Python如何解决两个线程共享数据
在Python中,解决两个线程共享数据问题的关键在于使用线程安全的同步机制、避免竞争条件、保证数据一致性。其中,最常用的方法是利用锁(Lock)来确保只有一个线程可以访问共享资源。锁可以防止数据竞争、提高数据一致性,具体的方法包括使用标准库中的threading
模块。我们可以通过锁机制来详细解释这个过程。
使用锁(Lock)
锁(Lock)是线程同步的基本方法之一。它允许我们在访问共享资源时,只允许一个线程进行操作,从而避免数据竞争和确保数据的一致性。下面我们详细讲解锁的使用方法。
一、线程同步机制
1. 使用线程模块
Python的threading
模块提供了多种方法来实现线程的同步,其中最基本的方法是使用Lock
对象。Lock
对象有两个基本方法:acquire()
和release()
。当一个线程调用acquire()
时,如果锁已经被其他线程持有,该线程将会被阻塞,直到锁被释放。release()
方法则用于释放锁,使其他被阻塞的线程可以继续运行。
import threading
定义一个全局变量
shared_data = 0
创建锁对象
lock = threading.Lock()
def thread_task():
global shared_data
for i in range(100000):
# 获取锁
lock.acquire()
try:
# 访问和修改共享数据
shared_data += 1
finally:
# 释放锁
lock.release()
创建线程
thread1 = threading.Thread(target=thread_task)
thread2 = threading.Thread(target=thread_task)
启动线程
thread1.start()
thread2.start()
等待线程完成
thread1.join()
thread2.join()
print(shared_data)
在这个例子中,两个线程都试图增加shared_data
的值,但由于使用了锁机制,每次只有一个线程能够访问和修改shared_data
,从而避免了竞争条件。
2. 使用RLock
除了Lock
对象,Python还提供了RLock
(可重入锁),它允许同一个线程多次获取锁而不会引起死锁。RLock
在一些递归操作中非常有用。
import threading
定义一个全局变量
shared_data = 0
创建可重入锁对象
rlock = threading.RLock()
def thread_task():
global shared_data
for i in range(100000):
# 获取锁
rlock.acquire()
try:
# 访问和修改共享数据
shared_data += 1
finally:
# 释放锁
rlock.release()
创建线程
thread1 = threading.Thread(target=thread_task)
thread2 = threading.Thread(target=thread_task)
启动线程
thread1.start()
thread2.start()
等待线程完成
thread1.join()
thread2.join()
print(shared_data)
二、使用条件变量(Condition)
条件变量是另一种线程同步机制,它允许线程在满足特定条件时进行通信。条件变量通常与锁结合使用,来确保在等待条件时不会发生竞争条件。
import threading
定义一个全局变量
shared_data = 0
创建锁和条件变量对象
lock = threading.Lock()
condition = threading.Condition(lock)
def thread_task():
global shared_data
for i in range(100000):
with condition:
# 等待特定条件
while shared_data % 2 == 0:
condition.wait()
# 访问和修改共享数据
shared_data += 1
# 通知其他线程条件已满足
condition.notify_all()
def other_thread_task():
global shared_data
for i in range(100000):
with condition:
# 访问和修改共享数据
shared_data += 1
# 通知其他线程条件已满足
condition.notify_all()
创建线程
thread1 = threading.Thread(target=thread_task)
thread2 = threading.Thread(target=other_thread_task)
启动线程
thread1.start()
thread2.start()
等待线程完成
thread1.join()
thread2.join()
print(shared_data)
在这个例子中,两个线程通过条件变量进行通信,以确保在特定条件满足时进行操作。
三、使用信号量(Semaphore)
信号量是一种更高级的同步机制,它允许多个线程同时访问共享资源。信号量有一个计数器,表示可以同时访问资源的线程数量。
import threading
定义一个全局变量
shared_data = 0
创建信号量对象
semaphore = threading.Semaphore(1)
def thread_task():
global shared_data
for i in range(100000):
# 获取信号量
semaphore.acquire()
try:
# 访问和修改共享数据
shared_data += 1
finally:
# 释放信号量
semaphore.release()
创建线程
thread1 = threading.Thread(target=thread_task)
thread2 = threading.Thread(target=thread_task)
启动线程
thread1.start()
thread2.start()
等待线程完成
thread1.join()
thread2.join()
print(shared_data)
在这个例子中,信号量确保每次只有一个线程可以访问shared_data
,从而避免竞争条件。
四、使用队列(Queue)
队列是一种线程安全的数据结构,可以用于在线程之间传递数据。Python的queue
模块提供了线程安全的队列实现,包括Queue
、LifoQueue
和PriorityQueue
。
import threading
import queue
创建队列对象
data_queue = queue.Queue()
def producer():
for i in range(100000):
# 向队列中添加数据
data_queue.put(i)
def consumer():
while True:
# 从队列中获取数据
data = data_queue.get()
if data is None:
break
# 处理数据
print(data)
创建线程
producer_thread = threading.Thread(target=producer)
consumer_thread = threading.Thread(target=consumer)
启动线程
producer_thread.start()
consumer_thread.start()
等待生产者线程完成
producer_thread.join()
向队列中添加None,表示结束
data_queue.put(None)
等待消费者线程完成
consumer_thread.join()
在这个例子中,生产者线程向队列中添加数据,消费者线程从队列中获取数据并处理。队列确保了数据在多个线程之间的安全传递。
五、使用事件(Event)
事件是另一种线程同步机制,它允许一个线程等待另一个线程触发事件。事件可以用于在线程之间传递信号。
import threading
创建事件对象
event = threading.Event()
def thread_task():
print("Waiting for event...")
# 等待事件被触发
event.wait()
print("Event received!")
def other_thread_task():
# 触发事件
event.set()
创建线程
thread1 = threading.Thread(target=thread_task)
thread2 = threading.Thread(target=other_thread_task)
启动线程
thread1.start()
thread2.start()
等待线程完成
thread1.join()
thread2.join()
在这个例子中,thread_task
线程等待事件被触发,而other_thread_task
线程在准备好后触发事件,通知thread_task
线程继续运行。
六、使用线程本地数据(Thread-Local Data)
线程本地数据是一种特殊的数据存储方式,它允许每个线程拥有自己的独立数据,而不会与其他线程共享。Python的threading
模块提供了local
类来实现线程本地数据。
import threading
创建线程本地数据对象
thread_local_data = threading.local()
def thread_task():
# 设置线程本地数据
thread_local_data.value = threading.current_thread().name
print(f"Thread {thread_local_data.value} is running")
创建线程
thread1 = threading.Thread(target=thread_task, name="Thread-1")
thread2 = threading.Thread(target=thread_task, name="Thread-2")
启动线程
thread1.start()
thread2.start()
等待线程完成
thread1.join()
thread2.join()
在这个例子中,每个线程都有自己的value
属性,不会与其他线程共享,从而避免了数据竞争。
七、总结
在Python中,解决两个线程共享数据问题的关键在于使用线程安全的同步机制、避免竞争条件、保证数据一致性。我们详细介绍了使用锁(Lock)、可重入锁(RLock)、条件变量(Condition)、信号量(Semaphore)、队列(Queue)、事件(Event)和线程本地数据(Thread-Local Data)等方法来实现线程同步和数据共享。选择适当的同步机制可以有效地解决线程共享数据问题,确保数据的一致性和线程的正确运行。
相关问答FAQs:
如何在Python中安全地共享数据给多个线程?
在Python中,多个线程可以通过共享变量来交换数据。然而,为了确保数据的一致性和防止竞态条件,使用线程安全的机制是非常重要的。常用的方法包括使用threading.Lock
来创建锁,确保同一时间只有一个线程可以访问共享数据。此外,还可以使用queue.Queue
来安全地在线程之间传递数据,因为Queue
本身是线程安全的。
使用共享数据时如何避免死锁?
死锁是多线程编程中常见的问题,发生在两个或多个线程相互等待对方释放资源的情况下。为了避免死锁,可以采取一些策略,例如确保所有线程以相同的顺序请求锁、使用超时机制来放弃锁请求,以及避免在持有锁的情况下调用其他可能会引发锁的资源。
Python中哪些数据结构适合线程间共享?
在Python中,除了使用基本的数据类型(如列表和字典)外,还可以利用一些专为多线程设计的数据结构,例如queue.Queue
、collections.deque
和multiprocessing.Manager
提供的共享字典和列表。这些数据结构内部实现了必要的同步机制,可以安全地在多个线程之间共享数据,减少了手动管理锁的复杂性。
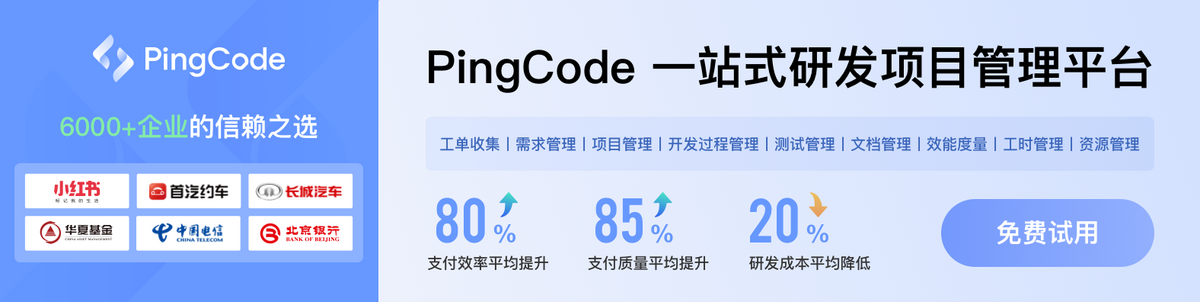