如何用Python画五星红旗
要用Python画五星红旗,可以使用matplotlib库、turtle库、PIL库。本文将详细介绍如何使用这三个库来绘制五星红旗,并对其中的matplotlib库进行详细描述。
要绘制五星红旗,首先需要理解其设计规范。五星红旗由一个大五角星和四个小五角星组成,所有星星都为黄色,位于红色背景上。大五角星位于旗帜左上角,小五角星围绕大五角星呈弧形排列。大五角星的中心距离旗帜左边和上边各1/10旗帜的长度,小五角星的中心分别距离旗帜左边2/10、3/10和4/10旗帜的长度,距离旗帜上边1/10、2/10和3/10旗帜的高度。
一、使用Matplotlib库绘制五星红旗
matplotlib是Python中一个强大的绘图库,广泛用于数据可视化。绘制五星红旗的步骤如下:
1.1、安装matplotlib库
在开始绘制之前,需要确保已经安装了matplotlib库。可以通过以下命令安装:
pip install matplotlib
1.2、绘制五星红旗的代码
以下是使用matplotlib绘制五星红旗的完整代码:
import matplotlib.pyplot as plt
import matplotlib.patches as patches
def draw_star(ax, x, y, size, angle=0):
star = patches.RegularPolygon((x, y), numVertices=5, radius=size, orientation=angle, color='yellow')
ax.add_patch(star)
def draw_flag():
fig, ax = plt.subplots()
# 绘制红色背景
ax.add_patch(patches.Rectangle((0, 0), 30, 20, color='red'))
# 绘制大五角星
draw_star(ax, 5, 15, 3)
# 绘制四个小五角星
small_star_coords = [(10, 18), (12, 16), (12, 12), (10, 10)]
for coord in small_star_coords:
draw_star(ax, coord[0], coord[1], 1, angle=0.5)
ax.set_xlim(0, 30)
ax.set_ylim(0, 20)
plt.axis('off')
plt.show()
draw_flag()
1.3、代码详细解释
- 创建绘图板: 使用
fig, ax = plt.subplots()
创建一个绘图板。 - 绘制红色背景: 使用
ax.add_patch(patches.Rectangle((0, 0), 30, 20, color='red'))
绘制红色背景。 - 绘制大五角星: 定义一个函数
draw_star
,使用patches.RegularPolygon
函数绘制五角星,并将其位置设置在(5, 15)。 - 绘制四个小五角星: 定义小五角星的坐标
small_star_coords
,使用循环调用draw_star
函数绘制小五角星。 - 设置绘图范围和隐藏坐标轴: 使用
ax.set_xlim(0, 30)
和ax.set_ylim(0, 20)
设置绘图范围,使用plt.axis('off')
隐藏坐标轴。
二、使用Turtle库绘制五星红旗
Turtle库是Python中的一个标准库,适用于绘制简单图形和动画。以下是使用Turtle库绘制五星红旗的步骤:
2.1、安装Turtle库
Turtle库是Python的标准库,无需额外安装,只需要在代码中导入即可:
import turtle
2.2、绘制五星红旗的代码
以下是使用Turtle库绘制五星红旗的完整代码:
import turtle
def draw_star(x, y, length):
turtle.penup()
turtle.goto(x, y)
turtle.pendown()
turtle.begin_fill()
for _ in range(5):
turtle.forward(length)
turtle.right(144)
turtle.end_fill()
def draw_flag():
turtle.speed(0)
turtle.penup()
turtle.goto(-150, 100)
turtle.pendown()
turtle.color('red')
turtle.begin_fill()
turtle.forward(300)
turtle.right(90)
turtle.forward(200)
turtle.right(90)
turtle.forward(300)
turtle.right(90)
turtle.forward(200)
turtle.end_fill()
turtle.color('yellow')
draw_star(-120, 70, 50)
small_star_coords = [(-90, 90), (-70, 70), (-70, 40), (-90, 20)]
for coord in small_star_coords:
draw_star(coord[0], coord[1], 20)
turtle.hideturtle()
turtle.done()
draw_flag()
2.3、代码详细解释
- 创建画布: 使用
turtle.speed(0)
设置绘图速度。 - 绘制红色背景: 使用
turtle.color('red')
设置颜色为红色,使用turtle.begin_fill()
和turtle.end_fill()
填充颜色。 - 绘制大五角星: 定义一个函数
draw_star
,使用turtle.forward
和turtle.right
绘制五角星,并设置其位置在(-120, 70)。 - 绘制四个小五角星: 定义小五角星的坐标
small_star_coords
,使用循环调用draw_star
函数绘制小五角星。 - 隐藏画笔: 使用
turtle.hideturtle()
隐藏画笔。
三、使用PIL库绘制五星红旗
PIL(Python Imaging Library)是Python中一个强大的图像处理库,适用于处理图像文件和绘制复杂图形。以下是使用PIL库绘制五星红旗的步骤:
3.1、安装PIL库
PIL库已经被Pillow库所取代,可以通过以下命令安装Pillow:
pip install pillow
3.2、绘制五星红旗的代码
以下是使用Pillow库绘制五星红旗的完整代码:
from PIL import Image, ImageDraw
def draw_star(draw, x, y, size):
points = []
for i in range(5):
angle = i * 144 - 90
x_point = x + size * cos(radians(angle))
y_point = y + size * sin(radians(angle))
points.append((x_point, y_point))
draw.polygon(points, fill='yellow')
def draw_flag():
width, height = 300, 200
image = Image.new('RGB', (width, height), 'red')
draw = ImageDraw.Draw(image)
draw_star(draw, 60, 40, 30)
small_star_coords = [(120, 20), (140, 40), (140, 80), (120, 100)]
for coord in small_star_coords:
draw_star(draw, coord[0], coord[1], 10)
image.show()
draw_flag()
3.3、代码详细解释
- 创建图像对象: 使用
Image.new('RGB', (width, height), 'red')
创建一个红色背景的图像对象。 - 绘制大五角星: 定义一个函数
draw_star
,使用ImageDraw.Draw.polygon
函数绘制五角星,并设置其位置在(60, 40)。 - 绘制四个小五角星: 定义小五角星的坐标
small_star_coords
,使用循环调用draw_star
函数绘制小五角星。 - 显示图像: 使用
image.show()
显示绘制好的图像。
四、总结
绘制五星红旗的方法有很多,不同的方法适用于不同的场景。使用matplotlib库、turtle库、PIL库都可以实现五星红旗的绘制,其中matplotlib库适用于数据可视化,turtle库适用于绘制简单图形和动画,PIL库适用于处理图像文件和绘制复杂图形。在实际应用中,可以根据具体需求选择合适的绘图库。希望本文对你有所帮助,并能在实际编程中灵活运用这些方法。
相关问答FAQs:
如何在Python中绘制五星红旗的步骤是什么?
绘制五星红旗可以使用Python的图形库,如Matplotlib或Turtle。首先,选择一个库并安装它。然后,设置画布背景为红色,接着使用图形绘制工具绘制五颗星星,分别放置在适当的位置。最后,调整星星的大小和颜色,确保它们的形状和位置符合五星红旗的设计要求。
使用哪种Python库最适合绘制五星红旗?
对于绘制五星红旗,Matplotlib和Turtle都是不错的选择。Matplotlib适合用于创建静态图形,提供丰富的绘图功能。而Turtle则更适合初学者,能够通过简单的命令来控制画笔绘图,适合教育和趣味项目。根据个人需求和编程水平,可以选择最适合的库。
绘制五星红旗时,如何确保星星的比例和位置准确?
为了确保星星的比例和位置准确,建议使用坐标系进行绘图。可以先设定一个标准的比例,例如星星的大小与国旗的尺寸比率,接着根据五星红旗的设计规范,计算出每颗星星的中心坐标。这种方法能够帮助保持绘制作品的准确性与美观性。
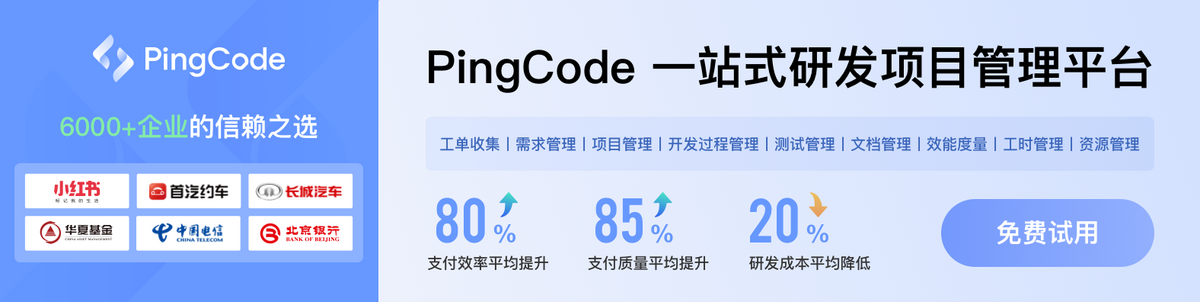