Python去掉字符串中的指定串的方法有多种,使用replace()方法、正则表达式、切片操作、字符串翻译表等。其中,replace()方法最为常用。在详细介绍其中一种方法之前,我们先来概述一下这些方法。
使用replace()方法
replace()
是字符串对象的一个方法,它可以用来将指定的子字符串替换为另一个子字符串。replace() 方法的优势在于简单、易用,适合处理较为简单的字符串替换任务。
使用正则表达式
正则表达式是一个强大的工具,可以用于复杂的模式匹配和替换任务。Python提供了 re
模块,可以使用 re.sub()
函数来替换字符串中的指定子串。
使用切片操作
切片操作可以用来手动截取字符串的某一部分,然后将其拼接起来,形成一个新的字符串。这种方法适合处理一些非常特定的情况。
使用字符串翻译表
Python的 str
类提供了 translate()
方法和 str.maketrans()
方法,可以用来构建字符串翻译表,从而实现字符串的批量替换和删除。
接下来,我们将详细介绍如何使用 replace()
方法来去掉字符串中的指定串。
一、使用replace()方法
基本用法
replace(old, new[, count])
是字符串对象的一个方法,它接受三个参数:
old
:需要被替换的子字符串。new
:用来替换old
的新子字符串。count
(可选):替换的次数。如果不指定,默认替换所有匹配的子字符串。
# 示例代码
original_string = "Hello, World! World is beautiful."
substring_to_remove = "World"
new_string = original_string.replace(substring_to_remove, "")
print(new_string) # 输出:Hello, ! is beautiful.
在这个例子中,我们使用 replace()
方法将字符串中的 "World" 替换为空字符串,从而实现删除指定子串的功能。
部分替换
如果我们只想替换字符串中的前几个匹配项,可以使用 count
参数。
# 示例代码
original_string = "Hello, World! World is beautiful. World is big."
substring_to_remove = "World"
new_string = original_string.replace(substring_to_remove, "", 1)
print(new_string) # 输出:Hello, ! World is beautiful. World is big.
在这个例子中,我们仅替换了第一个出现的 "World",后面的两个 "World" 保持不变。
二、使用正则表达式
基本用法
Python提供了 re
模块,可以使用 re.sub()
函数来替换字符串中的指定子串。
import re
示例代码
original_string = "Hello, World! World is beautiful."
substring_to_remove = "World"
new_string = re.sub(substring_to_remove, "", original_string)
print(new_string) # 输出:Hello, ! is beautiful.
在这个例子中,我们使用正则表达式将字符串中的 "World" 替换为空字符串。
高级用法
正则表达式的优势在于其强大的模式匹配能力,我们可以使用正则表达式来替换更加复杂的模式。
import re
示例代码
original_string = "Hello, World! World is beautiful."
pattern = r'\bWorld\b'
new_string = re.sub(pattern, "", original_string)
print(new_string) # 输出:Hello, ! is beautiful.
在这个例子中,我们使用了 \b
来表示单词边界,从而确保只替换完整的单词 "World"。
三、使用切片操作
基本用法
我们可以使用字符串的切片操作来手动截取字符串的一部分,然后将其拼接起来,形成一个新的字符串。
# 示例代码
original_string = "Hello, World! World is beautiful."
substring_to_remove = "World"
start_index = original_string.find(substring_to_remove)
end_index = start_index + len(substring_to_remove)
new_string = original_string[:start_index] + original_string[end_index:]
print(new_string) # 输出:Hello, ! is beautiful.
在这个例子中,我们首先找到需要删除的子串的起始位置,然后使用切片操作将其移除。
处理多个匹配项
如果字符串中有多个需要删除的子串,我们可以使用循环来处理。
# 示例代码
original_string = "Hello, World! World is beautiful."
substring_to_remove = "World"
while substring_to_remove in original_string:
start_index = original_string.find(substring_to_remove)
end_index = start_index + len(substring_to_remove)
original_string = original_string[:start_index] + original_string[end_index:]
print(original_string) # 输出:Hello, ! is beautiful.
在这个例子中,我们使用 while
循环来确保所有的匹配项都被删除。
四、使用字符串翻译表
基本用法
Python的 str
类提供了 translate()
方法和 str.maketrans()
方法,可以用来构建字符串翻译表,从而实现字符串的批量替换和删除。
# 示例代码
original_string = "Hello, World! World is beautiful."
substring_to_remove = "World"
translation_table = str.maketrans("", "", substring_to_remove)
new_string = original_string.translate(translation_table)
print(new_string) # 输出:Hello, ! is beautiful.
在这个例子中,我们使用 str.maketrans()
方法创建了一个翻译表,然后使用 translate()
方法将字符串中的 "World" 删除。
复杂翻译表
我们还可以使用翻译表来实现更加复杂的替换和删除任务。
# 示例代码
original_string = "Hello, World! World is beautiful."
translation_table = str.maketrans({
'H': 'h',
'W': 'w',
'd': ''
})
new_string = original_string.translate(translation_table)
print(new_string) # 输出:hello, world! worl is beautiful.
在这个例子中,我们使用翻译表将 "H" 替换为 "h",将 "W" 替换为 "w",并删除了所有的 "d"。
综上所述,Python提供了多种方法来去掉字符串中的指定串,包括 replace()方法、正则表达式、切片操作、字符串翻译表 等。每种方法都有其优缺点,选择哪种方法取决于具体的应用场景和需求。在实际开发中,replace()方法 和 正则表达式 是最常用的两种方法,适合处理大多数字符串替换任务。
相关问答FAQs:
如何在Python中删除字符串内的特定子串?
在Python中,可以使用str.replace()
方法来删除指定的子串。只需将要删除的子串作为第一个参数传入,并将第二个参数设置为空字符串。例如,my_string.replace("要删除的子串", "")
可以完成这一操作。
使用正则表达式能否去除字符串中的指定串?
绝对可以!通过re
模块中的re.sub()
函数,你可以使用正则表达式删除复杂模式的子串。示例代码如下:import re; re.sub("要删除的模式", "", my_string)
,这样可以灵活地处理各种字符串情况。
在处理字符串时,如何确保不影响其他部分?
为了确保只删除特定的子串而不影响其他部分,建议使用更精确的匹配模式。例如,利用正则表达式的边界匹配符,可以确保只有独立的子串被删除,而不会影响包含该子串的其他单词或字符。
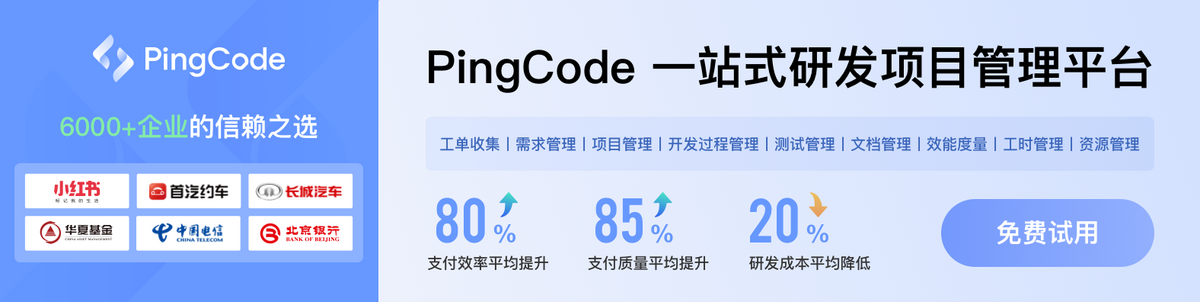