如何使用Python来连MySQL数据库
要使用Python连接MySQL数据库,你需要使用适当的库,如mysql-connector-python、PyMySQL、SQLAlchemy。这些库提供了不同的功能和接口,使得与MySQL数据库的交互变得简便。以下将详细讲解使用这些库连接和操作MySQL数据库的步骤。
一、安装所需库
在开始之前,你需要安装必要的Python库。可以使用pip来安装这些库。以下是安装的命令:
pip install mysql-connector-python
pip install pymysql
pip install sqlalchemy
二、使用mysql-connector-python连接MySQL
mysql-connector-python是一个官方的MySQL驱动,适用于Python。它提供了一个简单的接口来连接和操作MySQL数据库。
1、连接数据库
首先,导入mysql.connector模块,并使用它的connect方法来连接数据库。你需要提供数据库的主机地址、用户名、密码和数据库名称。
import mysql.connector
创建连接
conn = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
创建游标
cursor = conn.cursor()
2、执行SQL查询
一旦连接建立,你可以使用cursor对象来执行SQL查询。
# 执行查询
cursor.execute("SELECT * FROM yourtable")
获取结果
result = cursor.fetchall()
for row in result:
print(row)
3、关闭连接
当你完成操作后,确保关闭游标和连接。
cursor.close()
conn.close()
三、使用PyMySQL连接MySQL
PyMySQL是另一个流行的库,用于在Python中连接MySQL数据库。它的使用方式与mysql-connector-python类似,但有一些细微的差别。
1、连接数据库
首先,导入pymysql模块,并使用它的connect方法来连接数据库。
import pymysql
创建连接
conn = pymysql.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
创建游标
cursor = conn.cursor()
2、执行SQL查询
使用cursor对象执行SQL查询。
# 执行查询
cursor.execute("SELECT * FROM yourtable")
获取结果
result = cursor.fetchall()
for row in result:
print(row)
3、关闭连接
确保关闭游标和连接。
cursor.close()
conn.close()
四、使用SQLAlchemy连接MySQL
SQLAlchemy是一个强大的Python SQL工具包和ORM库。它提供了一个高层次的API来管理数据库连接和执行SQL查询。
1、连接数据库
首先,导入create_engine函数,并使用它来创建一个数据库引擎。
from sqlalchemy import create_engine
创建引擎
engine = create_engine("mysql+pymysql://yourusername:yourpassword@localhost/yourdatabase")
2、执行SQL查询
使用引擎对象的connect方法建立连接,并执行SQL查询。
# 创建连接
conn = engine.connect()
执行查询
result = conn.execute("SELECT * FROM yourtable")
获取结果
for row in result:
print(row)
3、关闭连接
确保关闭连接。
conn.close()
五、使用ORM进行数据库操作
ORM(对象关系映射)使得在Python中操作数据库更加方便。SQLAlchemy提供了强大的ORM功能。
1、定义模型类
首先,定义一个模型类来映射到数据库表。
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy import Column, Integer, String
Base = declarative_base()
class YourTable(Base):
__tablename__ = 'yourtable'
id = Column(Integer, primary_key=True)
name = Column(String)
age = Column(Integer)
2、创建表
使用引擎对象和Base类的metadata.create_all方法创建表。
Base.metadata.create_all(engine)
3、添加数据
使用SQLAlchemy的会话管理器(Session)来添加数据。
from sqlalchemy.orm import sessionmaker
创建会话
Session = sessionmaker(bind=engine)
session = Session()
添加数据
new_entry = YourTable(name="John Doe", age=30)
session.add(new_entry)
session.commit()
4、查询数据
使用会话对象来查询数据。
# 查询数据
results = session.query(YourTable).all()
for row in results:
print(row.name, row.age)
5、更新和删除数据
你可以使用会话对象来更新和删除数据。
# 更新数据
entry = session.query(YourTable).filter_by(name="John Doe").first()
entry.age = 31
session.commit()
删除数据
session.delete(entry)
session.commit()
六、最佳实践
1、使用上下文管理器
使用上下文管理器可以确保在操作完成后自动关闭游标和连接。
import mysql.connector
from mysql.connector import Error
try:
conn = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
if conn.is_connected():
cursor = conn.cursor()
cursor.execute("SELECT * FROM yourtable")
result = cursor.fetchall()
for row in result:
print(row)
except Error as e:
print(f"Error: {e}")
finally:
if conn.is_connected():
cursor.close()
conn.close()
2、使用参数化查询
使用参数化查询可以防止SQL注入攻击。
query = "SELECT * FROM yourtable WHERE id = %s"
cursor.execute(query, (1,))
通过以上步骤,你可以使用Python与MySQL数据库进行连接和操作。选择适合你需求的库和方法,可以大大简化你的数据库管理任务。
相关问答FAQs:
如何在Python中安装MySQL连接库?
要连接MySQL数据库,您需要安装一个适合的库,通常使用mysql-connector-python
或PyMySQL
。您可以通过运行以下命令来安装它们:
pip install mysql-connector-python
或
pip install PyMySQL
安装成功后,您就可以在Python脚本中导入这些库,开始与MySQL数据库进行交互。
在Python中如何建立与MySQL的连接?
建立连接的步骤相对简单。您需要提供数据库的主机名、用户名、密码和数据库名。例如,使用mysql-connector-python
库的代码如下:
import mysql.connector
connection = mysql.connector.connect(
host='localhost',
user='your_username',
password='your_password',
database='your_database'
)
确保替换相应的参数,以便正确连接到您的数据库。
如何在Python中执行SQL查询并处理结果?
执行SQL查询后,可以使用游标对象来处理结果。以下是一个示例,展示了如何执行查询并获取结果:
cursor = connection.cursor()
cursor.execute("SELECT * FROM your_table")
results = cursor.fetchall()
for row in results:
print(row)
cursor.close()
connection.close()
在这个例子中,fetchall()
方法用于获取查询的所有结果,您可以根据需要对结果进行处理。
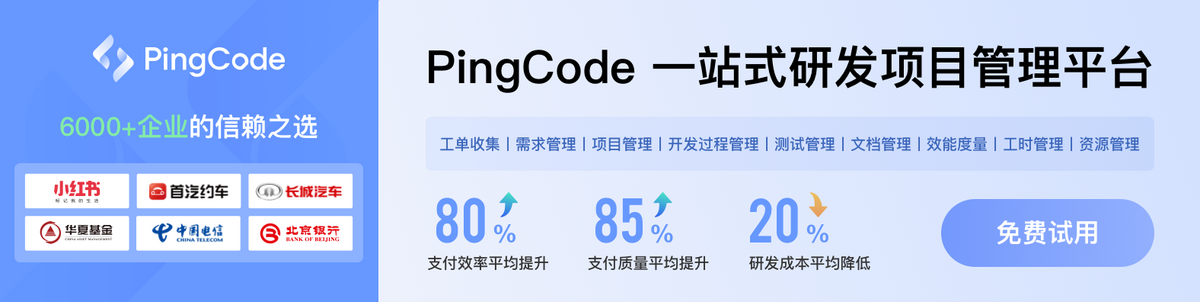