在Python中,统计字符串中的数字个数可以通过多种方法实现:使用循环、正则表达式、内置函数等。在这篇文章中,我们将详细探讨这些方法,并提供示例代码来帮助你更好地理解和应用这些技术。我们将重点介绍如何使用循环和正则表达式来实现这个任务。
一、使用循环统计字符串中的数字个数
使用循环来统计字符串中的数字个数是一种简单且直观的方法。通过遍历字符串中的每一个字符,我们可以检查每一个字符是否为数字,并在发现数字时增加计数器。
1、遍历字符串
首先,我们可以通过遍历字符串中的每一个字符来统计数字个数。以下是一个简单的示例:
def count_digits_in_string(s):
count = 0
for char in s:
if char.isdigit():
count += 1
return count
示例用法
string = "Hello123World456"
print(f"字符串中的数字个数是: {count_digits_in_string(string)}")
在这个例子中,我们定义了一个函数 count_digits_in_string
,它接受一个字符串 s
作为参数,并返回字符串中数字的个数。我们使用 char.isdigit()
方法来检查每一个字符是否为数字。
2、使用列表推导式
列表推导式是一种简洁的方式来实现循环操作。我们可以使用列表推导式来统计字符串中的数字个数:
def count_digits_in_string(s):
return sum([1 for char in s if char.isdigit()])
示例用法
string = "Hello123World456"
print(f"字符串中的数字个数是: {count_digits_in_string(string)}")
在这个例子中,我们使用列表推导式创建了一个包含所有数字的列表,并使用 sum()
函数计算列表中元素的总数。
二、使用正则表达式统计字符串中的数字个数
正则表达式是一种强大的字符串匹配工具,可以用来查找字符串中的特定模式。我们可以使用正则表达式来查找字符串中的所有数字,并计算它们的个数。
1、使用 re.findall()
方法
Python 的 re
模块提供了 findall()
方法,可以用来查找所有匹配的模式。我们可以使用这个方法来查找字符串中的所有数字:
import re
def count_digits_in_string(s):
return len(re.findall(r'\d', s))
示例用法
string = "Hello123World456"
print(f"字符串中的数字个数是: {count_digits_in_string(string)}")
在这个例子中,我们使用正则表达式模式 \d
来匹配所有数字,并使用 re.findall()
方法查找所有匹配的数字。最后,我们返回匹配数字的总数。
2、使用 re.finditer()
方法
re.finditer()
方法返回一个迭代器,可以逐个访问匹配的对象。我们可以使用这个方法来统计字符串中的数字个数:
import re
def count_digits_in_string(s):
return sum(1 for _ in re.finditer(r'\d', s))
示例用法
string = "Hello123World456"
print(f"字符串中的数字个数是: {count_digits_in_string(string)}")
在这个例子中,我们使用 re.finditer()
方法查找所有匹配的数字,并使用 sum()
函数计算总数。
三、使用内置函数统计字符串中的数字个数
Python 提供了一些内置函数,可以简化字符串操作。我们可以使用这些内置函数来统计字符串中的数字个数。
1、使用 filter()
函数
filter()
函数可以用来过滤符合特定条件的元素。我们可以使用 filter()
函数来过滤字符串中的数字,并计算它们的个数:
def count_digits_in_string(s):
return len(list(filter(str.isdigit, s)))
示例用法
string = "Hello123World456"
print(f"字符串中的数字个数是: {count_digits_in_string(string)}")
在这个例子中,我们使用 filter()
函数过滤出所有数字,并使用 len()
函数计算数字的个数。
2、使用 collections.Counter
类
collections.Counter
类是一个计数器,可以用来统计元素的出现次数。我们可以使用 Counter
类来统计字符串中的数字个数:
from collections import Counter
def count_digits_in_string(s):
return sum(c.isdigit() for c in Counter(s))
示例用法
string = "Hello123World456"
print(f"字符串中的数字个数是: {count_digits_in_string(string)}")
在这个例子中,我们使用 Counter
类统计每个字符的出现次数,并使用 sum()
函数计算数字的个数。
四、综合示例
为了更好地理解这些方法,我们可以将它们结合起来,并创建一个综合示例,展示如何使用不同的方法统计字符串中的数字个数:
import re
from collections import Counter
def count_digits_with_loop(s):
count = 0
for char in s:
if char.isdigit():
count += 1
return count
def count_digits_with_list_comprehension(s):
return sum([1 for char in s if char.isdigit()])
def count_digits_with_regex_findall(s):
return len(re.findall(r'\d', s))
def count_digits_with_regex_finditer(s):
return sum(1 for _ in re.finditer(r'\d', s))
def count_digits_with_filter(s):
return len(list(filter(str.isdigit, s)))
def count_digits_with_counter(s):
return sum(c.isdigit() for c in Counter(s))
示例用法
string = "Hello123World456"
print(f"使用循环统计数字个数: {count_digits_with_loop(string)}")
print(f"使用列表推导式统计数字个数: {count_digits_with_list_comprehension(string)}")
print(f"使用正则表达式 findall 统计数字个数: {count_digits_with_regex_findall(string)}")
print(f"使用正则表达式 finditer 统计数字个数: {count_digits_with_regex_finditer(string)}")
print(f"使用 filter 函数统计数字个数: {count_digits_with_filter(string)}")
print(f"使用 Counter 类统计数字个数: {count_digits_with_counter(string)}")
在这个综合示例中,我们定义了多个函数,每个函数使用不同的方法来统计字符串中的数字个数。我们可以看到,不同的方法都能有效地统计字符串中的数字个数。
五、性能比较
不同的方法在性能上可能会有所差异。我们可以使用 timeit
模块来比较不同方法的性能,以确定哪种方法在处理大字符串时表现更好。
import timeit
定义一个大字符串
large_string = "Hello123World456" * 10000
定义测试函数
def test_count_digits_with_loop():
count_digits_with_loop(large_string)
def test_count_digits_with_list_comprehension():
count_digits_with_list_comprehension(large_string)
def test_count_digits_with_regex_findall():
count_digits_with_regex_findall(large_string)
def test_count_digits_with_regex_finditer():
count_digits_with_regex_finditer(large_string)
def test_count_digits_with_filter():
count_digits_with_filter(large_string)
def test_count_digits_with_counter():
count_digits_with_counter(large_string)
测试每个方法的执行时间
print(f"使用循环的执行时间: {timeit.timeit(test_count_digits_with_loop, number=100)}")
print(f"使用列表推导式的执行时间: {timeit.timeit(test_count_digits_with_list_comprehension, number=100)}")
print(f"使用正则表达式 findall 的执行时间: {timeit.timeit(test_count_digits_with_regex_findall, number=100)}")
print(f"使用正则表达式 finditer 的执行时间: {timeit.timeit(test_count_digits_with_regex_finditer, number=100)}")
print(f"使用 filter 函数的执行时间: {timeit.timeit(test_count_digits_with_filter, number=100)}")
print(f"使用 Counter 类的执行时间: {timeit.timeit(test_count_digits_with_counter, number=100)}")
在这个例子中,我们使用 timeit.timeit()
方法来测量每个方法的执行时间。我们可以通过比较这些执行时间来确定哪种方法在处理大字符串时表现最佳。
总结
在本文中,我们详细探讨了如何在Python中统计字符串中的数字个数,介绍了使用循环、正则表达式和内置函数等多种方法。每种方法都有其优点和适用场景,选择哪种方法取决于具体需求和性能考虑。
使用循环和列表推导式适合初学者和简单场景,正则表达式提供了更强大的模式匹配能力,内置函数则提供了简洁的解决方案。在实际应用中,我们可以根据字符串的大小和复杂度来选择最合适的方法。
无论你选择哪种方法,掌握这些技术将帮助你更高效地处理字符串数据,提高Python编程技能。希望这篇文章对你有所帮助!
相关问答FAQs:
如何在Python中提取字符串中的所有数字?
在Python中,可以使用正则表达式来提取字符串中的所有数字。通过导入re
模块并使用re.findall()
方法,可以轻松地找到字符串中的数字。示例代码如下:
import re
text = "在2023年,Python编程语言有123种应用。"
numbers = re.findall(r'\d+', text)
print(numbers) # 输出:['2023', '123']
这段代码会提取出字符串中的所有数字并以列表的形式返回。
Python中如何计算字符串中所有数字的总和?
要计算字符串中所有数字的总和,可以先提取出数字,然后将它们转换为整数并计算总和。以下是实现的示例:
import re
text = "在2023年,Python编程语言有123种应用。"
numbers = map(int, re.findall(r'\d+', text))
total = sum(numbers)
print(total) # 输出:2146
这段代码不仅提取了数字,还将它们转换为整数并计算了总和。
如果字符串中没有数字,会导致什么结果?
当字符串中没有数字时,使用re.findall()
方法会返回一个空列表。计算总和时,sum()
函数将返回0。这可以通过以下代码验证:
import re
text = "没有任何数字的字符串。"
numbers = re.findall(r'\d+', text)
total = sum(map(int, numbers))
print(total) # 输出:0
这种情况在处理数据时要特别注意,以确保代码的健壮性和准确性。
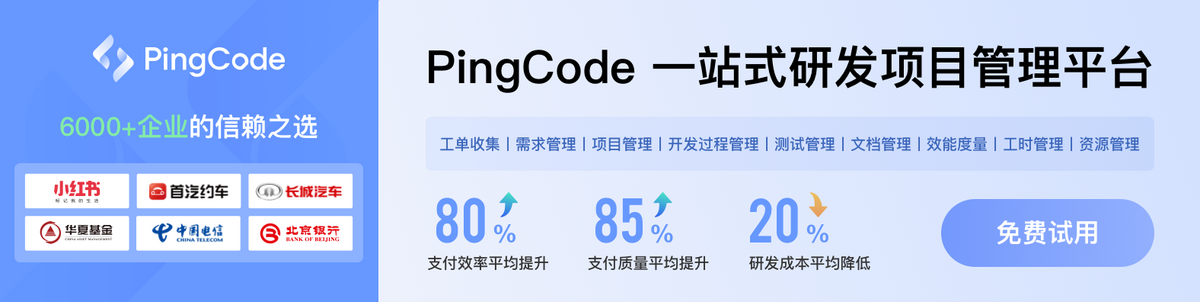