Python发送一个空文件夹的方法有多种:使用os模块创建文件夹、使用shutil模块压缩文件夹、使用smtplib模块发送邮件。下面将详细介绍如何使用这些方法来实现发送一个空文件夹的过程。
一、创建空文件夹
在发送空文件夹之前,我们首先需要创建一个空文件夹。Python的os模块提供了创建目录的功能。我们可以使用os.makedirs()
函数来创建一个空文件夹。
import os
def create_empty_folder(folder_path):
if not os.path.exists(folder_path):
os.makedirs(folder_path)
print(f"Empty folder '{folder_path}' created successfully.")
else:
print(f"Folder '{folder_path}' already exists.")
folder_path = 'empty_folder'
create_empty_folder(folder_path)
二、压缩空文件夹
为了便于传输,我们可以将空文件夹压缩成一个压缩文件。Python的shutil模块提供了make_archive()
函数,可以方便地将文件夹压缩成zip文件。
import shutil
def compress_folder(folder_path, output_filename):
shutil.make_archive(output_filename, 'zip', folder_path)
print(f"Folder '{folder_path}' compressed to '{output_filename}.zip' successfully.")
output_filename = 'empty_folder_compressed'
compress_folder(folder_path, output_filename)
三、通过邮件发送压缩文件夹
一旦我们有了压缩文件夹,我们可以通过电子邮件发送它。Python的smtplib模块可以用来发送电子邮件。为了发送附件,我们还需要使用email模块。
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.base import MIMEBase
from email import encoders
def send_email_with_attachment(smtp_server, port, sender_email, receiver_email, subject, body, attachment_path, password):
# Create a multipart message
msg = MIMEMultipart()
msg['From'] = sender_email
msg['To'] = receiver_email
msg['Subject'] = subject
# Attach the body with the msg instance
msg.attach(MIMEText(body, 'plain'))
# Open the file to be sent
attachment = open(attachment_path, "rb")
# Instance of MIMEBase and named as p
part = MIMEBase('application', 'octet-stream')
# To change the payload into encoded form
part.set_payload((attachment).read())
# Encode into base64
encoders.encode_base64(part)
part.add_header('Content-Disposition', f"attachment; filename= {os.path.basename(attachment_path)}")
# Attach the instance 'part' to instance 'msg'
msg.attach(part)
# Create SMTP session for sending the mail
with smtplib.SMTP(smtp_server, port) as server:
server.starttls() # Enable security
server.login(sender_email, password) # Login with the sender's email and password
text = msg.as_string()
server.sendmail(sender_email, receiver_email, text)
print(f"Email with attachment '{attachment_path}' sent successfully.")
Example usage
smtp_server = 'smtp.example.com'
port = 587
sender_email = 'you@example.com'
receiver_email = 'friend@example.com'
subject = 'Empty Folder Attachment'
body = 'Please find the attached empty folder.'
attachment_path = 'empty_folder_compressed.zip'
password = 'your-email-password'
send_email_with_attachment(smtp_server, port, sender_email, receiver_email, subject, body, attachment_path, password)
四、其他传输方法
除了通过电子邮件发送压缩文件夹之外,我们还可以通过其他方式传输空文件夹,例如使用FTP、SFTP或者通过云存储服务(如AWS S3、Google Drive等)。
1、通过FTP传输
我们可以使用Python的ftplib模块来通过FTP传输文件。
from ftplib import FTP
def upload_file_via_ftp(ftp_server, username, password, file_path, remote_path):
ftp = FTP(ftp_server)
ftp.login(user=username, passwd=password)
with open(file_path, 'rb') as file:
ftp.storbinary(f'STOR {remote_path}', file)
ftp.quit()
print(f"File '{file_path}' uploaded to FTP server at '{remote_path}' successfully.")
Example usage
ftp_server = 'ftp.example.com'
username = 'your-ftp-username'
password = 'your-ftp-password'
file_path = 'empty_folder_compressed.zip'
remote_path = 'remote_empty_folder_compressed.zip'
upload_file_via_ftp(ftp_server, username, password, file_path, remote_path)
2、通过SFTP传输
我们可以使用paramiko库来通过SFTP传输文件。
import paramiko
def upload_file_via_sftp(sftp_server, username, password, file_path, remote_path):
transport = paramiko.Transport((sftp_server, 22))
transport.connect(username=username, password=password)
sftp = paramiko.SFTPClient.from_transport(transport)
sftp.put(file_path, remote_path)
sftp.close()
transport.close()
print(f"File '{file_path}' uploaded to SFTP server at '{remote_path}' successfully.")
Example usage
sftp_server = 'sftp.example.com'
username = 'your-sftp-username'
password = 'your-sftp-password'
file_path = 'empty_folder_compressed.zip'
remote_path = 'remote_empty_folder_compressed.zip'
upload_file_via_sftp(sftp_server, username, password, file_path, remote_path)
3、通过云存储服务传输
我们也可以使用Boto3库将文件上传到AWS S3。
import boto3
def upload_file_to_s3(bucket_name, file_path, s3_path, aws_access_key, aws_secret_key):
s3 = boto3.client('s3', aws_access_key_id=aws_access_key, aws_secret_access_key=aws_secret_key)
s3.upload_file(file_path, bucket_name, s3_path)
print(f"File '{file_path}' uploaded to S3 bucket '{bucket_name}' at '{s3_path}' successfully.")
Example usage
bucket_name = 'your-s3-bucket'
file_path = 'empty_folder_compressed.zip'
s3_path = 'remote_empty_folder_compressed.zip'
aws_access_key = 'your-aws-access-key'
aws_secret_key = 'your-aws-secret-key'
upload_file_to_s3(bucket_name, file_path, s3_path, aws_access_key, aws_secret_key)
综上所述,通过创建、压缩和传输空文件夹的方法,可以方便地实现将空文件夹发送到其他计算机或服务器。在选择传输方法时,可以根据具体需求和环境选择合适的方式。
相关问答FAQs:
如何使用Python发送一个空文件夹?
在Python中,发送一个空文件夹并不直接,因为文件夹本身并不包含可发送的内容。然而,可以使用压缩文件的方式来实现这一目的。你可以使用shutil
模块的make_archive
方法将空文件夹压缩成一个ZIP或TAR文件,然后再发送这个压缩文件。
有哪些方法可以发送文件夹的内容而不是空文件夹?
如果你想发送文件夹内的所有文件而不是空文件夹,Python提供了多种方法来实现。例如,可以利用shutil
模块来复制文件夹及其内容到目标位置,或者使用zipfile
模块将文件夹压缩并发送。发送时,可以选择使用电子邮件、FTP或通过网络共享。
发送文件夹时,如何确保文件夹结构保持不变?
在发送文件夹时,保持文件夹结构不变是很重要的。压缩文件夹是一个有效的方法,因为它会保留文件夹的层级结构。使用shutil.make_archive
或zipfile.ZipFile
可以创建一个压缩包,发送后接收方解压缩时,文件夹结构会完整保留。此外,确保使用相对路径而非绝对路径可以帮助保持结构的一致性。
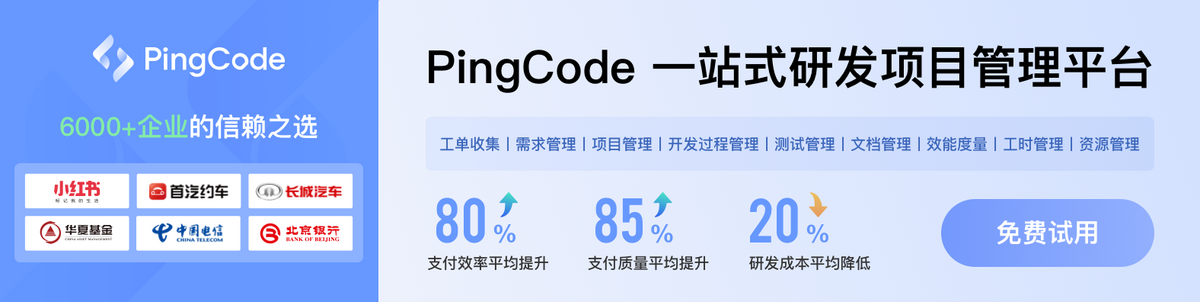