Python读取txt中的某一行:使用readlines()
方法、使用for
循环读取、使用linecache
模块。推荐使用linecache
模块,因为它更为简洁且高效。linecache
模块可以直接读取指定行而不需要遍历整个文件。
使用linecache
模块读取txt文件中的某一行的示例如下:
import linecache
def read_specific_line(file_path, line_number):
# 获取指定行内容
line = linecache.getline(file_path, line_number)
return line.strip()
file_path = 'example.txt'
line_number = 3
print(read_specific_line(file_path, line_number))
在上面的示例中,linecache.getline
函数会直接返回指定行的内容,这样既高效又方便。
一、使用readlines()方法
使用readlines()
方法可以将整个文件读取为一个列表,每个元素对应文件中的一行。这种方法适用于文件较小的情况,因为它会将整个文件加载到内存中。
def read_specific_line_with_readlines(file_path, line_number):
with open(file_path, 'r') as file:
lines = file.readlines()
if 0 < line_number <= len(lines):
return lines[line_number - 1].strip()
else:
return None
file_path = 'example.txt'
line_number = 3
print(read_specific_line_with_readlines(file_path, line_number))
在这个例子中,readlines()
方法会将整个文件读取到一个列表中,然后通过索引来获取指定行的内容。
二、使用for循环读取
使用for
循环逐行读取文件内容,当读取到指定行时,返回该行内容。这种方法适用于文件较大且不想将整个文件加载到内存中的情况。
def read_specific_line_with_for(file_path, line_number):
with open(file_path, 'r') as file:
for current_line_number, line in enumerate(file, start=1):
if current_line_number == line_number:
return line.strip()
return None
file_path = 'example.txt'
line_number = 3
print(read_specific_line_with_for(file_path, line_number))
在这个例子中,enumerate
函数会为每一行附加一个行号,当行号等于指定的行号时,返回该行内容。
三、使用linecache模块
linecache
模块是标准库中的一个模块,专门用于高效地读取文件中的特定行。它内部会缓存文件内容,以便于多次读取时提高效率。
import linecache
def read_specific_line_with_linecache(file_path, line_number):
line = linecache.getline(file_path, line_number)
return line.strip()
file_path = 'example.txt'
line_number = 3
print(read_specific_line_with_linecache(file_path, line_number))
在这个例子中,linecache.getline
函数会直接返回指定行的内容,不需要遍历整个文件,适用于频繁读取文件中某些特定行的场景。
四、性能比较
- readlines()方法:适用于文件较小且需要多次访问某些行的情况,因为它将整个文件加载到内存中,访问速度较快。
- for循环读取:适用于文件较大且只需要访问一次特定行的情况,因为它不需要将整个文件加载到内存中。
- linecache模块:适用于文件较大且需要频繁访问特定行的情况,因为它会缓存文件内容,提高访问效率。
五、实战应用
1、日志文件分析
在实际应用中,我们经常需要分析日志文件中的某些特定行,例如错误日志、警告日志等。使用上述方法可以方便地提取这些特定行进行分析。
def extract_error_logs(file_path, error_keyword):
with open(file_path, 'r') as file:
error_logs = [line.strip() for line in file if error_keyword in line]
return error_logs
file_path = 'server.log'
error_keyword = 'ERROR'
print(extract_error_logs(file_path, error_keyword))
在这个例子中,我们使用for
循环逐行读取日志文件,当发现行中包含错误关键字时,将该行加入错误日志列表中。
2、配置文件读取
在配置文件中,我们可能需要读取某些特定的配置项。使用上述方法可以方便地提取这些配置项的值。
def read_config_value(file_path, config_key):
with open(file_path, 'r') as file:
for line in file:
if line.startswith(config_key):
return line.split('=', 1)[1].strip()
return None
file_path = 'config.ini'
config_key = 'database_url'
print(read_config_value(file_path, config_key))
在这个例子中,我们使用for
循环逐行读取配置文件,当发现行以配置键开头时,提取其值。
3、大数据文件处理
在大数据文件处理中,可能需要随机访问文件中的某些行。使用linecache
模块可以高效地实现这一需求。
import linecache
def process_large_file(file_path, line_numbers):
results = []
for line_number in line_numbers:
line = linecache.getline(file_path, line_number)
results.append(line.strip())
return results
file_path = 'large_data.txt'
line_numbers = [1, 10, 100, 1000]
print(process_large_file(file_path, line_numbers))
在这个例子中,我们使用linecache
模块读取大数据文件中的指定行,将其结果存入列表中。
六、总结
通过本文的介绍,我们详细探讨了Python中如何读取txt文件中的某一行,分别使用了readlines()
方法、for
循环读取和linecache
模块。不同的方法适用于不同的场景,读者可以根据实际需求选择最合适的方法。特别是推荐使用linecache
模块,在需要频繁访问特定行的场景中,linecache
模块的效率是最高的。
相关问答FAQs:
如何在Python中指定读取文本文件的某一行?
在Python中,读取文本文件的特定行可以通过多种方法实现。常用的方法是打开文件后逐行读取,或者将文件的内容加载到列表中,然后通过索引访问特定行。以下是一个简单的示例:
with open('file.txt', 'r') as file:
lines = file.readlines()
specific_line = lines[2] # 读取第三行(索引从0开始)
print(specific_line)
这种方式有效且易于理解,适合处理小型文件。
读取文件时,如何处理异常情况?
在读取文本文件时,可能会遇到文件不存在或权限问题等异常情况。为了确保代码的健壮性,可以使用try-except
语句来捕捉这些异常。示例如下:
try:
with open('file.txt', 'r') as file:
lines = file.readlines()
specific_line = lines[2]
except FileNotFoundError:
print("文件未找到,请检查文件路径。")
except IndexError:
print("指定的行号超出了文件的行数。")
这种方式能够帮助用户更好地理解出现的问题并进行相应的修正。
是否可以在不加载整个文件的情况下读取特定行?
是的,可以通过使用enumerate()
函数结合文件对象的迭代来逐行读取而不将整个文件加载到内存中。这样做在处理大型文件时更为高效。例如:
line_number = 2 # 想要读取的行号
with open('file.txt', 'r') as file:
for current_line_number, line in enumerate(file):
if current_line_number == line_number:
print(line)
break
这种方法允许你在找到目标行时立即停止读取,从而节省了资源。
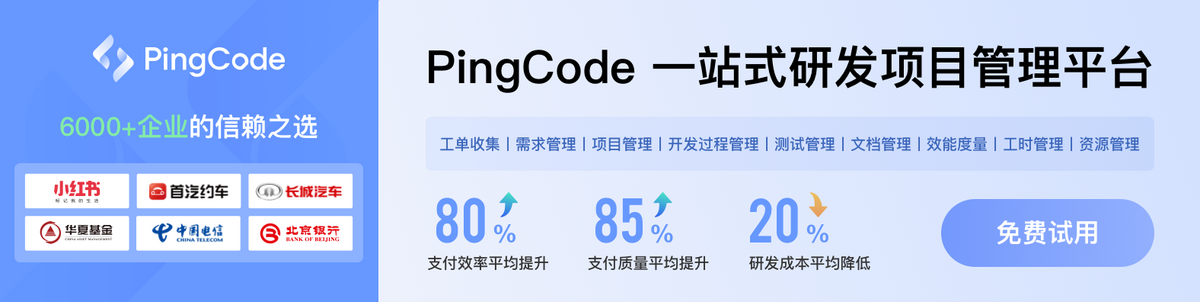