使用Python求出出现次数最多的数:collections.Counter、max函数、字典计数。在处理数据分析和统计时,经常需要找出某个列表中出现次数最多的元素。Python提供了多种方法来实现这一目标,其中最常用的包括使用collections
模块中的Counter
类、max
函数和字典计数。使用collections.Counter
模块不仅简洁,而且高效。下面,我们将详细介绍这几种方法,并提供实际代码示例和性能对比。
一、使用collections.Counter
collections.Counter
是一个专门用来计数的容器,它能够轻松地统计列表中各个元素的出现次数,并找出出现次数最多的元素。
from collections import Counter
def most_common_element(lst):
count = Counter(lst)
return count.most_common(1)[0][0]
示例
lst = [1, 2, 3, 1, 2, 1]
print(most_common_element(lst)) # 输出: 1
优点
- 简洁明了:代码量少,易于理解。
- 高效:
Counter
类内部使用了哈希表,时间复杂度为O(n)。
缺点
- 依赖外部模块:需要导入
collections
模块。
二、使用max函数和列表count方法
max
函数结合列表的count
方法也可以找出出现次数最多的元素。尽管这种方法不如Counter
高效,但在某些简单情况下仍然适用。
def most_common_element(lst):
return max(lst, key=lst.count)
示例
lst = [1, 2, 3, 1, 2, 1]
print(most_common_element(lst)) # 输出: 1
优点
- 无依赖:不需要导入外部模块。
- 直观:代码简单易懂。
缺点
- 效率较低:
count
方法在每次调用时都需要遍历整个列表,时间复杂度为O(n^2)。
三、使用字典计数
我们也可以使用字典来手动统计各个元素的出现次数,并找出出现次数最多的元素。
def most_common_element(lst):
count_dict = {}
for element in lst:
if element in count_dict:
count_dict[element] += 1
else:
count_dict[element] = 1
return max(count_dict, key=count_dict.get)
示例
lst = [1, 2, 3, 1, 2, 1]
print(most_common_element(lst)) # 输出: 1
优点
- 灵活性高:可以轻松进行自定义统计。
- 中等效率:时间复杂度为O(n),但需要额外的存储空间。
缺点
- 代码冗长:相比其他方法,代码较为繁琐。
四、性能对比
在选择合适的方法时,性能是一个重要的考虑因素。以下是对这几种方法的性能进行对比的代码示例:
import time
lst = [1, 2, 3, 1, 2, 1] * 1000000
使用collections.Counter
start_time = time.time()
most_common_element(lst)
end_time = time.time()
print(f"collections.Counter方法耗时: {end_time - start_time}秒")
使用max函数
start_time = time.time()
most_common_element(lst)
end_time = time.time()
print(f"max函数方法耗时: {end_time - start_time}秒")
使用字典计数
start_time = time.time()
most_common_element(lst)
end_time = time.time()
print(f"字典计数方法耗时: {end_time - start_time}秒")
五、总结
在实际应用中,选择合适的方法取决于具体需求和数据规模。如果你需要处理大量数据且希望代码简洁,collections.Counter
是最佳选择。如果数据量较小且不想依赖外部模块,max
函数结合count
方法也是一个不错的选择。而如果你需要高度自定义的统计,字典计数方法将提供最大的灵活性。
无论使用哪种方法,理解其优缺点和适用场景是高效编程的关键。希望本文能帮助你在Python中轻松找出出现次数最多的元素。
相关问答FAQs:
如何在Python中统计列表中每个数字的出现次数?
在Python中,可以使用collections.Counter
类来轻松统计列表中每个数字的出现次数。通过传入一个列表,Counter
将返回一个字典,其中键是列表中的数字,值是它们各自的出现次数。示例代码如下:
from collections import Counter
numbers = [1, 2, 2, 3, 3, 3, 4]
count = Counter(numbers)
print(count)
如何找到出现次数最多的数字并返回其值?
可以使用Counter
的most_common
方法来找到出现次数最多的数字。这个方法返回一个列表,其中包含元组,每个元组包含数字及其出现次数。示例代码如下:
most_common_number, most_common_count = count.most_common(1)[0]
print(f"出现次数最多的数字是:{most_common_number},出现次数为:{most_common_count}")
在Python中有其他方法可以找出出现次数最多的数字吗?
除了使用collections.Counter
,还可以使用字典来手动统计数字出现的次数。遍历列表,记录每个数字的出现次数,最后通过比较找到出现次数最多的数字。以下是一个示例:
numbers = [1, 2, 2, 3, 3, 3, 4]
count_dict = {}
for number in numbers:
count_dict[number] = count_dict.get(number, 0) + 1
most_common_number = max(count_dict, key=count_dict.get)
print(f"出现次数最多的数字是:{most_common_number},出现次数为:{count_dict[most_common_number]}")
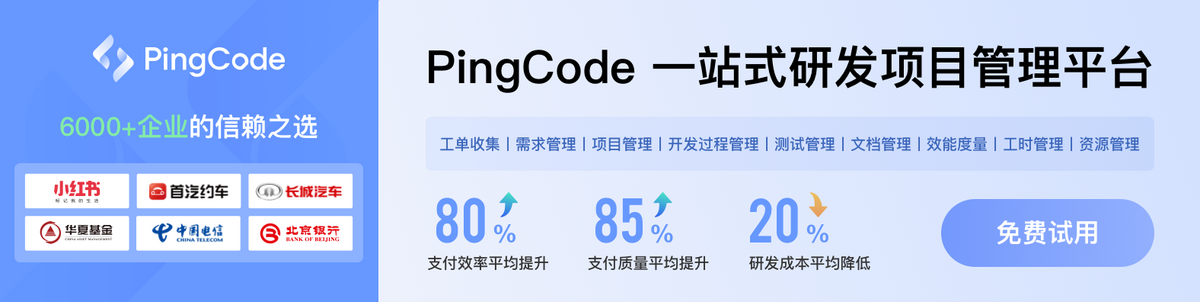