要使用Python编写一个天气预报程序,你需要掌握几个关键点:选择合适的API、安装所需的Python库、获取和解析天气数据、处理异常情况。其中,选择合适的API是最为关键的一步,因为它决定了你能够获得的数据的准确性和丰富程度。接下来,我们将详细介绍如何实现这一步骤。
在本文中,我们将详细介绍如何使用Python编写一个天气预报程序。我们将从选择合适的API入手,然后安装所需的Python库,最后编写代码来获取和解析天气数据,并处理可能的异常情况。
一、选择合适的API
选择一个合适的API是编写天气预报程序的第一步。市面上有很多免费的和付费的天气API,如OpenWeatherMap、Weatherstack、AccuWeather等。
1.1 OpenWeatherMap
OpenWeatherMap是一个非常流行的天气API,提供免费的和付费的API密钥。它的数据覆盖全球,并且有详细的文档,适合初学者使用。
- 优点:免费层次提供了足够多的功能、全球覆盖、详细的文档。
- 缺点:免费层次的调用次数有限。
1.2 Weatherstack
Weatherstack也是一个受欢迎的天气API,提供实时和历史天气数据。它的免费层次也非常适合初学者。
- 优点:实时和历史数据、易于使用。
- 缺点:免费层次的调用次数有限。
1.3 AccuWeather
AccuWeather提供高精度的天气预报数据,但其免费层次的功能相对较少,更多适用于商业用途。
- 优点:高精度数据、适用于商业用途。
- 缺点:免费层次功能有限。
二、安装所需的Python库
在选择好API之后,我们需要安装一些Python库来帮助我们进行HTTP请求和数据处理。常用的库包括requests
和json
。
2.1 安装requests库
requests
是一个非常流行的HTTP库,适用于各种HTTP请求操作。你可以使用以下命令来安装:
pip install requests
2.2 安装json库
json
是Python内置的库,无需额外安装。它用于解析JSON格式的数据,这是大多数API返回的数据格式。
import json
三、编写代码获取和解析天气数据
接下来,我们将编写代码来获取和解析天气数据。这里以OpenWeatherMap为例。
3.1 获取API密钥
首先,你需要在OpenWeatherMap网站上注册一个账号并获取API密钥。这是后续请求数据的必要条件。
3.2 编写请求函数
我们需要编写一个函数来发送HTTP请求并获取天气数据。以下是一个简单的示例:
import requests
import json
def get_weather(city, api_key):
url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}"
response = requests.get(url)
if response.status_code == 200:
return response.json()
else:
return None
3.3 解析天气数据
获取到天气数据后,我们需要解析它并提取有用的信息。以下是一个示例:
def parse_weather(data):
if data:
main = data['weather'][0]['main']
description = data['weather'][0]['description']
temp = data['main']['temp']
humidity = data['main']['humidity']
return {
'main': main,
'description': description,
'temp': temp,
'humidity': humidity
}
else:
return None
四、处理异常情况
在实际使用中,我们需要处理各种可能的异常情况,如网络错误、API限额超出等。
4.1 网络错误
网络错误是最常见的问题之一。我们可以使用try-except
块来捕获这些错误:
def get_weather(city, api_key):
try:
url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}"
response = requests.get(url)
response.raise_for_status() # 如果请求失败则引发HTTPError
return response.json()
except requests.exceptions.RequestException as e:
print(f"Error: {e}")
return None
4.2 API限额超出
大多数免费的API都有调用次数限制。我们可以通过检查API返回的状态码来处理这种情况:
def get_weather(city, api_key):
url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}"
response = requests.get(url)
if response.status_code == 200:
return response.json()
elif response.status_code == 429: # 429表示API限额超出
print("API call limit exceeded")
return None
else:
print("Failed to get data")
return None
五、完整代码示例
以下是一个完整的天气预报程序示例:
import requests
import json
def get_weather(city, api_key):
try:
url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}"
response = requests.get(url)
response.raise_for_status()
return response.json()
except requests.exceptions.RequestException as e:
print(f"Error: {e}")
return None
def parse_weather(data):
if data:
main = data['weather'][0]['main']
description = data['weather'][0]['description']
temp = data['main']['temp']
humidity = data['main']['humidity']
return {
'main': main,
'description': description,
'temp': temp,
'humidity': humidity
}
else:
return None
def main():
city = "London"
api_key = "your_api_key_here"
data = get_weather(city, api_key)
weather = parse_weather(data)
if weather:
print(f"Weather in {city}:")
print(f"Main: {weather['main']}")
print(f"Description: {weather['description']}")
print(f"Temperature: {weather['temp']}")
print(f"Humidity: {weather['humidity']}")
else:
print("Failed to get weather data")
if __name__ == "__main__":
main()
六、扩展功能
6.1 支持多个城市
你可以扩展程序以支持多个城市的天气预报。只需将城市列表传递给get_weather
函数即可。
def main():
cities = ["London", "New York", "Tokyo"]
api_key = "your_api_key_here"
for city in cities:
data = get_weather(city, api_key)
weather = parse_weather(data)
if weather:
print(f"Weather in {city}:")
print(f"Main: {weather['main']}")
print(f"Description: {weather['description']}")
print(f"Temperature: {weather['temp']}")
print(f"Humidity: {weather['humidity']}")
else:
print(f"Failed to get weather data for {city}")
if __name__ == "__main__":
main()
6.2 添加用户输入
你还可以让用户输入城市名称以获取天气预报。
def main():
api_key = "your_api_key_here"
city = input("Enter the city name: ")
data = get_weather(city, api_key)
weather = parse_weather(data)
if weather:
print(f"Weather in {city}:")
print(f"Main: {weather['main']}")
print(f"Description: {weather['description']}")
print(f"Temperature: {weather['temp']}")
print(f"Humidity: {weather['humidity']}")
else:
print("Failed to get weather data")
if __name__ == "__main__":
main()
6.3 转换温度单位
API返回的温度通常是凯氏温度。你可以将其转换为摄氏温度或华氏温度。
def kelvin_to_celsius(kelvin):
return kelvin - 273.15
def kelvin_to_fahrenheit(kelvin):
return (kelvin - 273.15) * 9/5 + 32
def parse_weather(data):
if data:
main = data['weather'][0]['main']
description = data['weather'][0]['description']
temp = data['main']['temp']
humidity = data['main']['humidity']
return {
'main': main,
'description': description,
'temp_celsius': kelvin_to_celsius(temp),
'temp_fahrenheit': kelvin_to_fahrenheit(temp),
'humidity': humidity
}
else:
return None
def main():
city = "London"
api_key = "your_api_key_here"
data = get_weather(city, api_key)
weather = parse_weather(data)
if weather:
print(f"Weather in {city}:")
print(f"Main: {weather['main']}")
print(f"Description: {weather['description']}")
print(f"Temperature: {weather['temp_celsius']} °C / {weather['temp_fahrenheit']} °F")
print(f"Humidity: {weather['humidity']}%")
else:
print("Failed to get weather data")
if __name__ == "__main__":
main()
通过以上步骤和扩展功能,你可以用Python编写一个功能丰富的天气预报程序。选择合适的API、安装所需的库、编写代码获取和解析数据以及处理异常情况是实现这一目标的关键步骤。希望这篇文章能帮助你更好地理解和实现这一过程。
相关问答FAQs:
如何选择合适的天气API来获取天气数据?
在编写天气预报程序时,选择一个可靠的天气API至关重要。常见的选择包括OpenWeatherMap、WeatherAPI和AccuWeather等。查看这些API的文档,了解它们提供的数据种类、访问限制和费用结构是关键。确保所选API能够满足你应用的需求,并注意API的调用频率限制,以避免超出免费额度。
用Python获取天气数据的基本步骤是什么?
使用Python获取天气数据的基本步骤包括:安装请求库(如requests),获取API密钥,使用API的URL构建请求,发送请求以获取天气数据,并对返回的JSON数据进行解析。解析之后,你可以提取所需的信息,如温度、湿度和天气状况,然后将其格式化并显示。
如何在天气预报程序中处理异常和错误?
在编写天气预报程序时,处理异常和错误是非常重要的。可以使用try-except语句来捕获网络请求中可能出现的错误,例如连接超时或无效的API密钥。此外,解析JSON数据时也可能会遇到格式错误,因此在提取数据之前,应该检查返回的状态码和数据结构是否符合预期。通过适当的错误处理,可以提高程序的健壮性并改善用户体验。
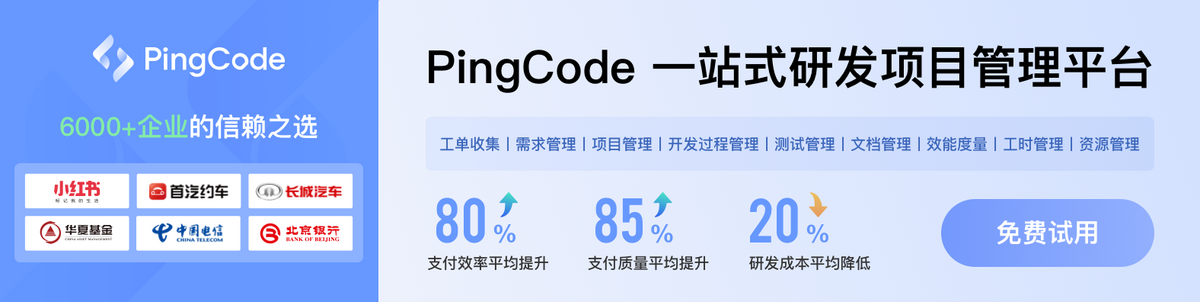