在Python中,可以通过多种方法在指定目录下创建新文件,使用open()
函数、os
模块、以及pathlib
模块。本文将主要介绍这几种方法,并详细解释如何使用它们来在指定目录下创建新文件。
一、使用open()
函数创建新文件
Python内置的open()
函数是创建和操作文件的最常用方法之一。通过指定文件路径和模式,可以方便地在指定目录下创建新文件。
1、基本用法
要在指定目录下创建新文件,可以使用如下代码:
file_path = '/path/to/directory/new_file.txt'
with open(file_path, 'w') as file:
file.write('This is a new file.')
在上述代码中,file_path
是要创建文件的完整路径,'w'
模式用于写入新文件。如果文件已存在,则会覆盖原文件内容。
2、确保目录存在
在实际应用中,目标目录可能不存在,因此需要确保目录存在。可以使用os
模块中的makedirs()
函数来创建目录:
import os
directory = '/path/to/directory'
if not os.path.exists(directory):
os.makedirs(directory)
file_path = os.path.join(directory, 'new_file.txt')
with open(file_path, 'w') as file:
file.write('This is a new file.')
上述代码首先检查目录是否存在,如果不存在则创建该目录,然后创建新文件。
二、使用os
模块创建新文件
os
模块提供了许多与操作系统交互的功能,包括创建文件和目录。
1、使用os.path
检查文件和目录
os.path
子模块提供了许多检查文件和目录的函数,例如os.path.exists()
、os.path.isdir()
等。结合这些函数,可以更灵活地创建新文件。
import os
directory = '/path/to/directory'
file_path = os.path.join(directory, 'new_file.txt')
if not os.path.exists(directory):
os.makedirs(directory)
if not os.path.exists(file_path):
with open(file_path, 'w') as file:
file.write('This is a new file.')
else:
print('File already exists.')
2、使用os.makedirs()
创建多级目录
有时候需要创建多级目录,可以使用os.makedirs()
函数:
import os
directory = '/path/to/multiple/levels/directory'
file_path = os.path.join(directory, 'new_file.txt')
os.makedirs(directory, exist_ok=True)
with open(file_path, 'w') as file:
file.write('This is a new file in a multi-level directory.')
exist_ok=True
参数确保当目录已经存在时不会引发错误。
三、使用pathlib
模块创建新文件
pathlib
模块是Python 3.4引入的,用于面向对象地处理文件和目录路径。相比os
模块,pathlib
更加直观和易用。
1、基本用法
使用pathlib
模块,可以很方便地创建新文件:
from pathlib import Path
directory = Path('/path/to/directory')
file_path = directory / 'new_file.txt'
directory.mkdir(parents=True, exist_ok=True)
with file_path.open('w') as file:
file.write('This is a new file.')
Path
对象提供了许多方便的方法,例如mkdir()
和open()
。
2、检查文件和目录
同样地,可以使用pathlib
模块中的方法来检查文件和目录:
from pathlib import Path
directory = Path('/path/to/directory')
file_path = directory / 'new_file.txt'
if not directory.exists():
directory.mkdir(parents=True)
if not file_path.exists():
with file_path.open('w') as file:
file.write('This is a new file.')
else:
print('File already exists.')
四、使用异常处理确保安全
无论使用哪种方法创建新文件,都应该考虑异常处理,以确保程序的健壮性。
1、捕获文件操作异常
在文件操作中,可能会遇到各种异常,例如权限错误、磁盘空间不足等。因此,使用try...except
块来捕获并处理这些异常是很重要的:
import os
directory = '/path/to/directory'
file_path = os.path.join(directory, 'new_file.txt')
try:
if not os.path.exists(directory):
os.makedirs(directory)
with open(file_path, 'w') as file:
file.write('This is a new file.')
except OSError as e:
print(f'Error: {e}')
2、确保文件资源释放
使用with
语句可以确保文件操作完成后,文件资源被正确释放,即使在发生异常时也是如此:
from pathlib import Path
directory = Path('/path/to/directory')
file_path = directory / 'new_file.txt'
try:
directory.mkdir(parents=True, exist_ok=True)
with file_path.open('w') as file:
file.write('This is a new file.')
except OSError as e:
print(f'Error: {e}')
五、总结
在Python中,通过多种方法可以在指定目录下创建新文件,主要包括使用open()
函数、os
模块和pathlib
模块。这些方法各有优缺点,选择适合的工具可以提高代码的可读性和可维护性。
- 使用
open()
函数:适合简单的文件操作,尤其是对于已有的代码库。 - 使用
os
模块:提供了丰富的文件和目录操作函数,适合复杂的文件系统操作。 - 使用
pathlib
模块:面向对象的路径操作,更加直观和易用,推荐在新的项目中使用。
无论选择哪种方法,都应该注意确保目录存在、处理文件操作异常,以及确保文件资源被正确释放。通过这些方法,您可以在Python中轻松创建和操作文件,提高开发效率。
相关问答FAQs:
在Python中,可以使用哪些方法在指定目录下创建新文件?
在Python中,创建新文件有多种方法。常用的方法包括使用内置的open()
函数和pathlib
模块。使用open()
函数时,可以指定文件的路径和模式,例如写入模式('w'),这将创建一个新文件或覆盖现有文件。而使用pathlib.Path
对象的touch()
方法,能够以更简洁的方式创建文件。在创建文件之前,确保指定的目录存在,否则将会抛出错误。
如果指定的目录不存在,如何在Python中创建目录并文件?
可以使用os
模块中的makedirs()
函数来创建指定目录。使用此函数时,可以设置exist_ok=True
参数,以防止因目录已存在而引发异常。在目录创建后,可以使用open()
函数或pathlib
模块创建文件。例如,创建目录后,可以直接创建文件,确保目录结构完整,避免路径错误。
在创建新文件时,有哪些常见的错误需要注意?
在创建新文件时,可能会遇到一些常见错误,例如权限不足、指定路径不正确或目录不存在等。确保在运行代码之前,拥有对目标目录的写入权限。此外,使用绝对路径可以减少因路径错误导致的问题。如果出现异常,可以使用try
和except
语句来捕获并处理这些错误,以提高代码的健壮性。
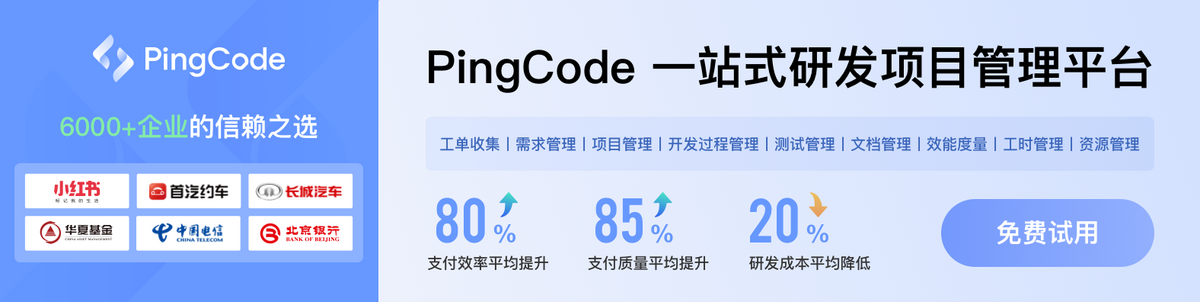