Python如何显示代码执行了多长时间这个问题可以通过多种方法来解决,包括使用内置的time
模块、timeit
模块以及其他第三方库如cProfile
等。使用time模块、使用timeit模块、使用cProfile模块是几种常见的方法。下面将详细介绍如何使用这些方法来测量Python代码执行的时间。
一、使用time模块
1、基本用法
Python的time
模块非常简单易用,可以通过获取当前时间戳来计算代码执行的时间。具体步骤如下:
import time
start_time = time.time()
需要测量的代码段
end_time = time.time()
execution_time = end_time - start_time
print(f"Execution Time: {execution_time} seconds")
详细描述:首先,使用time.time()
获取代码执行前的时间戳,然后在代码执行完成后再次获取时间戳,通过减法计算出代码的执行时间。这种方法适用于大多数简单的时间测量需求。
2、使用time.perf_counter()
time.perf_counter()
提供了更高精度的时间测量,适用于需要精确测量的场景。
import time
start_time = time.perf_counter()
需要测量的代码段
end_time = time.perf_counter()
execution_time = end_time - start_time
print(f"Execution Time: {execution_time} seconds")
二、使用timeit模块
timeit
模块是Python标准库中专门用于计时的小工具,非常适合用于测量小段代码的执行时间。
1、使用timeit.timeit()
可以直接在代码中使用timeit.timeit()
函数来测量特定代码段的执行时间。
import timeit
code_to_test = """
a = [i for i in range(1000)]
"""
execution_time = timeit.timeit(code_to_test, number=1000)
print(f"Execution Time: {execution_time} seconds")
详细描述:timeit.timeit()
函数接受一个字符串形式的代码段以及一个可选的参数number
,表示代码段将被执行的次数。通过多次执行来获得更稳定的时间测量结果。
2、使用timeit.Timer()
timeit.Timer
类提供了更多的灵活性,可以用于复杂的时间测量需求。
import timeit
def test_code():
a = [i for i in range(1000)]
timer = timeit.Timer(test_code)
execution_time = timer.timeit(number=1000)
print(f"Execution Time: {execution_time} seconds")
三、使用cProfile模块
cProfile
模块是Python内置的性能分析工具,适用于需要详细分析代码性能的场景。
1、基本用法
可以通过cProfile.run()
函数来运行并分析代码段。
import cProfile
def test_code():
a = [i for i in range(1000)]
cProfile.run('test_code()')
详细描述:cProfile.run()
函数会输出一个详细的性能分析报告,包括每个函数的调用次数、总耗时、每次调用的平均耗时等。
2、使用Profile类
Profile
类提供了更灵活的使用方式,可以手动控制性能分析的开始和结束。
import cProfile
def test_code():
a = [i for i in range(1000)]
profiler = cProfile.Profile()
profiler.enable()
test_code()
profiler.disable()
profiler.print_stats()
四、使用第三方库
除了Python内置的模块之外,还有许多第三方库可以用于测量代码执行时间,比如line_profiler
和memory_profiler
。
1、使用line_profiler
line_profiler
可以精确到每一行代码的执行时间,适用于需要详细分析代码性能的场景。
from line_profiler import LineProfiler
def test_code():
a = [i for i in range(1000)]
profiler = LineProfiler()
profiler.add_function(test_code)
profiler.enable_by_count()
test_code()
profiler.print_stats()
2、使用memory_profiler
memory_profiler
不仅可以测量代码执行时间,还可以监控内存使用情况。
from memory_profiler import profile
@profile
def test_code():
a = [i for i in range(1000)]
test_code()
五、最佳实践
1、选择合适的工具
在不同的场景下,选择合适的时间测量工具非常重要。对于简单的时间测量需求,可以使用time
模块;对于需要精确时间测量的场景,可以使用timeit
模块;而对于复杂的性能分析需求,则可以使用cProfile
模块或第三方库。
2、避免测量干扰
在进行时间测量时,要尽量避免其他因素的干扰,比如系统负载、网络延迟等。可以通过多次测量取平均值的方式来获得更稳定的时间测量结果。
3、分析和优化
在获得时间测量结果之后,要对代码进行详细分析和优化,找出性能瓶颈并进行针对性的优化。可以通过调整算法、减少不必要的计算等方式来提高代码的执行效率。
六、总结
通过上述方法,我们可以有效地测量Python代码的执行时间,从而帮助我们分析和优化代码性能。使用time模块、使用timeit模块、使用cProfile模块是几种常见且高效的方法,选择合适的工具和方法可以事半功倍。在实践中,结合具体需求和场景,灵活运用这些方法,可以更好地掌握代码的执行时间,提高代码的性能和效率。
相关问答FAQs:
如何在Python中测量代码执行时间?
在Python中,可以使用time
模块中的time()
函数或timeit
模块来测量代码的执行时间。简单的方法是使用time()
记录代码执行前后的时间差。例如,使用start_time = time.time()
记录开始时间,执行代码后,再记录结束时间end_time = time.time()
,然后通过execution_time = end_time - start_time
计算执行时间。
使用timeit
模块有什么优势?timeit
模块专门用于测量小段代码的执行时间,能提供更精确的结果。它会自动多次运行代码并计算平均执行时间,消除偶然因素的影响。使用timeit.timeit()
方法,可以轻松获取运行时间并进行比较,非常适合用于性能测试。
如何在Jupyter Notebook中显示代码执行时间?
在Jupyter Notebook中,可以使用%%time
或%%timeit
魔法命令来测量代码块的执行时间。只需在代码块的顶部添加这些命令,执行后会在输出中显示代码的执行时间,方便快速查看性能。
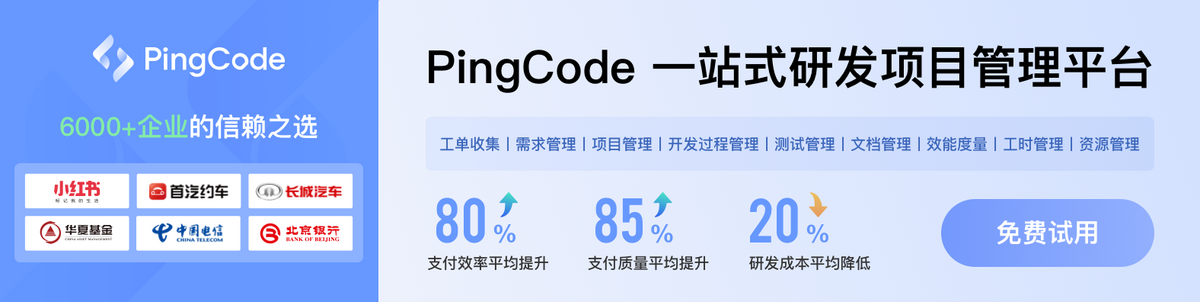