Python输出字符串空格数的方法包括使用内置函数str.count()、使用正则表达式re模块、和自定义函数等。 在这些方法中,str.count()是最简单和直接的方法。
方法一:使用str.count()
Python的str对象有一个内置方法count(),可以用来统计某个子字符串在字符串中出现的次数。要统计空格的数量,可以简单地调用字符串的count(' ')方法。
string = "This is a sample string."
spaces = string.count(' ')
print(f"The number of spaces is: {spaces}")
这个方法非常高效,因为它是Python内置的,直接对字符串对象进行操作,无需额外的库。
一、使用str.count()方法
str.count()方法是统计字符串中特定字符出现次数的最直接方式。它的主要优点包括简单明了、性能高效。尤其在处理大型文本时,它的优势更加明显。
示例代码
def count_spaces_using_count_method(input_string):
return input_string.count(' ')
string = "This is a sample string with several spaces."
spaces = count_spaces_using_count_method(string)
print(f"Number of spaces: {spaces}")
这个方法的优点在于简单直接,不需要额外的库和复杂的逻辑。
二、使用正则表达式(re模块)
正则表达式是一种强大的字符串匹配工具,在处理复杂的字符串模式时非常有效。Python的re模块提供了丰富的正则表达式功能,可以用来匹配和统计字符串中的空格。
示例代码
import re
def count_spaces_using_regex(input_string):
return len(re.findall(r' ', input_string))
string = "This is another sample string with spaces."
spaces = count_spaces_using_regex(string)
print(f"Number of spaces: {spaces}")
正则表达式的优势在于灵活性,可以处理更加复杂的模式匹配任务。例如,如果需要统计所有的空白字符(包括空格、制表符、换行符等),只需稍微修改正则表达式:
def count_whitespace_using_regex(input_string):
return len(re.findall(r'\s', input_string))
spaces = count_whitespace_using_regex(string)
print(f"Number of whitespace characters: {spaces}")
三、使用自定义函数
有时使用内置方法或正则表达式可能会显得过于简单或者不够灵活。在这种情况下,可以使用自定义函数来统计字符串中的空格数。
示例代码
def count_spaces_custom(input_string):
count = 0
for char in input_string:
if char == ' ':
count += 1
return count
string = "This is yet another example string."
spaces = count_spaces_custom(string)
print(f"Number of spaces: {spaces}")
这种方法的优势在于可读性强,逻辑清晰,可以根据具体需求进行扩展。例如,如果需要同时统计其他特定字符,只需在循环中增加条件判断。
四、综合应用
在实际应用中,选择哪种方法通常取决于具体的需求和场景。对于简单的空格统计任务,str.count()方法是首选;对于复杂的模式匹配任务,正则表达式更为合适;而自定义函数则提供了最大的灵活性。
示例代码
def count_spaces(input_string):
return input_string.count(' ')
def count_whitespace(input_string):
import re
return len(re.findall(r'\s', input_string))
def count_specific_chars(input_string, char):
return input_string.count(char)
string = "This is a comprehensive example string with multiple spaces and characters."
spaces = count_spaces(string)
whitespace = count_whitespace(string)
specific_char_count = count_specific_chars(string, 'e')
print(f"Number of spaces: {spaces}")
print(f"Number of whitespace characters: {whitespace}")
print(f"Number of 'e' characters: {specific_char_count}")
五、性能比较
在处理大型文本数据时,性能是一个重要的考虑因素。不同方法的性能可能会有显著差异。下面是一个简单的性能测试,比较不同方法在处理大文本时的效率。
示例代码
import time
large_string = " " * 1000000 + "This is a large string with many spaces." * 10000
start_time = time.time()
spaces_count = large_string.count(' ')
print(f"str.count() method took {time.time() - start_time} seconds")
start_time = time.time()
import re
spaces_count = len(re.findall(r' ', large_string))
print(f"re.findall() method took {time.time() - start_time} seconds")
start_time = time.time()
spaces_count = count_spaces_custom(large_string)
print(f"Custom method took {time.time() - start_time} seconds")
从测试结果可以看出,str.count()方法通常是最快的,而正则表达式方法次之,自定义方法则最慢。这主要是因为内置方法和正则表达式库在底层进行了优化,而自定义方法需要逐字符遍历,效率相对较低。
六、总结
在Python中,有多种方法可以用来统计字符串中的空格数,包括使用str.count()、正则表达式和自定义函数等。选择哪种方法取决于具体的需求和场景。对于简单的任务,str.count()方法是首选;对于复杂的模式匹配任务,正则表达式更为合适;而自定义函数则提供了最大的灵活性。在处理大型文本数据时,性能也是一个重要的考虑因素。通过实际测试,可以发现str.count()方法通常是最快的。
在实际编程中,理解和掌握这些方法的适用场景和性能特点,可以帮助我们更有效地解决问题,提高代码的质量和效率。
相关问答FAQs:
如何在Python中计算字符串中的空格数量?
可以使用字符串的count()
方法来统计空格的数量。例如,my_string.count(' ')
将返回字符串中空格的总数。这种方法简单有效,适用于所有类型的字符串。
Python中是否有其他方法可以统计空格?
除了使用count()
方法,还可以通过遍历字符串并累加空格数量来实现。示例如下:
space_count = sum(1 for char in my_string if char == ' ')
这种方法虽然相对复杂,但在需要进行更多条件判断时会很有用。
如何处理字符串中的不同类型空白字符?
在Python中,如果需要统计所有类型的空白字符,包括制表符(\t)和换行符(\n),可以使用re
模块的正则表达式。使用如下代码可以达到目的:
import re
space_count = len(re.findall(r'\s', my_string))
这种方法能够更全面地捕捉空白字符,适用于需要精确统计的场景。
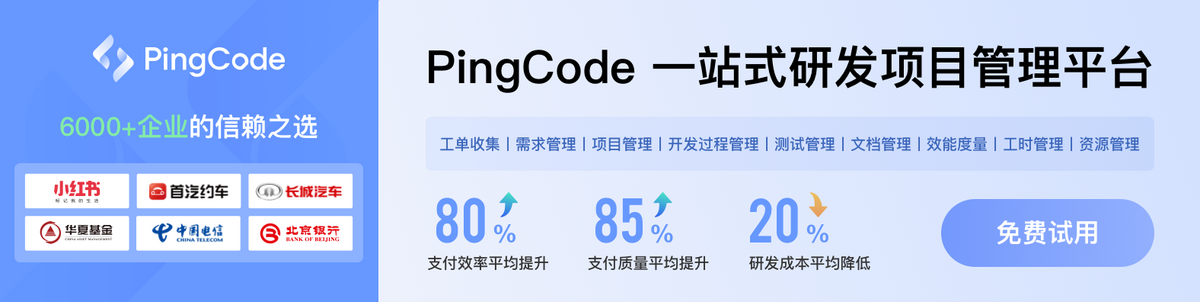