自定义类定义向量的模:使用类封装向量、实现向量的模方法、优化代码结构、考虑向量的维度。 在Python中,自定义类来定义向量的模可以通过封装向量的属性和方法来实现。具体来说,可以创建一个Vector类,包含向量的基本操作和计算模的方法。接下来,我们将详细描述如何实现这一点。
一、向量的基本概念
向量的定义与表示
向量是一种数学对象,可以在几何和物理中表示方向和大小。向量通常用有序数对或数列表示。例如,二维向量可以表示为 (x, y),三维向量可以表示为 (x, y, z)。
向量的模
向量的模(或长度)表示向量的大小。在二维空间中,向量 (x, y) 的模可以通过如下公式计算:
[ | \mathbf{v} | = \sqrt{x^2 + y^2} ]
在三维空间中,向量 (x, y, z) 的模为:
[ | \mathbf{v} | = \sqrt{x^2 + y^2 + z^2} ]
更一般地,对于 n 维向量 (x_1, x_2, …, x_n),其模为:
[ | \mathbf{v} | = \sqrt{x_1^2 + x_2^2 + … + x_n^2} ]
二、定义向量类
定义类和构造函数
首先,我们需要定义一个 Vector 类,并在构造函数中初始化向量的坐标。
import math
class Vector:
def __init__(self, *components):
self.components = components
计算向量的模
接下来,我们定义一个方法来计算向量的模。这个方法将使用前面提到的模的公式。
class Vector:
def __init__(self, *components):
self.components = components
def magnitude(self):
return math.sqrt(sum(x 2 for x in self.components))
三、添加其他常用方法
向量的加法
向量的加法是将对应的坐标相加。我们可以在 Vector 类中添加一个方法来实现这一点。
class Vector:
def __init__(self, *components):
self.components = components
def magnitude(self):
return math.sqrt(sum(x 2 for x in self.components))
def add(self, other):
if len(self.components) != len(other.components):
raise ValueError("Vectors must be of same dimensions")
added_components = [a + b for a, b in zip(self.components, other.components)]
return Vector(*added_components)
向量的减法
类似地,向量的减法是将对应的坐标相减。
class Vector:
def __init__(self, *components):
self.components = components
def magnitude(self):
return math.sqrt(sum(x 2 for x in self.components))
def add(self, other):
if len(self.components) != len(other.components):
raise ValueError("Vectors must be of same dimensions")
added_components = [a + b for a, b in zip(self.components, other.components)]
return Vector(*added_components)
def subtract(self, other):
if len(self.components) != len(other.components):
raise ValueError("Vectors must be of same dimensions")
subtracted_components = [a - b for a, b in zip(self.components, other.components)]
return Vector(*subtracted_components)
四、实现向量的点积和叉积
向量的点积
向量的点积是两个向量对应坐标的乘积之和。
class Vector:
def __init__(self, *components):
self.components = components
def magnitude(self):
return math.sqrt(sum(x 2 for x in self.components))
def add(self, other):
if len(self.components) != len(other.components):
raise ValueError("Vectors must be of same dimensions")
added_components = [a + b for a, b in zip(self.components, other.components)]
return Vector(*added_components)
def subtract(self, other):
if len(self.components) != len(other.components):
raise ValueError("Vectors must be of same dimensions")
subtracted_components = [a - b for a, b in zip(self.components, other.components)]
return Vector(*subtracted_components)
def dot(self, other):
if len(self.components) != len(other.components):
raise ValueError("Vectors must be of same dimensions")
return sum(a * b for a, b in zip(self.components, other.components))
向量的叉积
向量的叉积仅在三维空间中定义,并且结果是一个新的向量。
class Vector:
def __init__(self, *components):
self.components = components
def magnitude(self):
return math.sqrt(sum(x 2 for x in self.components))
def add(self, other):
if len(self.components) != len(other.components):
raise ValueError("Vectors must be of same dimensions")
added_components = [a + b for a, b in zip(self.components, other.components)]
return Vector(*added_components)
def subtract(self, other):
if len(self.components) != len(other.components):
raise ValueError("Vectors must be of same dimensions")
subtracted_components = [a - b for a, b in zip(self.components, other.components)]
return Vector(*subtracted_components)
def dot(self, other):
if len(self.components) != len(other.components):
raise ValueError("Vectors must be of same dimensions")
return sum(a * b for a, b in zip(self.components, other.components))
def cross(self, other):
if len(self.components) != 3 or len(other.components) != 3:
raise ValueError("Cross product is only defined for 3-dimensional vectors")
a1, a2, a3 = self.components
b1, b2, b3 = other.components
return Vector(a2 * b3 - a3 * b2, a3 * b1 - a1 * b3, a1 * b2 - a2 * b1)
五、优化代码结构
使用特殊方法增强类的表现
我们可以使用一些 Python 的特殊方法来增强 Vector 类的表现。例如,定义 __str__
方法来友好地打印向量,定义 __add__
和 __sub__
方法来使用 +
和 -
操作符进行向量加减。
class Vector:
def __init__(self, *components):
self.components = components
def magnitude(self):
return math.sqrt(sum(x 2 for x in self.components))
def add(self, other):
if len(self.components) != len(other.components):
raise ValueError("Vectors must be of same dimensions")
added_components = [a + b for a, b in zip(self.components, other.components)]
return Vector(*added_components)
def subtract(self, other):
if len(self.components) != len(other.components):
raise ValueError("Vectors must be of same dimensions")
subtracted_components = [a - b for a, b in zip(self.components, other.components)]
return Vector(*subtracted_components)
def dot(self, other):
if len(self.components) != len(other.components):
raise ValueError("Vectors must be of same dimensions")
return sum(a * b for a, b in zip(self.components, other.components))
def cross(self, other):
if len(self.components) != 3 or len(other.components) != 3:
raise ValueError("Cross product is only defined for 3-dimensional vectors")
a1, a2, a3 = self.components
b1, b2, b3 = other.components
return Vector(a2 * b3 - a3 * b2, a3 * b1 - a1 * b3, a1 * b2 - a2 * b1)
def __str__(self):
return f"Vector{self.components}"
def __add__(self, other):
return self.add(other)
def __sub__(self, other):
return self.subtract(other)
增加向量的归一化方法
归一化向量是将向量缩放到单位长度。我们可以在 Vector 类中添加一个方法来实现这一点。
class Vector:
def __init__(self, *components):
self.components = components
def magnitude(self):
return math.sqrt(sum(x 2 for x in self.components))
def add(self, other):
if len(self.components) != len(other.components):
raise ValueError("Vectors must be of same dimensions")
added_components = [a + b for a, b in zip(self.components, other.components)]
return Vector(*added_components)
def subtract(self, other):
if len(self.components) != len(other.components):
raise ValueError("Vectors must be of same dimensions")
subtracted_components = [a - b for a, b in zip(self.components, other.components)]
return Vector(*subtracted_components)
def dot(self, other):
if len(self.components) != len(other.components):
raise ValueError("Vectors must be of same dimensions")
return sum(a * b for a, b in zip(self.components, other.components))
def cross(self, other):
if len(self.components) != 3 or len(other.components) != 3:
raise ValueError("Cross product is only defined for 3-dimensional vectors")
a1, a2, a3 = self.components
b1, b2, b3 = other.components
return Vector(a2 * b3 - a3 * b2, a3 * b1 - a1 * b3, a1 * b2 - a2 * b1)
def normalize(self):
mag = self.magnitude()
if mag == 0:
raise ValueError("Cannot normalize zero vector")
normalized_components = [x / mag for x in self.components]
return Vector(*normalized_components)
def __str__(self):
return f"Vector{self.components}"
def __add__(self, other):
return self.add(other)
def __sub__(self, other):
return self.subtract(other)
六、测试和使用向量类
创建向量实例
我们可以创建向量实例并测试其方法。
v1 = Vector(3, 4)
v2 = Vector(1, 2)
print(f"v1: {v1}")
print(f"v2: {v2}")
print(f"v1 magnitude: {v1.magnitude()}")
print(f"v2 magnitude: {v2.magnitude()}")
v3 = v1 + v2
print(f"v1 + v2: {v3}")
v4 = v1 - v2
print(f"v1 - v2: {v4}")
dot_product = v1.dot(v2)
print(f"v1 · v2: {dot_product}")
v5 = v1.normalize()
print(f"Normalized v1: {v5}")
处理不同维度的向量
我们的 Vector 类已经能够处理不同维度的向量。我们只需要确保在进行操作时,向量的维度是相同的。
v3d_1 = Vector(1, 2, 3)
v3d_2 = Vector(4, 5, 6)
print(f"v3d_1: {v3d_1}")
print(f"v3d_2: {v3d_2}")
v3d_cross = v3d_1.cross(v3d_2)
print(f"v3d_1 × v3d_2: {v3d_cross}")
错误处理和边界条件
我们的 Vector 类在不同的方法中已经包含了一些基本的错误处理。例如,当向量的维度不匹配时抛出 ValueError。当尝试对零向量进行归一化时也会抛出错误。
七、总结
通过自定义 Vector 类,我们可以在 Python 中轻松地进行向量的各种操作。这个类不仅封装了向量的基本操作,还实现了向量的模、加法、减法、点积、叉积和归一化等方法。这种封装使得代码更具可读性、可维护性和可扩展性。
自定义类来定义向量的模和其他操作,使得我们可以在更复杂的数学计算和物理模拟中使用这些工具。通过这种方式,编写和维护数学计算代码变得更加简洁和高效。
相关问答FAQs:
如何在Python中自定义类来表示向量的模?
在Python中,您可以通过定义一个类来表示向量,并在类中实现一个方法来计算向量的模。通常,向量的模可以通过平方根函数和点积计算。以下是一个简单的示例:
import math
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def magnitude(self):
return math.sqrt(self.x <strong> 2 + self.y </strong> 2)
# 使用示例
v = Vector(3, 4)
print(v.magnitude()) # 输出 5.0
通过这种方式,您可以创建一个表示二维向量的类,并计算其模。
在自定义向量类中,如何支持多维向量的模计算?
要支持多维向量的模计算,您可以在类的构造函数中接受一个列表或元组作为坐标。这使得向量可以扩展到任意维度。以下是一个示例:
import math
class Vector:
def __init__(self, *components):
self.components = components
def magnitude(self):
return math.sqrt(sum(x ** 2 for x in self.components))
# 使用示例
v = Vector(1, 2, 3)
print(v.magnitude()) # 输出 3.7416573867739413
这种设计允许您创建任意维度的向量,并计算其模。
如何在自定义向量类中实现向量的加法和模的同时计算?
在向量类中,您可以实现一个加法方法,使其能够与另一个向量相加,并在加法后返回结果的模。以下是一个例子:
import math
class Vector:
def __init__(self, *components):
self.components = components
def magnitude(self):
return math.sqrt(sum(x ** 2 for x in self.components))
def add(self, other):
if len(self.components) != len(other.components):
raise ValueError("两个向量的维度必须相同")
new_components = [x + y for x, y in zip(self.components, other.components)]
return Vector(*new_components), Vector(*new_components).magnitude()
# 使用示例
v1 = Vector(1, 2)
v2 = Vector(3, 4)
result_vector, result_magnitude = v1.add(v2)
print(result_vector.components) # 输出 (4, 6)
print(result_magnitude) # 输出 7.211102550927978
通过此实现,您可以方便地进行向量的加法并同时得到结果的模。
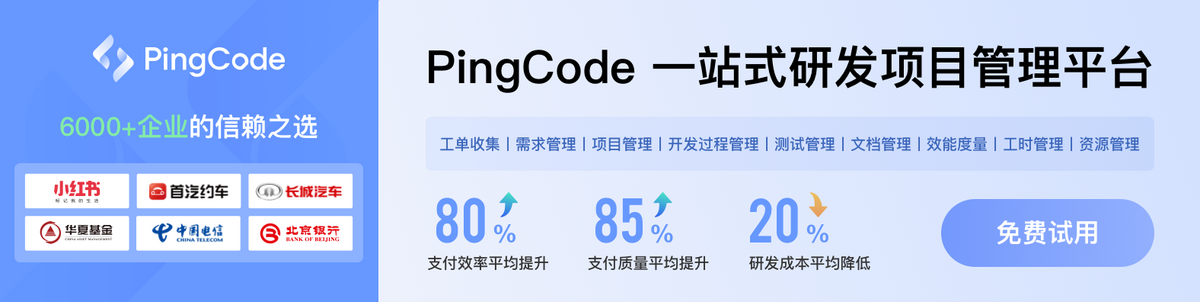