判断两个图片是否相同的Python方法包括:哈希比较、像素比较、直方图比较、特征点匹配。其中,哈希比较是一种常见且高效的方式,它通过将图像转换为哈希值来进行比较。接下来我们将详细介绍这些方法,并提供示例代码来说明如何使用这些方法进行图片相似度判断。
一、哈希比较
哈希比较是一种常用的图片相似度判断方法,通过将图片转换成哈希值,然后比较这两个哈希值的相似度来判断图片是否相同。常见的哈希算法包括感知哈希(Perceptual Hash)、差异哈希(Difference Hash)、平均哈希(Average Hash)等。
1、感知哈希(Perceptual Hash)
感知哈希通过对图像内容进行分析,提取其特征,生成一个固定长度的二进制字符串。即使图像有些许改变,感知哈希生成的字符串也会保持相似。
from PIL import Image
import imagehash
def perceptual_hash(image_path):
img = Image.open(image_path)
hash = imagehash.phash(img)
return hash
def are_images_similar(image_path1, image_path2, threshold=5):
hash1 = perceptual_hash(image_path1)
hash2 = perceptual_hash(image_path2)
return hash1 - hash2 < threshold
image1 = 'image1.jpg'
image2 = 'image2.jpg'
print(are_images_similar(image1, image2))
2、差异哈希(Difference Hash)
差异哈希通过比较相邻像素的灰度值差异来生成哈希值,具有较高的抗噪能力。
def difference_hash(image_path):
img = Image.open(image_path)
hash = imagehash.dhash(img)
return hash
def are_images_similar(image_path1, image_path2, threshold=5):
hash1 = difference_hash(image_path1)
hash2 = difference_hash(image_path2)
return hash1 - hash2 < threshold
print(are_images_similar(image1, image2))
3、平均哈希(Average Hash)
平均哈希通过将图像缩小并转换为灰度图,然后计算平均灰度值,并根据灰度值生成哈希值。
def average_hash(image_path):
img = Image.open(image_path)
hash = imagehash.average_hash(img)
return hash
def are_images_similar(image_path1, image_path2, threshold=5):
hash1 = average_hash(image_path1)
hash2 = average_hash(image_path2)
return hash1 - hash2 < threshold
print(are_images_similar(image1, image2))
二、像素比较
像素比较是最直接的图片相似度判断方法,通过逐像素比较两张图片的像素值来判断它们是否相同。这种方法适用于完全相同的图片比较。
1、逐像素比较
from PIL import Image
import numpy as np
def pixel_compare(image_path1, image_path2):
img1 = Image.open(image_path1)
img2 = Image.open(image_path2)
if img1.size != img2.size:
return False
pixels1 = np.array(img1)
pixels2 = np.array(img2)
return np.array_equal(pixels1, pixels2)
print(pixel_compare(image1, image2))
2、计算均方误差(MSE)
均方误差是另一种常用的像素比较方法,通过计算两张图片对应像素值的均方误差来判断相似度。
def mse(image_path1, image_path2):
img1 = Image.open(image_path1).convert('L')
img2 = Image.open(image_path2).convert('L')
pixels1 = np.array(img1)
pixels2 = np.array(img2)
err = np.sum((pixels1 - pixels2) 2)
err /= float(pixels1.shape[0] * pixels1.shape[1])
return err
def are_images_similar(image_path1, image_path2, threshold=1000):
error = mse(image_path1, image_path2)
return error < threshold
print(are_images_similar(image1, image2))
三、直方图比较
直方图比较通过比较两张图片的颜色直方图来判断相似度。这种方法适用于色彩分布相似的图片比较。
1、直方图相似度计算
def histogram_compare(image_path1, image_path2):
img1 = Image.open(image_path1).convert('RGB')
img2 = Image.open(image_path2).convert('RGB')
hist1 = img1.histogram()
hist2 = img2.histogram()
return sum(1 - (0 if l == r else float(abs(l - r)) / max(l, r)) for l, r in zip(hist1, hist2)) / len(hist1)
def are_images_similar(image_path1, image_path2, threshold=0.99):
similarity = histogram_compare(image_path1, image_path2)
return similarity > threshold
print(are_images_similar(image1, image2))
四、特征点匹配
特征点匹配通过提取图片中的特征点,然后比较特征点的匹配情况来判断图片相似度。这种方法适用于图像内容相似但存在变形、旋转等情况的比较。
1、ORB特征点匹配
import cv2
def orb_feature_matching(image_path1, image_path2, threshold=10):
img1 = cv2.imread(image_path1, 0)
img2 = cv2.imread(image_path2, 0)
orb = cv2.ORB_create()
kp1, des1 = orb.detectAndCompute(img1, None)
kp2, des2 = orb.detectAndCompute(img2, None)
bf = cv2.BFMatcher(cv2.NORM_HAMMING, crossCheck=True)
matches = bf.match(des1, des2)
matches = sorted(matches, key = lambda x:x.distance)
return len(matches) > threshold
print(orb_feature_matching(image1, image2))
2、SIFT特征点匹配
def sift_feature_matching(image_path1, image_path2, threshold=10):
img1 = cv2.imread(image_path1, 0)
img2 = cv2.imread(image_path2, 0)
sift = cv2.SIFT_create()
kp1, des1 = sift.detectAndCompute(img1, None)
kp2, des2 = sift.detectAndCompute(img2, None)
bf = cv2.BFMatcher()
matches = bf.knnMatch(des1, des2, k=2)
good_matches = [m for m, n in matches if m.distance < 0.75 * n.distance]
return len(good_matches) > threshold
print(sift_feature_matching(image1, image2))
通过以上几种方法,我们可以根据不同的需求选择合适的图片相似度判断方法。哈希比较适用于快速判断图片相似度,像素比较适用于完全相同的图片比较,直方图比较适用于色彩分布相似的图片比较,特征点匹配适用于图像内容相似但存在变形、旋转等情况的比较。结合这些方法,我们可以更准确地判断两张图片是否相同。
相关问答FAQs:
如何使用Python比较两张图片的相似度?
在Python中,可以使用多种库来比较图片的相似度。常用的库包括OpenCV、PIL(Pillow)和scikit-image等。通过计算图片的直方图、结构相似性(SSIM)或使用特征匹配的方法,可以有效判断两张图片是否相似。具体的实现方式可以参考相关库的文档。
在Python中如何处理不同格式的图片进行比较?
处理不同格式的图片时,可以使用PIL库将其转换为统一的格式(如JPEG或PNG)。转换后,利用OpenCV或其他库进行比较。确保在比较之前对图片进行调整,包括大小和色彩模式,以确保结果的准确性。
Python中有哪些库可以帮助我实现图片对比功能?
Python中有许多强大的库可以帮助实现图片对比功能。OpenCV是一个广泛使用的计算机视觉库,提供了多种图像处理功能。Pillow是一个友好的图像处理库,适合快速处理和比较图片。此外,scikit-image库也提供了许多图像处理算法,适合进行更复杂的比较和分析工作。根据需求选择合适的库可以提升开发效率。
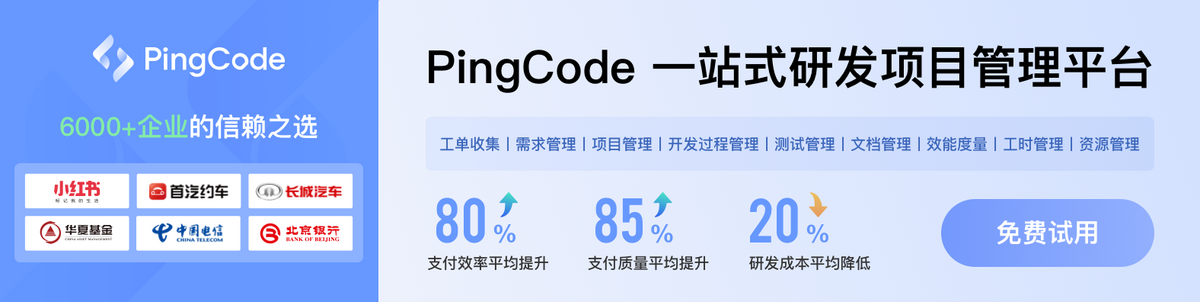