在Python中重新开启一行可以通过使用换行字符 \n
、三引号字符串、以及字符串连接和格式化等方式实现。其中,使用换行字符 \n
是最常见且简便的方法。这种方法能够在字符串内部插入一个换行符,使得输出时从新的一行开始。例如,通过使用 print("Hello\nWorld")
可以实现换行输出。接下来,我们将详细探讨这些方法及其应用场景。
一、使用换行字符 \n
基本用法
换行字符 \n
是Python中最直接的方式来开启新的一行。无论是单行字符串还是多行字符串,都可以使用这个字符来标记一个新的行开始。
print("Hello\nWorld")
上述代码输出结果为:
Hello
World
多行字符串中的应用
即使在复杂的多行字符串中,\n
仍然非常有用。可以将其嵌入到任何位置来精确控制换行。
text = "This is the first line.\nThis is the second line.\nAnd this is the third line."
print(text)
输出结果为:
This is the first line.
This is the second line.
And this is the third line.
二、使用三引号字符串
定义多行字符串
三引号字符串(使用 """
或 '''
)允许在定义字符串时直接换行,而不需要显式使用换行字符。这种方法对于编写大型文本块或文档字符串非常方便。
text = """This is the first line.
This is the second line.
And this is the third line."""
print(text)
输出结果为:
This is the first line.
This is the second line.
And this is the third line.
保持原始格式
三引号字符串还可以保持文本的原始格式,包括空格和换行符。这对于预设格式的文本(如代码片段或诗歌)非常有用。
code = """def hello_world():
print("Hello, World!")
"""
print(code)
输出结果为:
def hello_world():
print("Hello, World!")
三、字符串连接与格式化
使用加号连接
可以通过加号(+
)来连接字符串,并在需要换行的地方插入换行字符 \n
。
text = "This is the first line." + "\n" + "This is the second line."
print(text)
输出结果为:
This is the first line.
This is the second line.
使用 .format()
方法
Python的字符串格式化方法 .format()
也可以用于创建多行字符串。尽管这种方法不如直接使用 \n
简便,但在某些情况下可能更具可读性和灵活性。
text = "This is the first line.\n{}\nAnd this is the third line.".format("This is the second line.")
print(text)
输出结果为:
This is the first line.
This is the second line.
And this is the third line.
四、使用 f-string(格式化字符串)
基本用法
Python 3.6 引入了格式化字符串(f-string),使得在字符串中嵌入表达式更加简便。这种方法可以与换行字符 \n
结合使用。
line1 = "This is the first line."
line2 = "This is the second line."
text = f"{line1}\n{line2}\nAnd this is the third line."
print(text)
输出结果为:
This is the first line.
This is the second line.
And this is the third line.
动态内容生成
f-string 非常适合生成动态内容,可以在字符串中嵌入变量和表达式。
name = "John"
age = 30
text = f"Name: {name}\nAge: {age}"
print(text)
输出结果为:
Name: John
Age: 30
五、使用 os.linesep
提供平台无关的换行符
基本用法
在跨平台开发中,换行符可能会有所不同,例如 Windows 使用 \r\n
,而 Unix 使用 \n
。为了解决这个问题,可以使用 os.linesep
来获得平台无关的换行符。
import os
text = f"First line{os.linesep}Second line"
print(text)
输出结果为:
First line
Second line
实际应用
通过 os.linesep
,可以确保在不同平台上都能正确处理换行符,避免因换行符差异导致的错误。
import os
lines = ["First line", "Second line", "Third line"]
text = os.linesep.join(lines)
print(text)
输出结果为:
First line
Second line
Third line
六、使用 textwrap
模块
基本用法
textwrap
模块提供了许多处理文本的方法,其中 fill
方法可以自动换行。
import textwrap
text = "This is a very long text that we want to wrap into new lines for better readability."
wrapped_text = textwrap.fill(text, width=40)
print(wrapped_text)
输出结果为:
This is a very long text that we
want to wrap into new lines for
better readability.
高级用法
textwrap
还提供了更高级的功能,如缩进和减少空格。
import textwrap
text = " This is a very long text that we want to wrap into new lines for better readability."
dedented_text = textwrap.dedent(text)
wrapped_text = textwrap.fill(dedented_text, width=40)
print(wrapped_text)
输出结果为:
This is a very long text that we
want to wrap into new lines for
better readability.
七、使用 io.StringIO
模块
基本用法
io.StringIO
模块提供了一个内存中的文本流,可以使用该流来构建复杂的多行文本。
import io
output = io.StringIO()
output.write("This is the first line.\n")
output.write("This is the second line.\n")
output.write("And this is the third line.")
print(output.getvalue())
output.close()
输出结果为:
This is the first line.
This is the second line.
And this is the third line.
动态生成内容
StringIO
非常适合动态生成多行内容,例如从循环中生成内容。
import io
output = io.StringIO()
for i in range(1, 4):
output.write(f"This is line {i}\n")
print(output.getvalue())
output.close()
输出结果为:
This is line 1
This is line 2
This is line 3
八、使用 print
函数的 sep
和 end
参数
自定义分隔符
print
函数允许通过 sep
参数自定义多个字符串之间的分隔符。
print("First line", "Second line", sep="\n")
输出结果为:
First line
Second line
自定义结束符
print
函数的 end
参数可以自定义输出结束时的字符,默认是换行符 \n
。
print("First line", end="\n---\n")
print("Second line")
输出结果为:
First line
---
Second line
九、使用列表推导式生成多行字符串
基本用法
列表推导式是一种简洁的生成多行字符串的方法,可以与换行字符结合使用。
lines = [f"This is line {i}" for i in range(1, 4)]
text = "\n".join(lines)
print(text)
输出结果为:
This is line 1
This is line 2
This is line 3
动态生成内容
列表推导式可以用于动态生成复杂的多行内容。
data = {"name": "John", "age": 30, "city": "New York"}
text = "\n".join([f"{key}: {value}" for key, value in data.items()])
print(text)
输出结果为:
name: John
age: 30
city: New York
十、总结
在Python中重新开启一行有多种方法,每种方法都有其特定的应用场景和优势。换行字符 \n
是最常见且直接的方法,三引号字符串 适用于定义多行文本,字符串连接与格式化 提供了更多的灵活性,f-string 使得嵌入表达式更加简便,os.linesep
确保跨平台兼容性,textwrap
模块 提供了高级文本处理功能,io.StringIO
模块 适合动态生成内容,print
函数的 sep
和 end
参数 提供了自定义输出格式的能力,列表推导式 则是一种简洁的生成多行字符串的方法。根据具体需求,选择最适合的方法可以提高代码的可读性和维护性。
相关问答FAQs:
如何在Python中实现换行输出?
在Python中,可以通过使用换行符 \n
来实现换行输出。例如,使用 print("Hello\nWorld")
将会在控制台上输出:
Hello
World
这种方式可以灵活地在任何字符串中插入换行。
在Python中可以使用哪些方法来实现多行字符串的输出?
在Python中,可以使用三引号 '''
或 """
来定义多行字符串。这样可以方便地在代码中书写多行内容,而不需要手动插入换行符。例如:
multi_line_string = """这是第一行
这是第二行
这是第三行"""
print(multi_line_string)
这样就可以直接输出包含多行的内容。
如何在Python中通过字符串格式化实现换行?
使用字符串格式化时,可以将换行符 \n
包含在字符串中。例如:
name = "Alice"
age = 30
formatted_string = f"姓名: {name}\n年龄: {age}"
print(formatted_string)
这种方式既可以实现动态插入内容,又能控制换行输出。
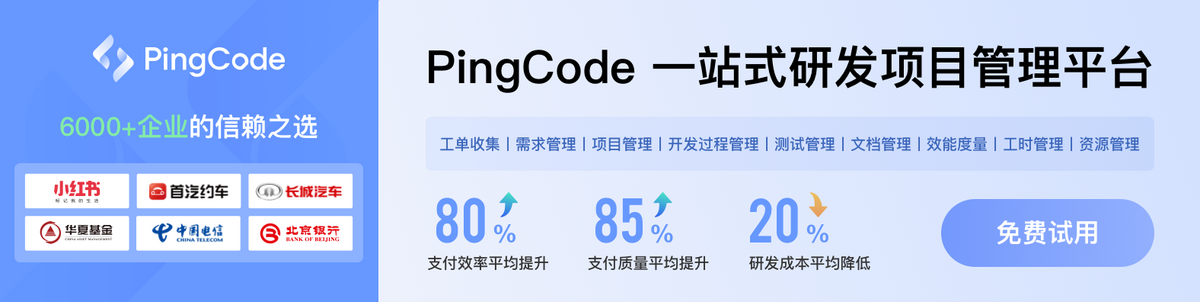