在Python中反转字符串中的单词,可以通过多种方法来实现,例如使用内置的字符串操作函数、使用列表、使用正则表达式等。 其中,最常见的方法是使用split()和join()方法。具体方法包括:使用split()和join()方法、使用列表的reverse()方法、使用re模块进行正则表达式操作。 下面我们将详细介绍其中一种方法,即使用split()和join()方法。
使用split()和join()方法是最简单和直观的方法之一。首先,通过split()方法将字符串分割成单词列表,然后使用列表的reverse()方法反转列表,最后通过join()方法将反转后的单词列表重新组合成字符串。这个方法不仅代码简洁,而且运行效率较高。
一、使用split()和join()方法反转字符串中的单词
split()和join()方法是Python中处理字符串的常用方法。split()方法用于将字符串分割成列表,而join()方法用于将列表中的元素连接成字符串。下面是具体实现步骤:
1.1 将字符串分割成单词列表
首先,我们需要将字符串按照空格分割成单词列表。可以使用split()方法完成这一操作。
input_string = "Hello world this is Python"
words_list = input_string.split()
print(words_list)
输出结果为:
['Hello', 'world', 'this', 'is', 'Python']
1.2 反转单词列表
接下来,我们需要将分割后的单词列表进行反转。可以使用列表的reverse()方法或切片操作来实现。
words_list.reverse()
print(words_list)
或者使用切片操作:
reversed_words_list = words_list[::-1]
print(reversed_words_list)
1.3 将反转后的单词列表重新组合成字符串
最后,我们使用join()方法将反转后的单词列表重新组合成字符串。
reversed_string = ' '.join(reversed_words_list)
print(reversed_string)
完整代码如下:
input_string = "Hello world this is Python"
words_list = input_string.split()
reversed_words_list = words_list[::-1]
reversed_string = ' '.join(reversed_words_list)
print(reversed_string)
输出结果为:
Python is this world Hello
二、使用列表的reverse()方法反转字符串中的单词
反转字符串中的单词还可以通过直接使用列表的reverse()方法来实现,这种方法更加简洁直观。
2.1 将字符串分割成单词列表
首先,同样需要将字符串分割成单词列表。
input_string = "Hello world this is Python"
words_list = input_string.split()
2.2 使用reverse()方法反转单词列表
然后,使用reverse()方法反转单词列表。
words_list.reverse()
2.3 将反转后的单词列表重新组合成字符串
最后,使用join()方法将反转后的单词列表重新组合成字符串。
reversed_string = ' '.join(words_list)
print(reversed_string)
完整代码如下:
input_string = "Hello world this is Python"
words_list = input_string.split()
words_list.reverse()
reversed_string = ' '.join(words_list)
print(reversed_string)
输出结果为:
Python is this world Hello
三、使用re模块进行正则表达式操作
使用正则表达式也可以实现反转字符串中的单词,尤其在处理复杂字符串时非常有用。
3.1 导入re模块
首先,需要导入re模块。
import re
3.2 使用re.split()方法分割字符串
使用re.split()方法按照空格分割字符串。
input_string = "Hello world this is Python"
words_list = re.split(r'\s+', input_string)
3.3 反转单词列表
同样,可以使用reverse()方法或切片操作反转单词列表。
words_list.reverse()
3.4 使用join()方法将反转后的单词列表重新组合成字符串
最后,使用join()方法将反转后的单词列表重新组合成字符串。
reversed_string = ' '.join(words_list)
print(reversed_string)
完整代码如下:
import re
input_string = "Hello world this is Python"
words_list = re.split(r'\s+', input_string)
words_list.reverse()
reversed_string = ' '.join(words_list)
print(reversed_string)
输出结果为:
Python is this world Hello
四、使用递归方法反转字符串中的单词
递归方法也是一种可以用来反转字符串中单词的方法。递归方法通过不断分割字符串并反转子字符串来实现反转操作。
4.1 定义递归函数
首先,需要定义一个递归函数来反转字符串中的单词。
def reverse_words_recursive(s):
words = s.split()
if len(words) <= 1:
return s
return ' '.join(words[-1:] + [reverse_words_recursive(' '.join(words[:-1]))])
4.2 调用递归函数
然后,调用递归函数来反转字符串中的单词。
input_string = "Hello world this is Python"
reversed_string = reverse_words_recursive(input_string)
print(reversed_string)
输出结果为:
Python is this world Hello
五、使用栈数据结构反转字符串中的单词
栈是一种后进先出(LIFO)的数据结构,可以用来反转字符串中的单词。
5.1 将字符串分割成单词列表
首先,需要将字符串分割成单词列表。
input_string = "Hello world this is Python"
words_list = input_string.split()
5.2 使用栈存储单词并反转
然后,使用栈将单词列表反转。
stack = []
for word in words_list:
stack.append(word)
reversed_words_list = []
while stack:
reversed_words_list.append(stack.pop())
5.3 将反转后的单词列表重新组合成字符串
最后,使用join()方法将反转后的单词列表重新组合成字符串。
reversed_string = ' '.join(reversed_words_list)
print(reversed_string)
完整代码如下:
input_string = "Hello world this is Python"
words_list = input_string.split()
stack = []
for word in words_list:
stack.append(word)
reversed_words_list = []
while stack:
reversed_words_list.append(stack.pop())
reversed_string = ' '.join(reversed_words_list)
print(reversed_string)
输出结果为:
Python is this world Hello
六、使用双端队列(deque)反转字符串中的单词
双端队列(deque)是一个高效的双端数据结构,可以用来反转字符串中的单词。
6.1 导入collections模块
首先,需要导入collections模块。
from collections import deque
6.2 将字符串分割成单词列表
然后,将字符串分割成单词列表。
input_string = "Hello world this is Python"
words_list = input_string.split()
6.3 使用双端队列反转单词列表
使用双端队列将单词列表反转。
dq = deque(words_list)
reversed_words_list = []
while dq:
reversed_words_list.append(dq.pop())
6.4 将反转后的单词列表重新组合成字符串
最后,使用join()方法将反转后的单词列表重新组合成字符串。
reversed_string = ' '.join(reversed_words_list)
print(reversed_string)
完整代码如下:
from collections import deque
input_string = "Hello world this is Python"
words_list = input_string.split()
dq = deque(words_list)
reversed_words_list = []
while dq:
reversed_words_list.append(dq.pop())
reversed_string = ' '.join(reversed_words_list)
print(reversed_string)
输出结果为:
Python is this world Hello
七、使用生成器函数反转字符串中的单词
生成器函数是一种特殊的函数,可以用来反转字符串中的单词。
7.1 定义生成器函数
首先,需要定义一个生成器函数来反转字符串中的单词。
def reverse_words_generator(s):
words = s.split()
for word in reversed(words):
yield word
7.2 调用生成器函数
然后,调用生成器函数来反转字符串中的单词。
input_string = "Hello world this is Python"
reversed_words = reverse_words_generator(input_string)
reversed_string = ' '.join(reversed_words)
print(reversed_string)
输出结果为:
Python is this world Hello
八、使用链表反转字符串中的单词
链表是一种线性数据结构,可以用来反转字符串中的单词。
8.1 定义链表节点类
首先,需要定义一个链表节点类。
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
8.2 将字符串分割成单词列表
然后,将字符串分割成单词列表。
input_string = "Hello world this is Python"
words_list = input_string.split()
8.3 构建链表
使用链表节点类构建链表。
dummy = ListNode()
current = dummy
for word in words_list:
current.next = ListNode(word)
current = current.next
8.4 反转链表
反转链表。
prev = None
current = dummy.next
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
8.5 将反转后的链表转换成字符串
将反转后的链表转换成字符串。
reversed_words_list = []
current = prev
while current:
reversed_words_list.append(current.val)
current = current.next
reversed_string = ' '.join(reversed_words_list)
print(reversed_string)
完整代码如下:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
input_string = "Hello world this is Python"
words_list = input_string.split()
dummy = ListNode()
current = dummy
for word in words_list:
current.next = ListNode(word)
current = current.next
prev = None
current = dummy.next
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
reversed_words_list = []
current = prev
while current:
reversed_words_list.append(current.val)
current = current.next
reversed_string = ' '.join(reversed_words_list)
print(reversed_string)
输出结果为:
Python is this world Hello
通过以上方法,我们可以在Python中反转字符串中的单词。每种方法都有其独特的优势和适用场景,可以根据具体需求选择合适的方法。
相关问答FAQs:
反转字符串中的单词有什么具体的方法?
在Python中,可以使用多种方法来反转字符串中的单词。常见的做法是利用字符串的 split()
方法将字符串拆分成单词,然后使用 reverse()
方法或者切片操作反转单词的顺序,最后用 join()
方法将它们重新组合成一个字符串。例如:
s = "Hello World"
reversed_words = ' '.join(s.split()[::-1])
print(reversed_words) # 输出: World Hello
如何处理字符串中的标点符号?
如果字符串中包含标点符号,反转单词时可能需要先去掉标点或在反转后再处理。可以使用正则表达式来去除标点符号,或者在反转后根据需要进行处理。例如,使用 re
模块:
import re
s = "Hello, World!"
cleaned = re.sub(r'[^\w\s]', '', s)
reversed_words = ' '.join(cleaned.split()[::-1])
print(reversed_words) # 输出: World Hello
反转字符串中的单词时,保留空格的做法是什么?
在反转单词的同时,如果需要保留原字符串中的空格位置,可以通过记录空格的索引并在反转后重新插入空格来实现。这种方法相对复杂,但可以达到保留空格的效果。
s = " Hello World "
words = s.split() # 拆分单词
reversed_words = ' '.join(words[::-1]) # 反转单词
# 计算原始空格数量
space_count = [len(x) for x in s.split(' ') if x == '']
# 将空格插入到反转后的字符串中
for count in space_count:
reversed_words = reversed_words + ' ' * count
print(reversed_words) # 输出: " World Hello "
这些方法都能帮助用户有效地反转字符串中的单词,根据具体需求选择合适的方式。
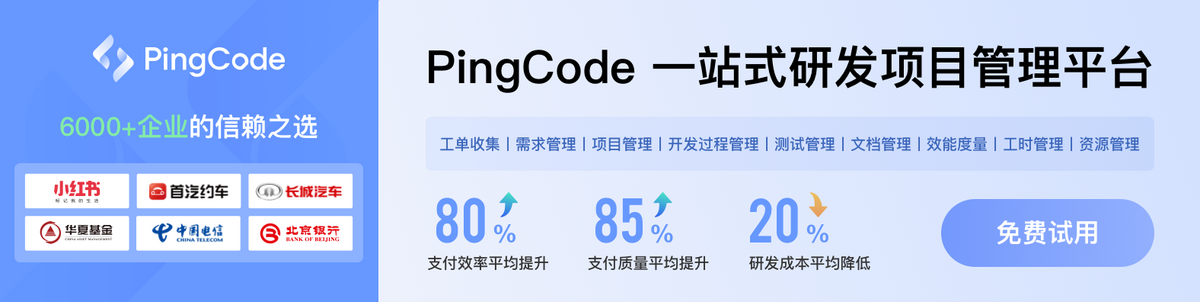