Python去掉字符串的空格和回车的方法包括使用strip()
、lstrip()
、rstrip()
、replace()
、re.sub()
等。具体方法包括:使用strip()
去掉字符串开头和结尾的空格和回车、replace()
替换字符串中的所有空格和回车、正则表达式re.sub()
灵活去除特定模式。下面将详细介绍这些方法及其应用场景。
一、strip()
、lstrip()
和rstrip()
方法
1. strip()
方法
strip()
方法用于去除字符串开头和结尾的空格、换行符以及其他指定字符。这是最常见和最简单的方法。
example_str = " Hello, World! \n"
clean_str = example_str.strip()
print(clean_str) # Output: "Hello, World!"
2. lstrip()
和 rstrip()
方法
lstrip()
方法用于去除字符串开头的空格和换行符,而rstrip()
方法用于去除字符串结尾的空格和换行符。
example_str = " Hello, World! \n"
l_clean_str = example_str.lstrip()
r_clean_str = example_str.rstrip()
print(l_clean_str) # Output: "Hello, World! \n"
print(r_clean_str) # Output: " Hello, World!"
二、replace()
方法
replace()
方法可以用来替换字符串中的所有空格和回车,这个方法非常灵活,可以指定要替换的字符和替换后的字符。
example_str = "Hello, \n World! "
clean_str = example_str.replace(" ", "").replace("\n", "")
print(clean_str) # Output: "Hello,World!"
三、正则表达式re.sub()
方法
正则表达式提供了更高级的字符串处理能力,可以用来去除字符串中的空格、回车以及其他特定模式的字符。
import re
example_str = "Hello, \n World! "
clean_str = re.sub(r'\s+', '', example_str)
print(clean_str) # Output: "Hello,World!"
四、结合以上方法
有时候,需要结合多种方法来处理复杂的字符串,例如同时去除空格、回车以及其他特殊字符。
import re
example_str = " Hello, \n World! \t"
使用 strip() 去除开头和结尾的空格和回车
stripped_str = example_str.strip()
使用 replace() 去除中间的空格和回车
clean_str = stripped_str.replace("\n", "").replace("\t", "").replace(" ", "")
print(clean_str) # Output: "Hello,World!"
五、处理多行字符串
对于多行字符串,特别是需要去除每一行开头和结尾的空格和回车,可以使用循环和strip()
方法。
multi_line_str = """
Hello, World!
This is a test string.
Let's clean it up.
"""
lines = multi_line_str.strip().split("\n")
clean_lines = [line.strip() for line in lines]
clean_str = " ".join(clean_lines)
print(clean_str) # Output: "Hello, World! This is a test string. Let's clean it up."
六、处理大型文本文件
当处理大型文本文件时,以上方法可能需要结合文件读取和写入操作。
with open('example.txt', 'r') as file:
lines = file.readlines()
clean_lines = [line.strip() for line in lines]
clean_str = " ".join(clean_lines)
with open('clean_example.txt', 'w') as file:
file.write(clean_str)
七、性能考虑
在处理大量数据时,性能是一个重要的考虑因素。使用strip()
、replace()
和正则表达式的方法在性能上有所不同。通常,strip()
和replace()
方法性能较好,而正则表达式方法较为灵活,但在处理超大文本时性能可能稍差。
八、总结
在Python中去除字符串的空格和回车有多种方法,包括strip()
、replace()
、正则表达式等。根据不同的需求选择合适的方法可以提高代码的效率和可读性。通过对字符串进行适当的处理,可以确保数据的清洁和一致性,这对于后续的数据处理和分析非常重要。
相关问答FAQs:
如何在Python中去除字符串开头和结尾的空格和回车?
在Python中,可以使用strip()
方法来去除字符串开头和结尾的空格和回车。这一方法不仅能够删除空格,还能移除换行符、制表符等。示例代码如下:
my_string = " Hello, World! \n"
cleaned_string = my_string.strip()
print(cleaned_string) # 输出: "Hello, World!"
是否可以只去掉字符串中的所有空格或回车?
若需去掉字符串中的所有空格或回车,可以使用replace()
方法。此方法允许用户替换特定字符或字符串。以下是一个示例:
my_string = " Hello, \n World! "
no_spaces = my_string.replace(" ", "").replace("\n", "")
print(no_spaces) # 输出: "Hello,World!"
在处理大型字符串时,有什么高效的方法去除空格和回车?
对于大型字符串,使用re
模块中的正则表达式能够实现更高效的空格和回车去除。通过这种方式,可以在一次操作中替换多个字符。示例代码如下:
import re
my_string = " Hello, \n World! "
cleaned_string = re.sub(r'\s+', '', my_string) # 去除所有空白字符
print(cleaned_string) # 输出: "Hello,World!"
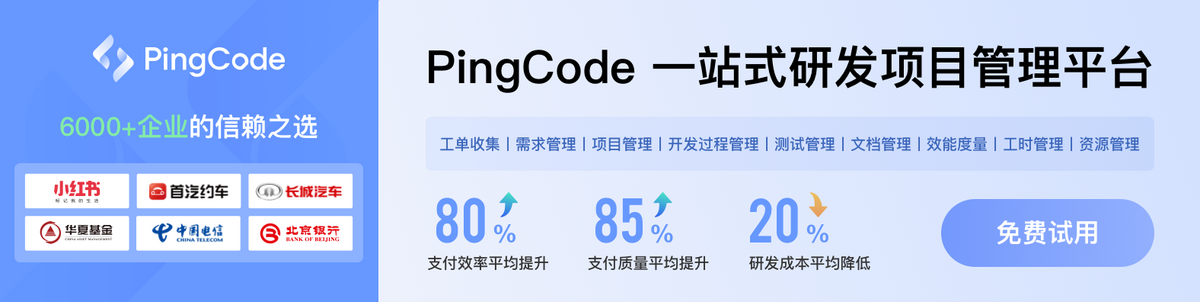