如何在Python上画三角形
在Python上画三角形的方法有很多,使用绘图库如matplotlib、使用pygame进行游戏开发、通过turtle模块进行简单绘图。其中,turtle模块是初学者最常用的方法,因为它提供了简单的绘图API,非常适合用来学习基本的编程和图形绘制。在这篇文章中,我们将详细讨论如何使用这些方法在Python上画三角形。
一、使用matplotlib绘制三角形
1. 安装和导入matplotlib
首先,你需要确保已经安装了matplotlib库。你可以使用以下命令来安装:
pip install matplotlib
安装完成后,可以通过以下代码导入matplotlib:
import matplotlib.pyplot as plt
import numpy as np
2. 绘制三角形
下面是一个使用matplotlib绘制三角形的示例代码:
# Define the vertices of the triangle
triangle = np.array([[1, 1], [2, 3], [3, 1]])
Create a new figure
plt.figure()
Plot the triangle
plt.plot(*triangle.T, marker='o')
Close the triangle by connecting the last point to the first
plt.plot([triangle[0][0], triangle[-1][0]], [triangle[0][1], triangle[-1][1]])
Set the aspect of the plot to be equal
plt.gca().set_aspect('equal', adjustable='box')
Show the plot
plt.show()
在这段代码中,我们首先定义了三角形的顶点坐标,然后使用plt.plot
函数绘制三角形的边。为了确保三角形闭合,我们再次绘制第一点和最后一点之间的边。
二、使用pygame绘制三角形
1. 安装和导入pygame
如果你打算使用pygame进行更高级的绘图和游戏开发,首先需要安装pygame库:
pip install pygame
安装完成后,可以通过以下代码导入pygame:
import pygame
import sys
from pygame.locals import QUIT
2. 初始化和绘制三角形
下面是一个使用pygame绘制三角形的示例代码:
# Initialize pygame
pygame.init()
Set up the display
screen = pygame.display.set_mode((400, 300))
Define the vertices of the triangle
triangle = [(100, 100), (200, 50), (300, 100)]
Main game loop
while True:
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
# Fill the screen with white color
screen.fill((255, 255, 255))
# Draw the triangle
pygame.draw.polygon(screen, (0, 0, 255), triangle)
# Update the display
pygame.display.flip()
在这段代码中,我们首先初始化了pygame并设置了显示窗口。然后在主循环中,我们使用pygame.draw.polygon
函数来绘制三角形,并不断刷新显示。
三、使用turtle绘制三角形
1. 导入turtle模块
turtle模块是Python内置的绘图库,因此不需要额外安装。可以直接导入:
import turtle
2. 初始化和绘制三角形
下面是一个使用turtle模块绘制三角形的示例代码:
# Initialize turtle
t = turtle.Turtle()
Draw the first side of the triangle
t.forward(100)
t.left(120)
Draw the second side of the triangle
t.forward(100)
t.left(120)
Draw the third side of the triangle
t.forward(100)
t.left(120)
Finish drawing
turtle.done()
在这段代码中,我们使用turtle模块的forward
和left
方法来绘制三角形的三条边,每次转动120度来形成等边三角形。
四、比较三种方法
1. 使用场景
- matplotlib:适合数据可视化和简单的几何图形绘制。
- pygame:适合游戏开发和复杂的图形处理。
- turtle:适合初学者和简单的绘图任务。
2. 优缺点
- matplotlib:功能强大,适合复杂的数据可视化,但对新手来说可能有些复杂。
- pygame:适合实时图形和游戏开发,但需要一定的编程基础。
- turtle:简单易用,适合初学者,但功能相对有限。
五、总结
在Python上画三角形有多种方法,使用matplotlib、使用pygame、通过turtle模块都是可行的选择。每种方法都有其优缺点和适用场景,根据你的需求选择合适的方法是关键。希望这篇文章能帮助你在Python上成功绘制三角形,并激发你进一步探索Python绘图库的兴趣。
相关问答FAQs:
如何在Python中选择合适的库来绘制三角形?
在Python中,绘制三角形可以使用多个库,如Matplotlib、Turtle和Pygame等。Matplotlib非常适合数据可视化和图形绘制,Turtle则更适合初学者和教育用途,Pygame则适合游戏开发。如果你的目标是简单绘图,Matplotlib和Turtle是不错的选择。
在Python中绘制三角形的基本步骤是什么?
要在Python中绘制三角形,首先需要安装相应的库。以Matplotlib为例,你可以使用pip install matplotlib
进行安装。接下来,导入库并使用plot
函数绘制三角形的三个顶点,最后调用show()
函数展示图形。简单的代码示例包括定义三角形的三个坐标点,并将它们连接起来形成三角形。
如何自定义三角形的颜色和样式?
在使用Matplotlib绘制三角形时,可以通过参数来自定义颜色和样式。使用fill()
函数可以填充三角形的颜色,传递颜色名称或十六进制颜色值来实现。可以通过设置linewidth
、linestyle
等参数来调整边界的样式。这样的自定义可以让绘制的三角形更加美观和符合个人的需求。
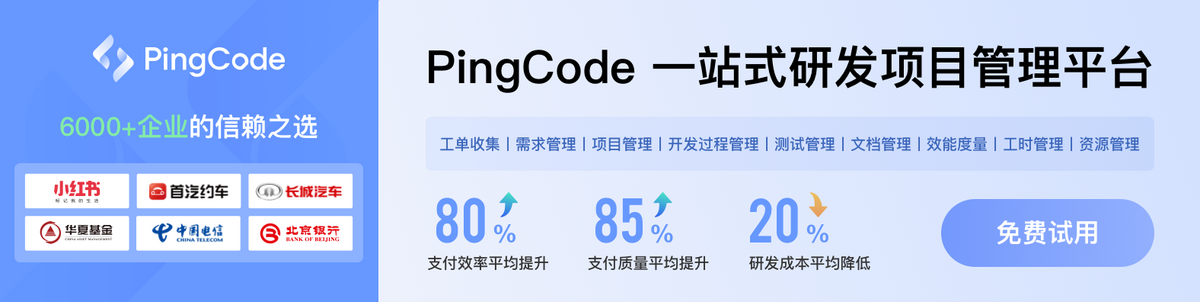