在Python中创建多线程一起启动的方法有多种,包括使用threading
模块、concurrent.futures
模块以及multiprocessing
模块。本文将详细介绍这些方法,并提供实用的代码示例和注意事项。
一、使用threading
模块
1. 基本概念
Python的threading
模块提供了一个简单的方式来创建和管理线程。线程是一个独立的执行流,可以用于并行执行任务,从而提高程序的效率。
2. 创建线程
要创建一个线程,可以使用threading.Thread
类。该类的构造函数接受以下主要参数:
target
: 线程的目标函数,即线程执行的任务。args
: 传递给目标函数的位置参数。kwargs
: 传递给目标函数的关键字参数。
import threading
def task(name):
print(f"Task {name} is running")
创建多个线程
threads = []
for i in range(5):
thread = threading.Thread(target=task, args=(i,))
threads.append(thread)
启动所有线程
for thread in threads:
thread.start()
等待所有线程完成
for thread in threads:
thread.join()
在上述代码中,我们创建了五个线程,并且通过调用start()
方法启动它们。最后,通过调用join()
方法来确保主线程等待所有线程完成。
3. 使用继承创建线程
除了直接使用threading.Thread
类,还可以通过继承该类来创建自定义的线程类。
import threading
class MyThread(threading.Thread):
def __init__(self, name):
super().__init__()
self.name = name
def run(self):
print(f"Task {self.name} is running")
创建多个线程
threads = []
for i in range(5):
thread = MyThread(name=i)
threads.append(thread)
启动所有线程
for thread in threads:
thread.start()
等待所有线程完成
for thread in threads:
thread.join()
4. 线程同步
在多线程编程中,线程之间的同步是一个重要的问题。Python提供了多种同步机制,如Lock
、RLock
、Semaphore
等。
import threading
counter = 0
lock = threading.Lock()
def task():
global counter
for _ in range(1000):
with lock:
counter += 1
创建多个线程
threads = []
for i in range(5):
thread = threading.Thread(target=task)
threads.append(thread)
启动所有线程
for thread in threads:
thread.start()
等待所有线程完成
for thread in threads:
thread.join()
print(f"Final counter value: {counter}")
在上述代码中,我们使用Lock
对象来确保对共享资源counter
的操作是线程安全的。
二、使用concurrent.futures
模块
1. 基本概念
concurrent.futures
模块提供了一个高级接口,用于异步执行任务。该模块中有两个主要的类:ThreadPoolExecutor
和ProcessPoolExecutor
,分别用于管理线程池和进程池。
2. 使用ThreadPoolExecutor
ThreadPoolExecutor
类提供了一种简单的方式来创建和管理线程池。
from concurrent.futures import ThreadPoolExecutor
def task(name):
print(f"Task {name} is running")
创建线程池
with ThreadPoolExecutor(max_workers=5) as executor:
futures = [executor.submit(task, i) for i in range(5)]
等待所有线程完成
for future in futures:
future.result()
在上述代码中,我们使用ThreadPoolExecutor
来创建一个包含五个线程的线程池。通过调用submit()
方法,将任务提交到线程池中,并返回一个Future
对象。
3. 使用map
方法
ThreadPoolExecutor
类还提供了一个map
方法,用于将一个可迭代对象中的每个元素映射到一个线程任务中。
from concurrent.futures import ThreadPoolExecutor
def task(name):
print(f"Task {name} is running")
创建线程池
with ThreadPoolExecutor(max_workers=5) as executor:
results = executor.map(task, range(5))
4. 异常处理
在多线程编程中,异常处理是一个重要的问题。concurrent.futures
模块提供了简单的异常处理机制。
from concurrent.futures import ThreadPoolExecutor
def task(name):
if name == 3:
raise ValueError("An error occurred")
print(f"Task {name} is running")
创建线程池
with ThreadPoolExecutor(max_workers=5) as executor:
futures = [executor.submit(task, i) for i in range(5)]
等待所有线程完成并处理异常
for future in futures:
try:
future.result()
except Exception as e:
print(f"Exception: {e}")
在上述代码中,我们通过调用future.result()
方法来获取任务的结果,并捕获可能的异常。
三、使用multiprocessing
模块
1. 基本概念
multiprocessing
模块提供了创建和管理进程的接口。与线程不同,进程是独立的执行单元,每个进程都有自己的内存空间。
2. 创建进程
要创建一个进程,可以使用multiprocessing.Process
类。该类的构造函数接受以下主要参数:
target
: 进程的目标函数,即进程执行的任务。args
: 传递给目标函数的位置参数。kwargs
: 传递给目标函数的关键字参数。
import multiprocessing
def task(name):
print(f"Task {name} is running")
创建多个进程
processes = []
for i in range(5):
process = multiprocessing.Process(target=task, args=(i,))
processes.append(process)
启动所有进程
for process in processes:
process.start()
等待所有进程完成
for process in processes:
process.join()
在上述代码中,我们创建了五个进程,并且通过调用start()
方法启动它们。最后,通过调用join()
方法来确保主进程等待所有进程完成。
3. 使用Pool
multiprocessing
模块提供了Pool
类,用于管理进程池。
import multiprocessing
def task(name):
print(f"Task {name} is running")
创建进程池
with multiprocessing.Pool(processes=5) as pool:
pool.map(task, range(5))
4. 进程间通信
multiprocessing
模块提供了多种进程间通信的机制,如Queue
、Pipe
等。
import multiprocessing
def task(queue, name):
queue.put(f"Task {name} is running")
创建队列
queue = multiprocessing.Queue()
创建多个进程
processes = []
for i in range(5):
process = multiprocessing.Process(target=task, args=(queue, i))
processes.append(process)
启动所有进程
for process in processes:
process.start()
等待所有进程完成
for process in processes:
process.join()
获取队列中的数据
while not queue.empty():
print(queue.get())
在上述代码中,我们使用Queue
对象来实现进程间通信。
四、线程和进程的选择
1. 线程的优点和缺点
优点:
- 轻量级:线程比进程更轻量级,创建和销毁开销较小。
- 共享内存:线程共享相同的内存空间,数据传递更方便。
缺点:
- 全局解释器锁(GIL):Python的GIL限制了同一时间只有一个线程在执行字节码,导致多线程在CPU密集型任务中的性能提升有限。
- 线程安全:由于线程共享内存,必须使用锁等同步机制来防止数据竞争。
2. 进程的优点和缺点
优点:
- 独立内存空间:进程有独立的内存空间,不受GIL的限制,适合CPU密集型任务。
- 更高的稳定性:一个进程的崩溃不会影响其他进程,提高了程序的稳定性。
缺点:
- 开销较大:进程的创建和销毁开销较大,进程间通信也比线程复杂。
- 内存开销:每个进程都有独立的内存空间,导致内存开销较大。
五、实际应用场景
1. I/O密集型任务
对于I/O密集型任务,如网络请求、文件读写等,使用多线程可以显著提高性能。
import threading
import requests
def fetch_url(url):
response = requests.get(url)
print(f"Fetched {url} with status {response.status_code}")
urls = ["https://www.example.com", "https://www.python.org", "https://www.github.com"]
创建多个线程
threads = []
for url in urls:
thread = threading.Thread(target=fetch_url, args=(url,))
threads.append(thread)
启动所有线程
for thread in threads:
thread.start()
等待所有线程完成
for thread in threads:
thread.join()
2. CPU密集型任务
对于CPU密集型任务,如数据处理、图像处理等,使用多进程可以显著提高性能。
import multiprocessing
def compute_square(n):
return n * n
numbers = range(1000000)
创建进程池
with multiprocessing.Pool(processes=4) as pool:
results = pool.map(compute_square, numbers)
print("Computation done")
六、性能优化
1. 使用合适的数据结构
在多线程或多进程编程中,选择合适的数据结构可以显著提高性能。比如,使用Queue
来实现线程安全的队列,使用Manager
来实现进程间共享数据。
2. 避免过多的上下文切换
上下文切换是多线程和多进程编程中的一个重要开销。通过减少线程或进程的数量,可以有效降低上下文切换的开销。
3. 使用异步编程
对于I/O密集型任务,异步编程是一种更高效的选择。Python提供了asyncio
模块来实现异步编程。
import asyncio
import aiohttp
async def fetch_url(session, url):
async with session.get(url) as response:
print(f"Fetched {url} with status {response.status}")
async def main():
async with aiohttp.ClientSession() as session:
tasks = [fetch_url(session, url) for url in ["https://www.example.com", "https://www.python.org", "https://www.github.com"]]
await asyncio.gather(*tasks)
asyncio.run(main())
七、总结
在Python中,创建多线程一起启动的方法有多种,包括使用threading
模块、concurrent.futures
模块和multiprocessing
模块。每种方法都有其优点和缺点,选择适合的方法可以根据具体的应用场景来决定。通过合理的设计和优化,可以显著提高程序的性能和稳定性。
相关问答FAQs:
在Python中,如何有效地创建和管理多个线程?
在Python中创建多线程可以使用threading
模块。你可以定义一个线程类,重写run
方法,然后创建多个线程的实例并使用start()
方法启动它们。为了有效管理线程,可以使用join()
方法确保主线程等待所有子线程完成。可以考虑使用ThreadPoolExecutor
来简化线程管理。
使用多线程时有哪些常见的注意事项?
使用多线程时,需要注意线程安全问题。多个线程可能会同时访问共享资源,导致数据不一致。可以使用锁(如threading.Lock
)来保护共享资源,确保同一时间只有一个线程可以访问这些资源。此外,避免使用过多的线程,以免造成上下文切换的开销。
在Python中,使用多线程的最佳实践是什么?
最佳实践包括合理设定线程数量,通常线程数不应超过CPU核心数的2倍。尽量避免在主线程中执行阻塞操作,以保持响应性。使用Queue
模块来在线程之间安全地传递数据,减少竞争条件。同时,考虑使用asyncio
库来处理IO密集型任务,这样可以提升应用的性能。
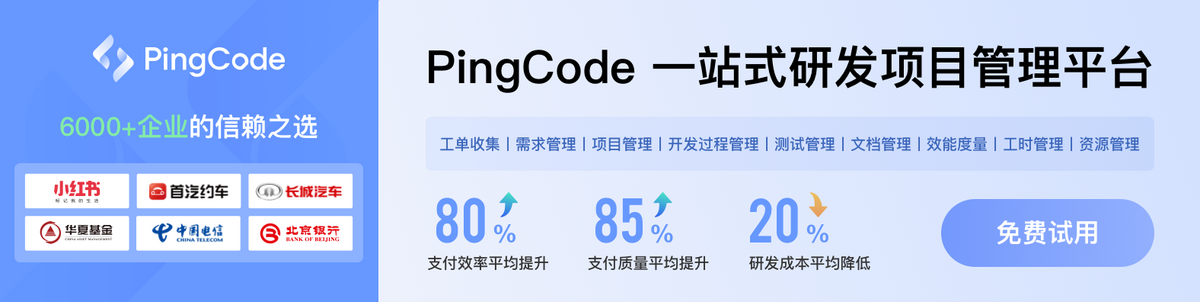