yes/no): ")
if cancel.lower() == 'yes':
timer.cancel()
print("The timer has been cancelled.")
except ValueError:
print("Invalid input. Please enter a valid number.")
在这个扩展功能中,crash_computer
函数将在执行死机命令之前,先显示一个警告消息,并将关闭计算机的时间延迟60秒。这样,用户将有足够的时间保存工作并关闭程序。
六、总结
通过使用Python的threading
模块和操作系统命令,可以编写一个定时死机程序。本文详细介绍了如何使用threading.Timer
类设置定时器,并结合操作系统命令实现电脑死机效果。同时,还介绍了跨平台实现方法和一些注意事项。在实际应用中,应谨慎使用定时死机程序,并确保已经备份重要数据。
相关问答FAQs:
如何使用Python实现定时死机的功能?
可以通过使用Python的os
模块或subprocess
模块来执行系统命令以实现定时死机。你可以使用time.sleep()
函数来设置延迟,然后调用相应的命令。对于Windows系统,可以使用shutdown /s /t
命令;对于Linux系统,可以使用shutdown now
命令。确保在执行之前做好数据备份,以免丢失重要信息。
在编写定时死机程序时需要注意哪些安全性问题?
确保在使用该程序时,您有权进行系统关机操作。避免在生产环境或公共计算机上执行此类代码,以防止意外断电或数据丢失。建议在运行代码之前充分测试,并考虑添加用户确认的步骤,以避免误操作。
是否可以通过Python设置定时重启而不是死机?
是的,Python可以实现定时重启。与定时死机类似,你可以使用os
或subprocess
模块,调用系统重启命令。对于Windows,可以使用shutdown /r /t
命令,而在Linux上可以使用reboot
命令。确保设置合适的时间间隔,并在重启前保存所有未保存的工作。
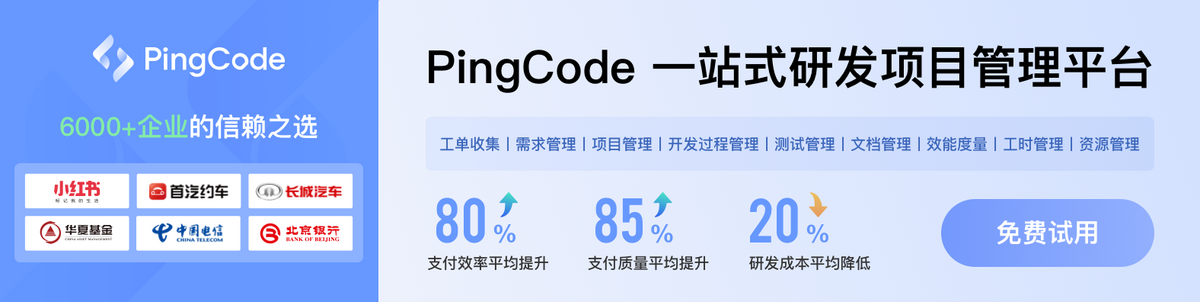