通过Python获取网页中的文字元素的核心步骤包括:选择正确的工具、理解HTML结构、编写抓取代码、处理动态网页和优化代码性能。其中,选择正确的工具是最关键的一步。Python有许多强大的库,如BeautifulSoup、Scrapy和Selenium,可以帮助你高效地抓取网页内容。下面我们将详细展开如何通过Python获取网页中的文字元素。
一、选择正确的工具
1. BeautifulSoup
BeautifulSoup是一个非常流行的Python库,用于解析HTML和XML文档。它提供了Pythonic的方式来处理这些文档,可以轻松地找到、导航和修改树结构。
安装BeautifulSoup
pip install beautifulsoup4
pip install lxml
使用BeautifulSoup解析HTML
from bs4 import BeautifulSoup
html_doc = """
<html><head><title>The Dormouse's story</title></head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
"""
soup = BeautifulSoup(html_doc, 'lxml')
print(soup.title.string)
print(soup.get_text())
2. Scrapy
Scrapy是一个强大的开源网页抓取框架,适用于大型项目。它提供了许多内置功能,如处理请求、解析内容和存储抓取的数据。
安装Scrapy
pip install scrapy
使用Scrapy抓取网页
创建一个新的Scrapy项目:
scrapy startproject myproject
cd myproject
scrapy genspider example example.com
编辑生成的example.py
文件,添加抓取逻辑:
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['http://example.com']
def parse(self, response):
for title in response.css('title::text').getall():
yield {'title': title}
运行Scrapy爬虫:
scrapy crawl example
3. Selenium
Selenium是一个自动化测试工具,可以模拟用户在浏览器中的操作,非常适合处理需要JavaScript渲染的动态网页。
安装Selenium
pip install selenium
使用Selenium抓取动态网页
from selenium import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get('http://example.com')
title = driver.find_element(By.TAG_NAME, 'title').text
print(title)
driver.quit()
二、理解HTML结构
在抓取网页内容之前,理解HTML文档的结构是非常重要的。HTML文档由一系列标签组成,这些标签定义了网页的布局和内容。常见的标签包括<html>
, <head>
, <body>
, <div>
, <p>
, <a>
等。
1. 标签和属性
每个HTML标签都有自己的属性,例如<a>
标签的href
属性用于定义链接的目标地址。通过分析这些标签和属性,可以确定需要抓取的具体内容。
示例HTML文档
<html>
<head>
<title>Example Domain</title>
</head>
<body>
<div>
<h1>Example Domain</h1>
<p>This domain is for use in illustrative examples in documents.</p>
<p><a href="http://www.iana.org/domains/example">More information...</a></p>
</div>
</body>
</html>
2. DOM树结构
HTML文档可以表示为一个树结构,称为DOM(文档对象模型)。理解DOM树结构有助于编写更高效的抓取代码。
示例DOM树
html
├── head
│ └── title
└── body
└── div
├── h1
├── p
└── p
└── a
三、编写抓取代码
1. 使用BeautifulSoup抓取网页内容
BeautifulSoup可以轻松地解析HTML文档,并提供多种方法来查找和提取内容。
示例代码
from bs4 import BeautifulSoup
import requests
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'lxml')
title = soup.title.string
print('Title:', title)
paragraphs = soup.find_all('p')
for p in paragraphs:
print('Paragraph:', p.get_text())
2. 使用Scrapy抓取网页内容
Scrapy适用于需要抓取大量数据的情况,可以轻松处理多页抓取和数据存储。
示例代码
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['http://example.com']
def parse(self, response):
title = response.xpath('//title/text()').get()
print('Title:', title)
paragraphs = response.xpath('//p/text()').getall()
for p in paragraphs:
print('Paragraph:', p)
3. 使用Selenium处理动态网页
Selenium可以模拟用户在浏览器中的操作,适合处理需要JavaScript渲染的动态网页。
示例代码
from selenium import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get('http://example.com')
title = driver.find_element(By.TAG_NAME, 'title').text
print('Title:', title)
paragraphs = driver.find_elements(By.TAG_NAME, 'p')
for p in paragraphs:
print('Paragraph:', p.text)
driver.quit()
四、处理动态网页
在许多情况下,网页内容需要通过JavaScript渲染。这时,使用Selenium这样的工具可以模拟用户操作,等待页面完全加载后再抓取内容。
1. 等待页面加载
Selenium提供了多种方法来等待页面加载完成,例如显式等待和隐式等待。
显式等待
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
driver.get('http://example.com')
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.TAG_NAME, 'p'))
)
print('Paragraph:', element.text)
隐式等待
driver.implicitly_wait(10)
driver.get('http://example.com')
paragraph = driver.find_element(By.TAG_NAME, 'p')
print('Paragraph:', paragraph.text)
2. 处理异步加载内容
许多现代网页使用异步加载技术,例如AJAX。这时,可以通过抓取网络请求的返回数据来获取内容。
使用Selenium抓取AJAX内容
from selenium import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get('http://example.com')
点击加载更多按钮
load_more_button = driver.find_element(By.ID, 'load-more')
load_more_button.click()
等待新内容加载
driver.implicitly_wait(10)
new_content = driver.find_element(By.CLASS_NAME, 'new-content')
print('New Content:', new_content.text)
driver.quit()
五、优化代码性能
在抓取大量数据时,优化代码性能可以显著提高效率。以下是一些优化技巧:
1. 使用多线程或多进程
Python的多线程和多进程库可以显著提高抓取速度,特别是在处理I/O密集型任务时。
多线程示例
import threading
import requests
from bs4 import BeautifulSoup
def fetch_content(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'lxml')
print('Title:', soup.title.string)
urls = ['http://example.com/page1', 'http://example.com/page2', 'http://example.com/page3']
threads = [threading.Thread(target=fetch_content, args=(url,)) for url in urls]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
多进程示例
from multiprocessing import Pool
import requests
from bs4 import BeautifulSoup
def fetch_content(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'lxml')
return soup.title.string
urls = ['http://example.com/page1', 'http://example.com/page2', 'http://example.com/page3']
with Pool(4) as p:
titles = p.map(fetch_content, urls)
for title in titles:
print('Title:', title)
2. 使用缓存
缓存可以避免重复抓取相同的内容,从而提高效率。可以使用requests-cache库来实现缓存。
安装requests-cache
pip install requests-cache
使用requests-cache
import requests
import requests_cache
requests_cache.install_cache('example_cache')
response = requests.get('http://example.com')
print(response.from_cache) # True if the response is from cache
print(response.text)
3. 合理设置抓取间隔
为了避免被目标网站封禁,应合理设置抓取间隔,模拟人类用户的访问行为。
设置抓取间隔
import time
import requests
urls = ['http://example.com/page1', 'http://example.com/page2', 'http://example.com/page3']
for url in urls:
response = requests.get(url)
print(response.text)
time.sleep(2) # 等待2秒
通过以上步骤,你可以高效地使用Python抓取网页中的文字元素。选择合适的工具、理解HTML结构、编写抓取代码、处理动态网页和优化代码性能是成功抓取的关键。希望这些内容能帮助你在实际项目中获得所需的数据。
相关问答FAQs:
如何使用Python获取网页中的特定文本元素?
要获取网页中的特定文本元素,您可以使用Python的Beautiful Soup库。首先,您需要安装requests和beautifulsoup4库。通过requests库获取网页HTML内容,然后使用Beautiful Soup解析HTML。您可以使用查找方法如find()
或find_all()
来定位特定的文本元素,如标题、段落或其他标签。
获取网页元素时需要注意哪些问题?
在获取网页元素时,您需要注意网页的结构和可能的动态内容。某些网页使用JavaScript加载内容,此时可能需要使用Selenium等工具来模拟浏览器行为。此外,注意遵循robots.txt文件中的爬虫协议,以避免违反网站的爬虫政策。
如何处理获取到的网页文本数据?
获取到的网页文本数据可以进行进一步处理,如清洗和分析。您可以使用Python的字符串处理方法或正则表达式来清理文本,例如去除多余的空格、标签和特殊字符。同时,您还可以使用pandas库将文本数据存储在数据框中,以便进行更深入的分析和可视化。
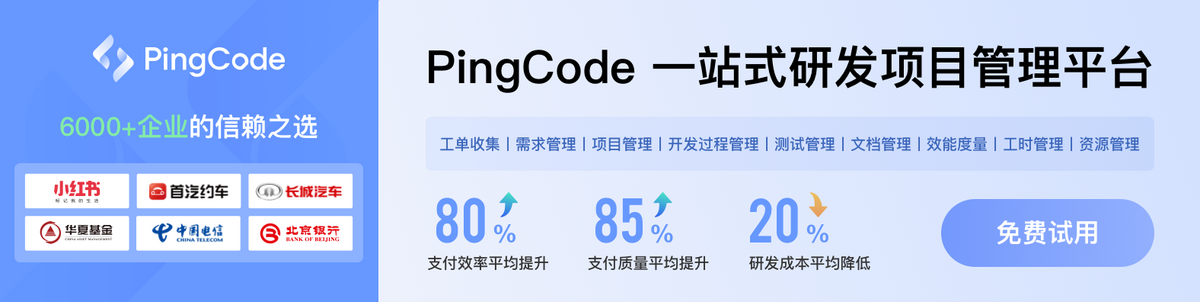