在Python中,一行输出到文件的常见方法包括使用文件操作函数如 write()
、writelines()
和 print()
函数。 最常见的方法是使用 write()
函数,因为它提供了简洁且高效的方式来将字符串写入文件。下面将详细介绍 write()
函数的使用方法。
write()
函数:这个方法通过打开文件(可以是文本文件或二进制文件),并使用 write()
方法将内容写入文件。这个方法的优点是简单易用且性能较好。
with open('example.txt', 'w') as file:
file.write('This is a single line written to the file.')
上述代码展示了如何使用 write()
函数将一行内容写入文件。接下来,我们将详细探讨Python中一行输出到文件的不同方法,并提供一些有用的技巧和注意事项。
一、文件操作基础
1、打开文件
在Python中,使用 open()
函数打开文件。open()
函数的第一个参数是文件名,第二个参数是模式(mode),常见的模式包括:
'r'
:只读模式,文件必须存在。'w'
:写入模式,文件存在则清空,不存在则创建。'a'
:追加模式,文件存在则在末尾追加,不存在则创建。
file = open('example.txt', 'w')
2、写入文件
一旦文件被打开,可以使用 write()
方法将字符串写入文件。
file.write('This is a single line written to the file.')
3、关闭文件
完成写入操作后,必须关闭文件以确保数据被正确写入文件中。
file.close()
二、使用 with
语句
1、简化文件操作
with
语句提供了一种简化文件操作的方法。它确保文件在操作完成后自动关闭,即使发生异常也会关闭。
with open('example.txt', 'w') as file:
file.write('This is a single line written to the file.')
2、避免资源泄漏
使用 with
语句可以避免资源泄漏问题,因为它自动管理文件的打开和关闭。
三、print()
函数
1、使用 file
参数
print()
函数同样可以用于写入文件,只需指定 file
参数。
with open('example.txt', 'w') as file:
print('This is a single line written to the file.', file=file)
2、自动换行
使用 print()
函数时,默认会在字符串末尾添加换行符。如果不需要换行,可以通过 end
参数来控制。
with open('example.txt', 'w') as file:
print('This is a single line written to the file.', file=file, end='')
四、writelines()
方法
1、写入多行
writelines()
方法可以写入多个字符串,但需要注意的是,它不会自动添加换行符。
lines = ['Line 1\n', 'Line 2\n', 'Line 3\n']
with open('example.txt', 'w') as file:
file.writelines(lines)
2、手动添加换行符
如果使用 writelines()
方法,必须确保每个字符串末尾都有换行符。
lines = ['Line 1', 'Line 2', 'Line 3']
with open('example.txt', 'w') as file:
file.writelines([line + '\n' for line in lines])
五、二进制文件写入
1、打开二进制文件
要写入二进制文件,需要在模式中使用 'b'
。
with open('example.bin', 'wb') as file:
file.write(b'This is a binary file.')
2、写入二进制数据
确保写入的数据是字节类型(bytes),可以使用 b''
字符串表示法。
data = b'This is a binary file.'
with open('example.bin', 'wb') as file:
file.write(data)
六、字符串格式化
1、格式化字符串
在写入文件之前,可以使用字符串格式化来生成要写入的内容。
name = 'John'
age = 30
with open('example.txt', 'w') as file:
file.write(f'Name: {name}, Age: {age}')
2、使用 str.format()
另一种常见的字符串格式化方法是使用 str.format()
方法。
name = 'John'
age = 30
with open('example.txt', 'w') as file:
file.write('Name: {}, Age: {}'.format(name, age))
七、异常处理
1、捕获异常
在文件操作中,捕获异常是一个好习惯,以便在出现错误时可以处理。
try:
with open('example.txt', 'w') as file:
file.write('This is a single line written to the file.')
except IOError as e:
print(f'An error occurred: {e}')
2、确保文件关闭
即使发生异常,也应确保文件被正确关闭。使用 with
语句可以自动处理这一点。
try:
with open('example.txt', 'w') as file:
file.write('This is a single line written to the file.')
except IOError as e:
print(f'An error occurred: {e}')
八、性能优化
1、批量写入
对于大量数据,批量写入比逐行写入效率更高。
data = ['Line {}\n'.format(i) for i in range(1000)]
with open('example.txt', 'w') as file:
file.writelines(data)
2、缓冲区管理
Python的文件操作有内部缓冲区,可以通过 buffering
参数来调整。
with open('example.txt', 'w', buffering=1) as file:
file.write('This is a single line written to the file.')
九、文本编码
1、指定编码
在处理非ASCII字符时,指定文件编码非常重要。
with open('example.txt', 'w', encoding='utf-8') as file:
file.write('这是用UTF-8编码写入的一行。')
2、读取文件编码
读取文件时,也应指定相同的编码。
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
print(content)
十、实际应用案例
1、日志文件
日志文件是文件写入的一个常见应用,可以使用追加模式来记录日志。
import datetime
def log_message(message):
timestamp = datetime.datetime.now().strftime('%Y-%m-%d %H:%M:%S')
with open('log.txt', 'a') as log_file:
log_file.write(f'[{timestamp}] {message}\n')
log_message('This is a log message.')
2、配置文件
另一个常见的应用是配置文件,可以使用简单的键值对格式来保存配置信息。
config = {'username': 'admin', 'password': 'secret'}
with open('config.txt', 'w') as config_file:
for key, value in config.items():
config_file.write(f'{key}={value}\n')
通过以上的详细介绍,相信大家对Python中如何一行输出到文件有了更深入的了解。希望这些内容能够对你的实际编程工作提供帮助。
相关问答FAQs:
如何在Python中将一行文本写入文件?
在Python中,可以使用内置的open()
函数与文件的write()
方法来实现一行文本的输出。首先,打开文件(如果文件不存在,Python会自动创建新文件),然后使用write()
方法将字符串写入文件。确保在写入后关闭文件,或者使用with
语句来自动管理文件打开和关闭。示例代码如下:
with open('output.txt', 'w') as file:
file.write('这一行将被写入文件中\n')
如何在Python中添加内容到已有文件的末尾?
如果希望将文本添加到已存在文件的末尾,而不是覆盖原有内容,可以使用open()
函数的'a'
模式。这种模式允许你在文件末尾追加新的内容,而不会影响到文件中已有的内容。例如:
with open('output.txt', 'a') as file:
file.write('这行将被追加到文件末尾\n')
在Python中如何确保写入文件时处理异常?
在进行文件操作时,可能会遇到各种异常情况,例如文件权限不足或文件路径错误。为了确保程序的健壮性,可以使用try-except
语句来捕获这些异常。这样可以在出现错误时提供友好的提示,而不是让程序直接崩溃。示例代码如下:
try:
with open('output.txt', 'w') as file:
file.write('安全写入这一行\n')
except IOError as e:
print(f'写入文件时发生错误: {e}')
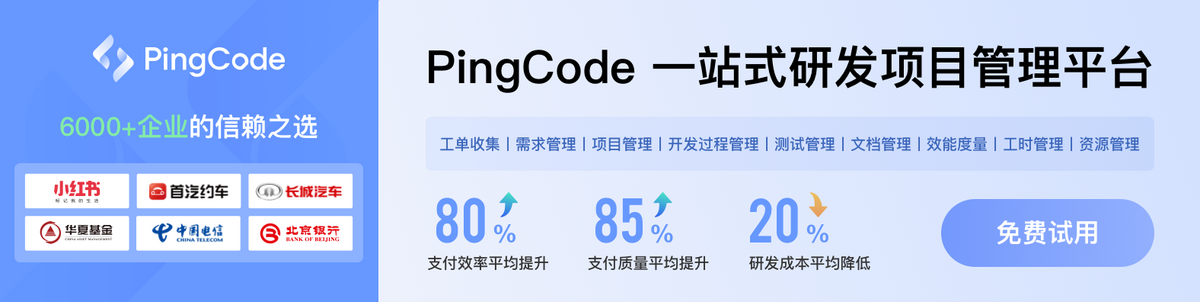