Python 中格式化输出字符串的方法有多种,包括使用百分号 (%) 运算符、str.format() 方法以及更现代的 f-string(格式化字符串字面量)。其中,f-string 是最推荐的方式,因为它简洁、直观且功能强大。百分号运算符方法、str.format() 方法、f-string 方法。 下面我将详细介绍 f-string 方法。
f-string 方法: f-string 是 Python 3.6 引入的一种格式化字符串的方式。它使用大括号 {} 包含表达式,并在字符串前加上字母 f 来表示是一个 f-string。f-string 不仅简洁,而且支持多种格式化选项,允许嵌入表达式和调用函数,非常灵活。例如:
name = "Alice"
age = 30
formatted_string = f"Name: {name}, Age: {age}"
print(formatted_string)
输出将是:
Name: Alice, Age: 30
在此基础上,我们可以进一步探索 f-string 的更多功能和用法。
一、百分号 (%) 运算符
1、基本用法
百分号 (%) 运算符是 Python 中最早的字符串格式化方法。它类似于 C 语言中的 printf 格式化。基本用法是用 %s 表示字符串、%d 表示整数、%f 表示浮点数等。
name = "Alice"
age = 30
formatted_string = "Name: %s, Age: %d" % (name, age)
print(formatted_string)
输出:
Name: Alice, Age: 30
2、格式化浮点数
使用 %f 来格式化浮点数,可以指定精度,例如保留两位小数:
pi = 3.141592653589793
formatted_string = "Pi: %.2f" % pi
print(formatted_string)
输出:
Pi: 3.14
二、str.format() 方法
1、基本用法
str.format() 方法是 Python 2.7 和 3.0 引入的更强大的字符串格式化方法。它允许使用大括号 {} 作为占位符,并在后面使用 .format() 方法填充数据。
name = "Alice"
age = 30
formatted_string = "Name: {}, Age: {}".format(name, age)
print(formatted_string)
输出:
Name: Alice, Age: 30
2、命名参数
str.format() 方法还允许使用命名参数,增加了代码的可读性:
formatted_string = "Name: {name}, Age: {age}".format(name="Alice", age=30)
print(formatted_string)
输出:
Name: Alice, Age: 30
3、格式化选项
str.format() 方法还提供了丰富的格式化选项,例如对齐、填充、精度控制等:
pi = 3.141592653589793
formatted_string = "Pi: {:.2f}".format(pi)
print(formatted_string)
输出:
Pi: 3.14
三、f-string 方法
1、基本用法
f-string 是 Python 3.6 引入的格式化字符串字面量,它通过在字符串前加 f,并在大括号 {} 中嵌入表达式来实现格式化。f-string 比前两种方法更简洁、直观。
name = "Alice"
age = 30
formatted_string = f"Name: {name}, Age: {age}"
print(formatted_string)
输出:
Name: Alice, Age: 30
2、表达式嵌入
f-string 允许在大括号内嵌入任意 Python 表达式,包括函数调用:
import math
formatted_string = f"The square root of 16 is {math.sqrt(16)}"
print(formatted_string)
输出:
The square root of 16 is 4.0
3、格式化选项
f-string 同样支持丰富的格式化选项:
pi = 3.141592653589793
formatted_string = f"Pi: {pi:.2f}"
print(formatted_string)
输出:
Pi: 3.14
四、总结
Python 提供了多种格式化输出字符串的方法,每种方法都有其独特的优点和适用场景。百分号 (%) 运算符适合简单的格式化需求,str.format() 方法提供了更强大的功能,而 f-string 则结合了简洁性和强大功能,是当前最推荐的方式。无论选择哪种方法,都可以根据具体需求进行灵活运用。
相关问答FAQs:
如何在Python中使用f-string格式化输出字符串?
f-string是Python 3.6及以上版本中提供的一种方便的字符串格式化方法。您可以在字符串前加上字母“f”,并在字符串中使用花括号{}来插入变量。例如:
name = "Alice"
age = 30
formatted_string = f"My name is {name} and I am {age} years old."
print(formatted_string)
这样可以直接在字符串中引用变量,格式化输出的效果直观且易于理解。
Python中有哪些方法可以格式化浮点数?
在Python中,格式化浮点数可以使用多种方法,如使用格式化字符串、format()
方法或round()
函数。例如:
value = 3.14159
formatted_value = "{:.2f}".format(value) # 使用format方法
print(formatted_value) # 输出:3.14
rounded_value = round(value, 2) # 使用round函数
print(rounded_value) # 输出:3.14
这些方法可以帮助您控制小数点后的位数,确保输出符合需求。
如何在Python中格式化输出日期和时间?
在Python中,可以使用datetime
模块来格式化日期和时间。通过strftime()
方法,您可以定义输出的格式。例如:
from datetime import datetime
now = datetime.now()
formatted_date = now.strftime("%Y-%m-%d %H:%M:%S")
print(formatted_date) # 输出当前日期和时间,例如:2023-10-03 14:45:00
这种方式使您能够灵活地展示日期和时间,满足不同场景的需求。
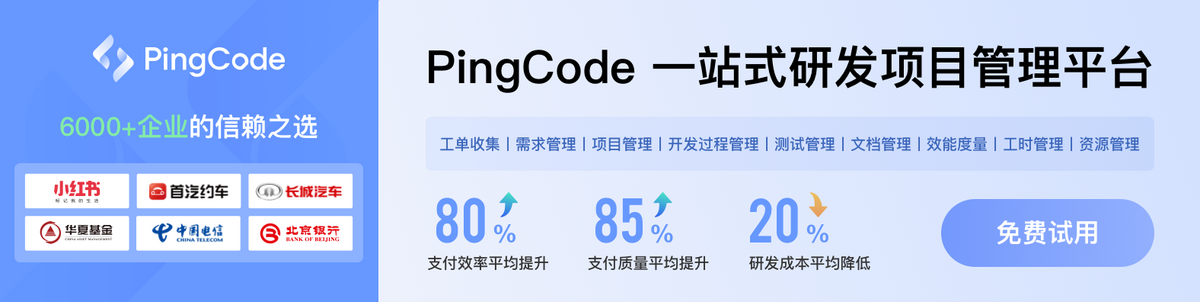