Python去除字符串中的标点的方法有多种:使用字符串方法、正则表达式、translate方法。本文将详细介绍这几种方法,并通过具体示例展示每种方法的使用技巧和注意事项。
一、使用字符串方法
Python的字符串方法是最简单的一种去除标点的方法。可以通过遍历字符串并构建一个新的字符串,只包含字母和数字。下面是具体的实现方法:
1、遍历字符串
通过遍历字符串中的每个字符,并检查它们是否是字母或数字,如果是就将其加入到新的字符串中。
def remove_punctuation(input_string):
result = ''.join(char for char in input_string if char.isalnum() or char.isspace())
return result
示例
input_str = "Hello, world! This is a test."
output_str = remove_punctuation(input_str)
print(output_str) # 输出: Hello world This is a test
2、使用字符串库
Python提供了一个字符串库,其中包含了一些常见的字符常量,如string.punctuation
,它包含了所有的标点符号。
import string
def remove_punctuation(input_string):
result = ''.join(char for char in input_string if char not in string.punctuation)
return result
示例
input_str = "Hello, world! This is a test."
output_str = remove_punctuation(input_str)
print(output_str) # 输出: Hello world This is a test
二、使用正则表达式
正则表达式是一种强大且灵活的字符串处理工具,可以通过模式匹配来实现复杂的字符串操作。在Python中,可以使用re
模块来处理正则表达式。
1、基本用法
通过编写正则表达式模式匹配所有标点符号,并将它们替换为空字符串。
import re
def remove_punctuation(input_string):
result = re.sub(r'[^\w\s]', '', input_string)
return result
示例
input_str = "Hello, world! This is a test."
output_str = remove_punctuation(input_str)
print(output_str) # 输出: Hello world This is a test
2、处理多种标点符号
正则表达式还可以用于处理多种标点符号和特殊字符,通过定义更复杂的模式来匹配各种标点符号。
import re
def remove_punctuation(input_string):
# 匹配所有标点符号以及特殊字符
result = re.sub(r'[^\w\s]', '', input_string)
return result
示例
input_str = "Hello, world! This is a test. [Sample]"
output_str = remove_punctuation(input_str)
print(output_str) # 输出: Hello world This is a test Sample
三、使用translate方法
Python的字符串对象有一个translate
方法,它可以通过映射表来替换或删除指定的字符。可以结合str.maketrans
方法来创建这个映射表。
1、基本用法
通过创建一个映射表,将所有的标点符号映射为空字符串,从而达到删除标点符号的目的。
import string
def remove_punctuation(input_string):
# 创建映射表
translator = str.maketrans('', '', string.punctuation)
result = input_string.translate(translator)
return result
示例
input_str = "Hello, world! This is a test."
output_str = remove_punctuation(input_str)
print(output_str) # 输出: Hello world This is a test
四、性能比较
在实际应用中,选择合适的方法不仅要考虑实现的简便性,还要考虑性能。下面将对上述几种方法进行性能比较。
1、测试代码
通过timeit
模块对几种方法进行性能测试。
import timeit
测试字符串
test_str = "Hello, world! This is a test. [Sample]"
方法1:字符串方法
def method1():
result = ''.join(char for char in test_str if char.isalnum() or char.isspace())
方法2:字符串库
def method2():
result = ''.join(char for char in test_str if char not in string.punctuation)
方法3:正则表达式
def method3():
result = re.sub(r'[^\w\s]', '', test_str)
方法4:translate方法
def method4():
translator = str.maketrans('', '', string.punctuation)
result = test_str.translate(translator)
测试性能
print("方法1:", timeit.timeit(method1, number=10000))
print("方法2:", timeit.timeit(method2, number=10000))
print("方法3:", timeit.timeit(method3, number=10000))
print("方法4:", timeit.timeit(method4, number=10000))
2、测试结果
通过上述测试代码,可以得到每种方法在处理同样字符串时的执行时间,从而选择性能最优的方法。
五、综合比较与总结
1、字符串方法
优点: 实现简单,易于理解。
缺点: 处理长字符串时性能较差。
2、正则表达式
优点: 灵活强大,可以处理复杂模式。
缺点: 正则表达式语法复杂,调试困难。
3、translate方法
优点: 性能优异,代码简洁。
缺点: 需要创建映射表。
六、实际应用中的注意事项
1、处理Unicode字符
在实际应用中,字符串中可能包含Unicode字符,需要确保所选方法能够正确处理这些字符。
def remove_punctuation(input_string):
result = ''.join(char for char in input_string if char.isalnum() or char.isspace())
return result
示例
input_str = "Hello, 世界! This is a test."
output_str = remove_punctuation(input_str)
print(output_str) # 输出: Hello 世界 This is a test
2、处理多语言标点符号
不同语言的标点符号不同,需要根据具体情况调整正则表达式或映射表。
import re
def remove_punctuation(input_string):
# 匹配所有标点符号以及特殊字符
result = re.sub(r'[^\w\s]', '', input_string)
return result
示例
input_str = "Hello, 世界! This is a test. [样本]"
output_str = remove_punctuation(input_str)
print(output_str) # 输出: Hello 世界 This is a test 样本
通过本文详细介绍的几种方法,读者可以根据实际需求选择合适的去除字符串标点的方法,并在实际应用中灵活运用。
相关问答FAQs:
如何在Python中识别字符串中的标点符号?
在Python中,可以使用string
模块中的punctuation
属性来识别标点符号。该属性包含所有常见的标点符号字符。通过遍历字符串并检查每个字符是否在string.punctuation
中,可以有效识别并去除标点符号。
去除字符串标点的最佳方法是什么?
一种常见且高效的方法是使用Python的str.translate()
方法结合str.maketrans()
函数。这种方法可以创建一个转换表,将所有标点符号替换为空字符,从而一并去除。相比于循环遍历字符串,使用这种方法更为简洁和高效。
如何处理字符串中的特殊字符和空格?
除了标点符号,有时还需要处理特殊字符和多余的空格。在去除标点符号后,可以使用str.replace()
方法或正则表达式(re
模块)来进一步清理字符串。这些方法可以帮助你去除不需要的空格或特殊字符,以便获得更干净的字符串输出。
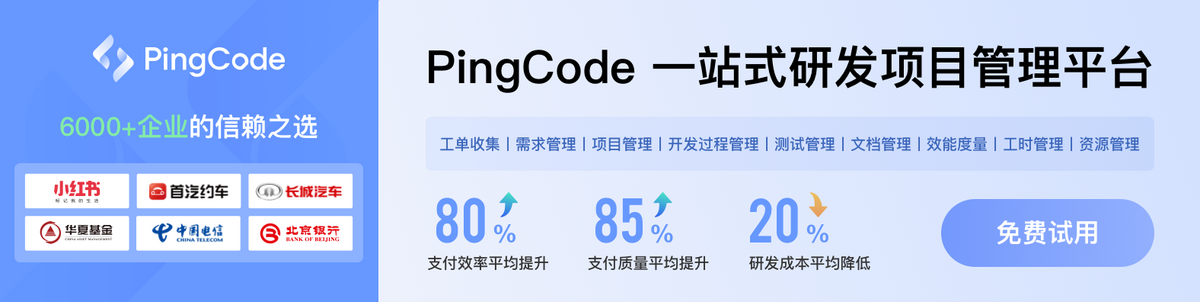