Python饼图显示百分比可以通过使用Matplotlib库来实现,具体方法包括设置autopct参数、调整字体和颜色、添加图例。其中,设置autopct参数是最核心的步骤,因为它直接负责在饼图上显示百分比。以下是详细的步骤和专业见解。
一、设置autopct参数
要在饼图上显示百分比,我们需要利用Matplotlib库中的autopct参数。这个参数可以接受一个格式字符串或一个函数,用来控制百分比的显示格式。通常使用格式字符串,如'%1.1f%%',代表保留一位小数并加上百分号。
import matplotlib.pyplot as plt
labels = ['A', 'B', 'C', 'D']
sizes = [15, 30, 45, 10]
colors = ['gold', 'yellowgreen', 'lightcoral', 'lightskyblue']
explode = (0.1, 0, 0, 0) # 突出显示第一块
plt.pie(sizes, explode=explode, labels=labels, colors=colors, autopct='%1.1f%%', shadow=True, startangle=140)
plt.axis('equal') # 保证饼图是圆形的
plt.show()
二、调整字体和颜色
除了autopct参数,调整字体和颜色可以使饼图更加美观和专业。可以使用fontdict
参数来设定字体属性,使用colors
参数来设定每块饼的颜色。
font = {'family': 'serif',
'color': 'darkred',
'weight': 'normal',
'size': 16,
}
plt.pie(sizes, explode=explode, labels=labels, colors=colors, autopct='%1.1f%%', shadow=True, startangle=140, textprops=font)
plt.axis('equal')
plt.show()
三、添加图例
在一些复杂的饼图中,添加图例可以帮助读者更好地理解数据。Matplotlib提供了简单的方法来添加图例,例如使用plt.legend()
函数。
plt.pie(sizes, explode=explode, labels=labels, colors=colors, autopct='%1.1f%%', shadow=True, startangle=140, textprops=font)
plt.axis('equal')
plt.legend(labels, loc="best")
plt.show()
四、细化autopct显示
autopct参数也可以接受一个函数,用来提供更加灵活的显示方式。例如,可以根据条件来决定是否显示百分比。
def autopct_func(pct):
return ('%1.1f%%' % pct) if pct > 5 else ''
plt.pie(sizes, explode=explode, labels=labels, colors=colors, autopct=autopct_func, shadow=True, startangle=140, textprops=font)
plt.axis('equal')
plt.show()
五、使用外部库Seaborn和Pandas
除了Matplotlib,Seaborn和Pandas也可以用于创建饼图,并具有更高的可定制性和数据处理能力。使用Pandas的plot.pie()
方法可以直接从DataFrame生成饼图。
import pandas as pd
data = {'Category': ['A', 'B', 'C', 'D'],
'Values': [15, 30, 45, 10]}
df = pd.DataFrame(data)
df.set_index('Category', inplace=True)
df['Values'].plot.pie(autopct='%1.1f%%', colors=colors, shadow=True, startangle=140)
plt.ylabel('') # 去掉y轴标签
plt.show()
六、案例分析:市场份额分析
以市场份额分析为例,假设我们有四个竞争品牌的市场份额数据,通过饼图显示它们的百分比,可以清晰地看到各品牌的市场占有情况。
import matplotlib.pyplot as plt
brands = ['Brand A', 'Brand B', 'Brand C', 'Brand D']
market_share = [20, 35, 30, 15]
colors = ['#ff9999','#66b3ff','#99ff99','#ffcc99']
explode = (0.1, 0, 0, 0) # 突出显示第一块
plt.pie(market_share, explode=explode, labels=brands, colors=colors, autopct='%1.1f%%', shadow=True, startangle=140)
plt.axis('equal')
plt.title('Market Share Analysis')
plt.legend(brands, loc="best")
plt.show()
七、动态数据更新
在实际应用中,数据可能会动态变化。例如,实时监控系统的数据可能需要不断更新。可以使用Matplotlib的动画功能来实现动态饼图。
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
import numpy as np
fig, ax = plt.subplots()
def update(num):
ax.clear()
sizes = np.random.randint(1, 100, 4)
ax.pie(sizes, labels=labels, autopct='%1.1f%%', shadow=True, startangle=140)
ax.axis('equal')
ani = FuncAnimation(fig, update, frames=10, repeat=True)
plt.show()
八、总结
Python饼图显示百分比的关键在于设置autopct参数、调整字体和颜色、添加图例。通过这些方法,可以创建专业且美观的饼图。无论是静态分析还是动态监控,Matplotlib和其他库如Seaborn和Pandas都提供了强大的工具集,使得数据可视化变得更加简单高效。
相关问答FAQs:
如何在Python饼图中添加百分比标签?
在Python中使用Matplotlib库绘制饼图时,可以通过设置autopct
参数来显示百分比。通过传入格式化字符串,比如'%.1f%%'
,可以将百分比精确到小数点后一位。示例代码如下:
import matplotlib.pyplot as plt
sizes = [15, 30, 45, 10]
labels = ['A', 'B', 'C', 'D']
plt.pie(sizes, labels=labels, autopct='%.1f%%')
plt.axis('equal') # 使饼图为圆形
plt.show()
这个代码片段将显示一个饼图,并在每个扇区上方显示其对应的百分比。
是否可以自定义饼图中百分比的显示样式?
当然可以。使用autopct
参数时,可以传入一个函数来自定义百分比的显示格式。例如,可以根据条件来改变字体颜色或大小。代码示例:
def func(pct):
return f'{pct:.1f}%' if pct > 10 else ''
plt.pie(sizes, labels=labels, autopct=func)
此示例中,只有当百分比大于10%时,才会显示对应的百分比标签。
如何控制饼图的颜色和样式?
在绘制饼图时,可以通过colors
参数自定义每个扇区的颜色。例如:
colors = ['gold', 'lightcoral', 'lightskyblue', 'lightgreen']
plt.pie(sizes, labels=labels, colors=colors, autopct='%.1f%%')
可以根据需求指定任意颜色,以使饼图更具视觉吸引力。此外,还可以通过explode
参数来突出显示某个扇区,增强饼图的效果。
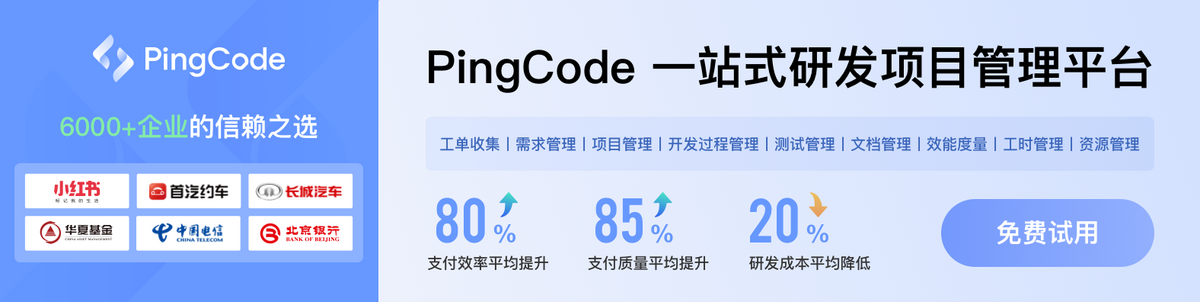