Python对文件夹下生成文档的方法有:使用os模块创建目录、使用open函数创建文件、利用with语句自动管理文件资源。以下是具体步骤。首先,使用os模块可以方便地操作文件系统。其次,open函数用于创建和写入文件,配合with语句可以确保文件在操作完成后自动关闭,防止资源泄漏。
一、使用os模块创建目录
Python的os模块提供了丰富的文件和目录操作功能。首先,我们需要导入os模块,然后使用os.makedirs()函数创建目录。这是一个递归创建目录的函数,意味着如果指定的路径中有不存在的目录,os.makedirs()将自动创建所有必要的目录。使用示例如下:
import os
def create_directory(path):
try:
os.makedirs(path, exist_ok=True)
print(f"Directory '{path}' created successfully")
except Exception as e:
print(f"Error creating directory '{path}': {e}")
Example usage
create_directory("/path/to/your/new_directory")
在这个示例中,create_directory
函数接收一个路径参数,并尝试创建该路径下的所有目录。exist_ok=True
参数保证如果目录已经存在,不会抛出异常。
二、使用open函数创建文件
创建完目录后,我们可以在该目录下创建文件并写入内容。Python内置的open()函数可以方便地打开一个文件进行读写操作。结合with语句,可以确保文件在操作完成后自动关闭。示例如下:
def create_file(file_path, content):
try:
with open(file_path, 'w') as file:
file.write(content)
print(f"File '{file_path}' created successfully")
except Exception as e:
print(f"Error creating file '{file_path}': {e}")
Example usage
create_file("/path/to/your/new_directory/example.txt", "This is a sample content.")
在这个示例中,create_file
函数接收文件路径和内容作为参数,打开文件并写入内容。使用with语句确保文件在操作完成后自动关闭。
三、利用with语句自动管理文件资源
使用with语句是Python中处理文件的最佳实践。它不仅可以确保文件操作的安全性,还能简化代码的编写。以下是一个更复杂的示例,展示如何在创建多个文件并写入不同内容:
def create_multiple_files(base_path, files_content):
try:
os.makedirs(base_path, exist_ok=True)
for file_name, content in files_content.items():
file_path = os.path.join(base_path, file_name)
with open(file_path, 'w') as file:
file.write(content)
print(f"File '{file_path}' created successfully")
except Exception as e:
print(f"Error creating files in '{base_path}': {e}")
Example usage
files_content = {
"example1.txt": "This is the first example content.",
"example2.txt": "This is the second example content.",
}
create_multiple_files("/path/to/your/new_directory", files_content)
在这个示例中,create_multiple_files
函数接收一个基础路径和一个包含文件名与内容的字典。函数首先创建目录,然后遍历字典,创建并写入每个文件。
四、处理大文件和多线程
对于大文件或需要并行处理的情况,可以使用多线程或多进程技术来提高效率。Python的threading和multiprocessing模块提供了强大的并发处理能力。以下是一个使用多线程的示例:
import os
from threading import Thread
def create_file_thread(file_path, content):
try:
with open(file_path, 'w') as file:
file.write(content)
print(f"File '{file_path}' created successfully")
except Exception as e:
print(f"Error creating file '{file_path}': {e}")
def create_multiple_files_threaded(base_path, files_content):
try:
os.makedirs(base_path, exist_ok=True)
threads = []
for file_name, content in files_content.items():
file_path = os.path.join(base_path, file_name)
thread = Thread(target=create_file_thread, args=(file_path, content))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
except Exception as e:
print(f"Error creating files in '{base_path}': {e}")
Example usage
files_content = {
"example1.txt": "This is the first example content.",
"example2.txt": "This is the second example content.",
}
create_multiple_files_threaded("/path/to/your/new_directory", files_content)
在这个示例中,我们使用threading模块创建多个线程,同时写入多个文件。每个线程负责创建一个文件并写入内容。
五、错误处理和日志记录
在文件操作过程中,错误处理和日志记录是非常重要的。Python的logging模块可以帮助我们记录运行时的信息,错误和调试信息。以下是一个结合logging模块的示例:
import os
import logging
def setup_logging(log_file):
logging.basicConfig(filename=log_file, level=logging.INFO,
format='%(asctime)s - %(levelname)s - %(message)s')
def create_file(file_path, content):
try:
with open(file_path, 'w') as file:
file.write(content)
logging.info(f"File '{file_path}' created successfully")
except Exception as e:
logging.error(f"Error creating file '{file_path}': {e}")
def create_multiple_files(base_path, files_content, log_file):
setup_logging(log_file)
try:
os.makedirs(base_path, exist_ok=True)
for file_name, content in files_content.items():
file_path = os.path.join(base_path, file_name)
create_file(file_path, content)
except Exception as e:
logging.error(f"Error creating files in '{base_path}': {e}")
Example usage
files_content = {
"example1.txt": "This is the first example content.",
"example2.txt": "This is the second example content.",
}
create_multiple_files("/path/to/your/new_directory", files_content, "/path/to/your/log_file.log")
在这个示例中,我们使用logging模块记录文件操作的成功和失败信息。setup_logging
函数配置了日志文件和日志级别。所有的日志信息将被写入指定的日志文件。
六、总结
通过以上步骤,我们可以使用Python高效地在文件夹下生成文档。使用os模块创建目录、open函数创建文件、利用with语句自动管理文件资源、多线程处理大文件和并发任务、错误处理和日志记录,这些都是我们在实际开发中常用的技巧。希望通过本文,您能对Python的文件操作有更深入的了解,并能在实际项目中灵活运用这些方法。
相关问答FAQs:
如何在Python中创建一个新的文件夹?
要在Python中创建新的文件夹,可以使用os
模块中的mkdir
或makedirs
方法。mkdir
用于创建单个目录,而makedirs
可以创建多层目录。例如:
import os
os.mkdir('new_folder') # 创建单个目录
os.makedirs('parent_folder/child_folder') # 创建多层目录
确保在创建目录之前检查该目录是否已存在,以避免引发异常。
Python如何读取文件夹中的所有文件?
要读取文件夹中的所有文件,可以使用os
模块中的listdir
方法。该方法将返回目录中所有文件和文件夹的列表。示例代码如下:
import os
files = os.listdir('your_folder_path')
for file in files:
print(file)
你可以结合os.path
模块来过滤特定类型的文件,比如只显示文本文件或图片文件。
如何使用Python生成文档并保存到指定文件夹?
生成文档可以使用docx
库(用于创建Word文档)或open
函数(用于创建文本文件)。以下是使用open
函数创建文本文件的示例:
with open('your_folder_path/document.txt', 'w') as file:
file.write('这是文档内容')
对于Word文档,首先需要安装python-docx
库,然后可以这样操作:
from docx import Document
doc = Document()
doc.add_heading('文档标题', level=1)
doc.add_paragraph('这是文档内容')
doc.save('your_folder_path/document.docx')
确保在保存时提供正确的文件路径,以便文档能够被成功创建。
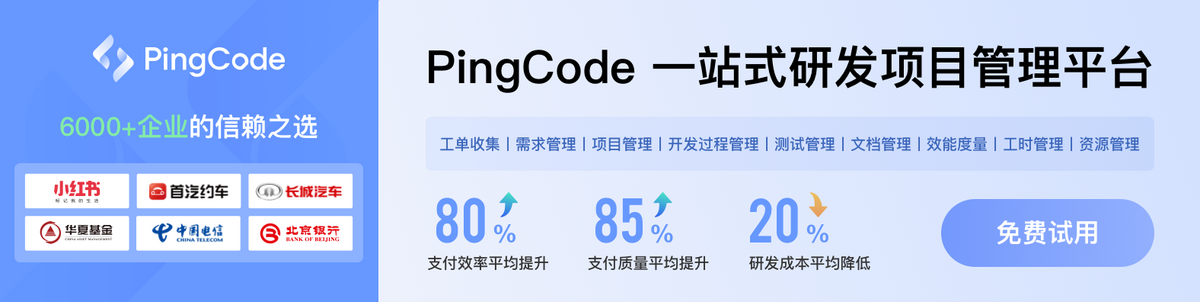