在Python中实现鼠标控制图片大小的方法有多种,最常用的方式包括使用图形用户界面(GUI)库如Tkinter、PyQt或Pygame。 本文将详细介绍如何使用这些库来实现鼠标控制图片大小的功能,重点将放在PyQt库上,因为它提供了更多的控件和功能,使得实现复杂的图形界面变得更为简单。具体步骤包括:加载图片、处理鼠标事件、调整图片大小并实时更新显示。
一、Tkinter中的实现方法
1、加载图片
在Tkinter中加载图片通常使用PhotoImage
或PIL库中的Image
模块。
from tkinter import Tk, Label, PhotoImage
from PIL import Image, ImageTk
root = Tk()
image = Image.open("path/to/your/image.jpg")
photo = ImageTk.PhotoImage(image)
label = Label(root, image=photo)
label.pack()
root.mainloop()
2、鼠标事件处理
在Tkinter中,可以使用bind
方法绑定鼠标事件。
def resize_image(event):
global image, photo, label
new_width = event.x
new_height = event.y
resized_image = image.resize((new_width, new_height), Image.ANTIALIAS)
photo = ImageTk.PhotoImage(resized_image)
label.config(image=photo)
label.image = photo
label.bind("<B1-Motion>", resize_image)
二、PyQt中的实现方法
1、加载图片
在PyQt中,可以使用QPixmap
来加载图片。
from PyQt5.QtWidgets import QApplication, QLabel, QMainWindow
from PyQt5.QtGui import QPixmap
from PyQt5.QtCore import Qt
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.image = QPixmap("path/to/your/image.jpg")
self.label = QLabel(self)
self.label.setPixmap(self.image)
self.setCentralWidget(self.label)
self.label.setMouseTracking(True)
self.setMouseTracking(True)
app = QApplication([])
window = MainWindow()
window.show()
app.exec_()
2、鼠标事件处理
在PyQt中,可以重写mouseMoveEvent
方法来处理鼠标事件。
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.image = QPixmap("path/to/your/image.jpg")
self.label = QLabel(self)
self.label.setPixmap(self.image)
self.setCentralWidget(self.label)
self.label.setMouseTracking(True)
self.setMouseTracking(True)
def mouseMoveEvent(self, event):
new_width = event.x()
new_height = event.y()
resized_image = self.image.scaled(new_width, new_height, Qt.KeepAspectRatio)
self.label.setPixmap(resized_image)
app = QApplication([])
window = MainWindow()
window.show()
app.exec_()
三、Pygame中的实现方法
1、加载图片
在Pygame中,加载图片使用pygame.image.load
方法。
import pygame
pygame.init()
screen = pygame.display.set_mode((800, 600))
image = pygame.image.load("path/to/your/image.jpg")
rect = image.get_rect()
screen.blit(image, rect)
pygame.display.flip()
2、鼠标事件处理
在Pygame中,可以使用事件循环来处理鼠标事件。
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.MOUSEMOTION:
new_width, new_height = event.pos
resized_image = pygame.transform.scale(image, (new_width, new_height))
screen.fill((0, 0, 0))
screen.blit(resized_image, (0, 0))
pygame.display.flip()
pygame.quit()
四、综合对比
1、易用性
Tkinter:适合简单的图形界面应用,容易上手,但功能较为基础。
PyQt:功能强大,适合复杂的图形界面应用,学习曲线稍陡。
Pygame:主要用于游戏开发,适合需要实时更新图形的应用。
2、性能
Tkinter:性能较低,适合小型应用。
PyQt:性能较高,适合大型应用。
Pygame:性能优异,适合需要高帧率的应用。
五、应用场景
1、Tkinter
适用于需要快速原型设计的小型应用,如简单的图形编辑工具或图片浏览器。
2、PyQt
适用于需要复杂交互和高级控件的大型应用,如图形编辑软件或数据可视化工具。
3、Pygame
适用于需要实时图形更新的应用,如游戏开发或实时数据监控。
六、结论
在Python中实现鼠标控制图片大小的方法多种多样,选择合适的库取决于应用的复杂度和性能需求。对于简单的应用,Tkinter是一个不错的选择,而对于复杂和大型的应用,PyQt更为适合。 如果需要实时图形更新,Pygame是不二之选。希望本文能为您提供一个全方位的参考,帮助您在项目中选择最合适的实现方法。
相关问答FAQs:
如何在Python中使用鼠标动态调整图片大小?
在Python中,可以使用Tkinter库来创建图形用户界面,并结合鼠标事件来实现动态调整图片大小的功能。通过绑定鼠标拖动事件,可以实时更新图片的显示尺寸。可以使用PIL库处理图片,以确保在调整大小时不会失去质量。
我需要安装哪些库来实现鼠标控制图片大小的功能?
要实现鼠标控制图片大小的功能,建议安装Tkinter和Pillow(PIL)库。Tkinter是Python自带的库,通常无需安装。Pillow可以通过pip安装,命令为pip install Pillow
。这两个库结合使用,可以轻松实现图片的加载、显示及大小调整。
如何处理图片缩放时的质量问题?
在调整图片大小时,确保使用Pillow库中的resize()
方法,并设置适当的抗锯齿参数,以保证缩放后的图片质量。可以选择不同的插值方法,例如Image.ANTIALIAS
,以获得更平滑的图像效果。这一点在处理高分辨率图片时尤为重要,能够有效减少失真现象。
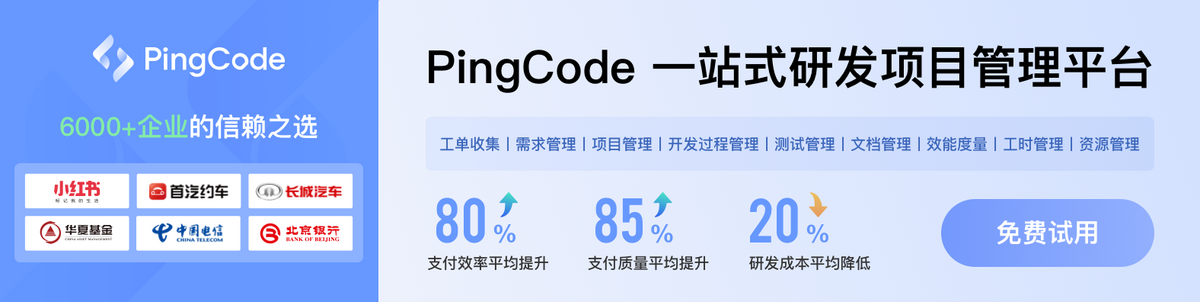