如何用Python爬取网页数据库
要用Python爬取网页数据库,可以采取以下步骤:选择合适的库(如BeautifulSoup、Scrapy)、解析网页内容、处理反爬虫机制、存储数据。其中,选择合适的库是关键,因为不同的库有不同的优势和应用场景。以BeautifulSoup为例,它是一个简单易用的库,可以轻松解析HTML文档,并提取所需的数据。接下来,将详细介绍如何使用BeautifulSoup进行网页爬取。
一、选择合适的库
BeautifulSoup
BeautifulSoup是一个Python库,专门用于从HTML和XML文件中提取数据。它能够处理多种编码格式,并且支持与多种解析器(如lxml和html.parser)配合使用。
Scrapy
Scrapy是一个强大的爬虫框架,适用于需要爬取大量数据的项目。它不仅支持高效的数据提取,还自带许多处理反爬虫机制的功能,如自动处理Cookies和用户代理。
二、解析网页内容
获取网页源代码
首先,需要使用requests库获取网页的源代码。requests是一个简单易用的HTTP库,能够轻松发送HTTP请求并获取响应。
import requests
url = 'https://example.com'
response = requests.get(url)
html_content = response.text
使用BeautifulSoup解析HTML
接下来,使用BeautifulSoup解析获取到的HTML内容。首先需要创建一个BeautifulSoup对象,然后可以根据需要提取特定的HTML标签和内容。
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
提取数据
可以使用BeautifulSoup提供的各种方法提取所需的数据。例如,使用find_all
方法查找所有的特定标签,或者使用select
方法进行CSS选择。
# 查找所有的链接
links = soup.find_all('a')
for link in links:
print(link.get('href'))
使用CSS选择器查找特定元素
items = soup.select('.item')
for item in items:
print(item.text)
三、处理反爬虫机制
设置请求头
为了避免被网站识别为爬虫,可以设置请求头,模拟正常的浏览器请求。
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
使用代理
有些网站会通过IP地址限制访问频率,可以使用代理池来绕过这一限制。
proxies = {
'http': 'http://your_proxy',
'https': 'https://your_proxy'
}
response = requests.get(url, headers=headers, proxies=proxies)
四、存储数据
存储到CSV文件
可以使用Python的csv库将提取到的数据存储到CSV文件中。
import csv
with open('data.csv', 'w', newline='') as csvfile:
fieldnames = ['name', 'price']
writer = csv.DictWriter(csvfile, fieldnames=fieldnames)
writer.writeheader()
for item in items:
writer.writerow({'name': item['name'], 'price': item['price']})
存储到数据库
如果需要存储大量数据,可以使用数据库,如MySQL或MongoDB。首先需要安装相应的库,然后使用这些库提供的方法进行数据插入。
import pymysql
connection = pymysql.connect(host='localhost',
user='user',
password='passwd',
db='database')
try:
with connection.cursor() as cursor:
sql = "INSERT INTO `items` (`name`, `price`) VALUES (%s, %s)"
for item in items:
cursor.execute(sql, (item['name'], item['price']))
connection.commit()
finally:
connection.close()
五、处理动态网页
有些网页的内容是通过JavaScript动态加载的,可以使用Selenium或Playwright等工具进行处理。
使用Selenium
Selenium是一个自动化测试工具,可以模拟浏览器操作,适用于处理动态网页。
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('https://example.com')
等待页面加载完成
driver.implicitly_wait(10)
html_content = driver.page_source
soup = BeautifulSoup(html_content, 'html.parser')
driver.quit()
使用Playwright
Playwright是一个现代化的Web自动化库,支持多种浏览器和平台。
from playwright.sync_api import sync_playwright
with sync_playwright() as p:
browser = p.chromium.launch()
page = browser.new_page()
page.goto('https://example.com')
# 获取页面内容
html_content = page.content()
soup = BeautifulSoup(html_content, 'html.parser')
browser.close()
六、处理分页数据
有些网站的数据是分页显示的,需要循环遍历每一页,提取数据。
url_template = 'https://example.com/page/{}'
page_num = 1
while True:
url = url_template.format(page_num)
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
# 提取数据
items = soup.select('.item')
if not items:
break
for item in items:
print(item.text)
page_num += 1
七、处理数据清洗和存储
数据清洗
在存储数据之前,可能需要对数据进行清洗和处理。可以使用pandas库进行数据清洗和处理。
import pandas as pd
data = pd.DataFrame(items)
data['price'] = data['price'].str.replace('$', '').astype(float)
存储到数据库
清洗后的数据可以存储到数据库中。
import sqlalchemy
engine = sqlalchemy.create_engine('mysql+pymysql://user:passwd@localhost/database')
data.to_sql('items', engine, if_exists='append', index=False)
八、定时任务
如果需要定期爬取数据,可以使用定时任务工具,如cron或APScheduler。
使用APScheduler
APScheduler是一个轻量级的Python库,可以方便地调度定时任务。
from apscheduler.schedulers.blocking import BlockingScheduler
import datetime
def job():
print("Job executed at", datetime.datetime.now())
scheduler = BlockingScheduler()
scheduler.add_job(job, 'interval', hours=1)
scheduler.start()
九、处理异常
在实际操作中,可能会遇到各种异常,需要进行异常处理,确保程序的稳定运行。
try:
response = requests.get(url, headers=headers)
response.raise_for_status()
except requests.exceptions.RequestException as e:
print(f"Error: {e}")
通过以上步骤,可以实现一个完整的网页爬取流程,从选择合适的库、解析网页内容、处理反爬虫机制、存储数据,到处理动态网页、分页数据、数据清洗和存储,以及定时任务和异常处理。希望这些内容对你有所帮助。
相关问答FAQs:
如何选择合适的Python库进行网页爬取?
在使用Python进行网页爬取时,常用的库包括Beautiful Soup、Scrapy和Requests。Beautiful Soup适合处理HTML和XML文档,能够轻松解析和提取数据;Scrapy是一个功能强大的框架,适合大型项目,支持异步下载和数据存储;Requests库则用于发送HTTP请求,简单易用,适合初学者。根据项目的复杂性和需求,选择合适的库可以大大提高爬取效率。
在爬取网页时,如何处理网站的反爬措施?
许多网站会采取反爬措施来保护数据,常见的方法包括使用CAPTCHA、IP封锁和请求频率限制。为了应对这些挑战,可以使用代理IP服务来隐藏真实IP,设置请求头模拟浏览器访问,控制请求频率以避免触发安全机制。此外,考虑使用爬虫框架中的中间件功能,自动处理这些反爬措施。
爬取数据后,如何有效存储和管理这些信息?
爬取到的数据需要有效存储和管理,以便后续使用。可以选择将数据存储在CSV文件、数据库(如MySQL或MongoDB)或者使用云存储服务。使用数据库不仅可以更好地管理和查询数据,还能够处理大量数据的存储需求。根据数据特性和使用场景,选择合适的存储方式可以提高数据的可访问性和管理效率。
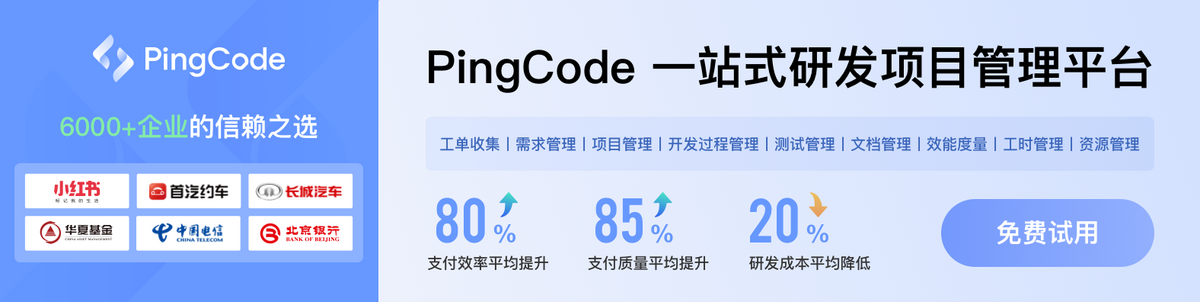