Python 替换字符串中的某个单词,可以使用字符串的内置方法 replace()
、正则表达式 re
模块、字符串切片与拼接。 其中,使用 replace()
方法是最简单和直接的方式。接下来,我们将详细介绍这几种方法,并探讨它们的优缺点和适用场景。
一、使用 replace()
方法
简介
replace()
方法是 Python 中字符串对象的一个方法,用于替换字符串中的某个子字符串。它的语法非常简单:str.replace(old, new[, count])
,其中 old
是要替换的子字符串,new
是替换后的子字符串,count
是可选参数,表示替换的次数。
示例代码
original_string = "Hello world! Welcome to the world of Python."
new_string = original_string.replace("world", "universe")
print(new_string)
详细描述
优点:
- 简洁易用:
replace()
方法的语法非常简单,适合新手使用。 - 高效:对于简单的替换操作,
replace()
方法执行效率较高。
缺点:
- 不支持复杂匹配:
replace()
方法仅支持简单的字符串替换,不支持正则表达式等复杂匹配。 - 全局替换:默认情况下,
replace()
方法会替换所有匹配的子字符串。如果只想替换第一次出现的子字符串,需要手动指定count
参数。
二、使用正则表达式 re
模块
简介
Python 的 re
模块提供了对正则表达式的支持,可以用于复杂的字符串匹配和替换操作。re.sub(pattern, repl, string, count=0, flags=0)
是 re
模块中用于替换的函数。
示例代码
import re
original_string = "Hello world! Welcome to the world of Python."
new_string = re.sub(r'\bworld\b', 'universe', original_string)
print(new_string)
详细描述
优点:
- 支持复杂匹配:
re
模块支持正则表达式,可以进行复杂的字符串替换操作。 - 灵活性高:可以使用正则表达式的各种特性,进行灵活的匹配和替换。
缺点:
- 语法复杂:正则表达式的语法相对复杂,不适合新手使用。
- 性能较低:对于简单的替换操作,使用
re
模块的性能不如replace()
方法。
三、使用字符串切片与拼接
简介
字符串切片与拼接是一种较为灵活的替换方法,通过手动分割字符串并进行拼接来实现替换操作。
示例代码
original_string = "Hello world! Welcome to the world of Python."
target = "world"
replacement = "universe"
手动切片与拼接
start = original_string.find(target)
end = start + len(target)
new_string = original_string[:start] + replacement + original_string[end:]
print(new_string)
详细描述
优点:
- 灵活性高:可以手动控制替换的细节,适合一些特殊场景。
- 不依赖额外模块:不需要引入额外的模块,纯粹使用字符串操作。
缺点:
- 实现复杂:代码实现较为复杂,需要手动处理字符串的分割与拼接。
- 不易维护:代码可读性和维护性较差,不推荐在复杂场景下使用。
四、使用 string
模块的模板字符串
简介
Python 的 string
模块提供了模板字符串功能,可以通过模板字符串进行替换操作。
示例代码
from string import Template
original_string = "Hello $place! Welcome to the $place of Python."
template = Template(original_string)
new_string = template.substitute(place="world")
print(new_string)
详细描述
优点:
- 易于理解:模板字符串的语法简单易懂,适合新手使用。
- 灵活性适中:可以通过模板变量进行灵活替换。
缺点:
- 功能有限:模板字符串的功能相对有限,不支持复杂的匹配和替换操作。
- 性能较低:模板字符串的性能不如
replace()
方法。
五、综合对比与建议
综合对比
- 简单替换:对于简单的字符串替换操作,推荐使用
replace()
方法,它的语法简单,性能较高。 - 复杂匹配:对于需要复杂匹配的替换操作,推荐使用
re
模块的sub()
函数,它支持正则表达式,可以进行灵活的匹配和替换。 - 特殊场景:对于一些特殊的替换需求,可以考虑使用字符串切片与拼接的方法,虽然实现复杂,但灵活性最高。
- 模板替换:对于需要使用模板字符串进行替换的场景,可以使用
string
模块的模板字符串功能,虽然功能有限,但易于理解和使用。
建议
- 新手推荐:对于新手,推荐使用
replace()
方法和string
模块的模板字符串功能,它们的语法简单,易于理解和使用。 - 复杂需求:对于有复杂需求的替换操作,可以深入学习正则表达式,并使用
re
模块进行替换操作。 - 性能考虑:在性能要求较高的场景下,尽量使用
replace()
方法,它的性能相对较高。
六、代码示例与实战
简单替换示例
original_string = "Hello world! Welcome to the world of Python."
new_string = original_string.replace("world", "universe")
print(new_string)
正则表达式替换示例
import re
original_string = "Hello world! Welcome to the world of Python."
new_string = re.sub(r'\bworld\b', 'universe', original_string)
print(new_string)
字符串切片与拼接示例
original_string = "Hello world! Welcome to the world of Python."
target = "world"
replacement = "universe"
手动切片与拼接
start = original_string.find(target)
end = start + len(target)
new_string = original_string[:start] + replacement + original_string[end:]
print(new_string)
模板字符串替换示例
from string import Template
original_string = "Hello $place! Welcome to the $place of Python."
template = Template(original_string)
new_string = template.substitute(place="world")
print(new_string)
七、总结
通过本文的介绍,我们详细探讨了 Python 中替换字符串某个单词的几种方法,包括 replace()
方法、正则表达式 re
模块、字符串切片与拼接以及 string
模块的模板字符串功能。每种方法都有其优缺点和适用场景,在实际应用中,可以根据具体需求选择合适的方法。
希望这篇文章能帮助你更好地理解和使用 Python 进行字符串替换操作,并在实际项目中灵活运用这些技巧。
相关问答FAQs:
如何在Python中替换字符串中的单词?
在Python中,可以使用str.replace()
方法来替换字符串中的某个单词。这个方法接受两个参数,第一个是要替换的单词,第二个是用来替换的新单词。例如,my_string.replace("old_word", "new_word")
会将字符串中的"old_word"替换为"new_word"。这个方法是大小写敏感的,因此请确保输入的单词完全匹配。
可以使用正则表达式来替换字符串中的单词吗?
是的,Python的re
模块提供了强大的正则表达式功能,可以用来替换字符串中的单词。使用re.sub()
方法可以实现更复杂的替换需求。例如,re.sub(r'\bold_word\b', 'new_word', my_string)
可以替换整个单词,而不是部分匹配。这里的\b
表示单词边界,确保只替换完整的单词。
替换字符串中的单词时,是否可以指定替换次数?
在Python的str.replace()
方法中,可以通过第三个参数来指定替换的次数。例如,my_string.replace("old_word", "new_word", 1)
将只替换第一次出现的"old_word"。同样地,在使用re.sub()
时,也可以通过count
参数来限制替换次数,例如re.sub(r'old_word', 'new_word', my_string, count=1)
。这样可以灵活地控制替换的行为。
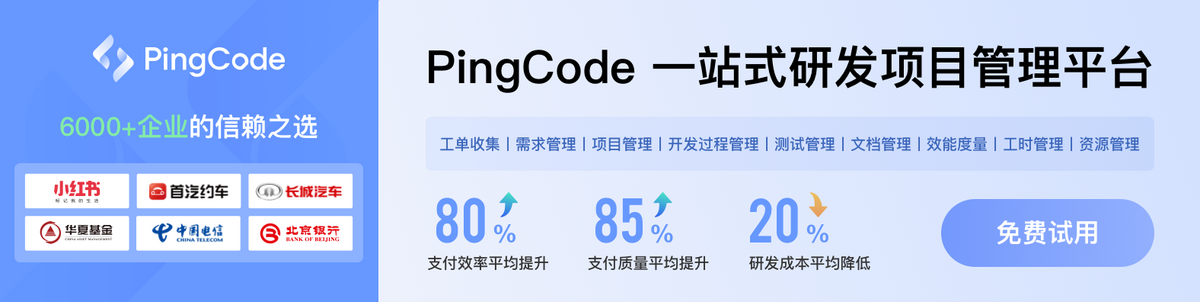