在使用Python大幅增强图片的清晰度时,可以采用多种技术和工具,如使用OpenCV库进行图像处理、利用深度学习模型进行超分辨率重建、以及通过调整对比度和锐化图像等方法。本文将详细介绍这些方法,并提供代码示例来帮助你实现这些目标。
使用OpenCV库、深度学习模型、调整对比度和锐化图像是增强图片清晰度的核心方法。利用深度学习模型进行超分辨率重建,可以显著提升图片的分辨率和细节,下面我们将详细探讨这一点。
超分辨率重建是指从低分辨率图像生成高分辨率图像的过程,这通常需要使用训练好的深度学习模型,如SRCNN(Super-Resolution Convolutional Neural Network)或ESRGAN(Enhanced Super-Resolution Generative Adversarial Networks)。这些模型已经在大规模数据集上进行了预训练,能够生成高质量的高分辨率图像。
接下来,我们将详细介绍这些方法,并提供相应的代码示例。
一、使用OpenCV库进行图像处理
OpenCV(Open Source Computer Vision Library)是一个强大的图像处理库,可以用于各种图像增强任务,如调整对比度和锐化图像。
1. 调整对比度和亮度
调整对比度和亮度可以改善图像的视觉效果,使其更加清晰。以下是使用OpenCV调整对比度和亮度的示例代码:
import cv2
import numpy as np
def adjust_contrast_brightness(image, contrast=1.0, brightness=0):
"""
调整图像的对比度和亮度
:param image: 输入图像
:param contrast: 对比度系数
:param brightness: 亮度增量
:return: 调整后的图像
"""
return cv2.convertScaleAbs(image, alpha=contrast, beta=brightness)
读取图像
image = cv2.imread('input.jpg')
调整对比度和亮度
adjusted_image = adjust_contrast_brightness(image, contrast=1.5, brightness=30)
显示图像
cv2.imshow('Adjusted Image', adjusted_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
2. 图像锐化
图像锐化可以增强图像的边缘,使其更加清晰。以下是使用OpenCV进行图像锐化的示例代码:
import cv2
import numpy as np
def sharpen_image(image):
"""
锐化图像
:param image: 输入图像
:return: 锐化后的图像
"""
kernel = np.array([[0, -1, 0],
[-1, 5,-1],
[0, -1, 0]])
return cv2.filter2D(image, -1, kernel)
读取图像
image = cv2.imread('input.jpg')
锐化图像
sharpened_image = sharpen_image(image)
显示图像
cv2.imshow('Sharpened Image', sharpened_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
二、利用深度学习模型进行超分辨率重建
深度学习模型可以显著提升图像分辨率和细节,常用的模型包括SRCNN和ESRGAN。
1. 使用SRCNN模型进行超分辨率重建
SRCNN(Super-Resolution Convolutional Neural Network)是一个经典的超分辨率模型,可以将低分辨率图像放大并增强细节。以下是使用预训练的SRCNN模型进行超分辨率重建的示例代码:
import cv2
import numpy as np
from keras.models import load_model
def load_srcnn_model(weights_path):
"""
加载预训练的SRCNN模型
:param weights_path: 模型权重文件路径
:return: 加载的模型
"""
model = load_model(weights_path)
return model
def preprocess_image(image, scale):
"""
预处理图像
:param image: 输入图像
:param scale: 缩放因子
:return: 预处理后的图像
"""
height, width, _ = image.shape
image = cv2.resize(image, (width // scale, height // scale), interpolation=cv2.INTER_CUBIC)
image = cv2.resize(image, (width, height), interpolation=cv2.INTER_CUBIC)
image = image.astype(np.float32) / 255.0
return image
def super_resolve_image(model, image):
"""
使用SRCNN模型进行超分辨率重建
:param model: SRCNN模型
:param image: 输入图像
:return: 超分辨率重建后的图像
"""
image = np.expand_dims(image, axis=0)
output = model.predict(image)
output = output[0] * 255.0
output = np.clip(output, 0, 255)
output = output.astype(np.uint8)
return output
读取图像
image = cv2.imread('input.jpg')
预处理图像
preprocessed_image = preprocess_image(image, scale=2)
加载预训练的SRCNN模型
srcnn_model = load_srcnn_model('srcnn_weights.h5')
使用SRCNN模型进行超分辨率重建
super_resolved_image = super_resolve_image(srcnn_model, preprocessed_image)
显示图像
cv2.imshow('Super Resolved Image', super_resolved_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
2. 使用ESRGAN模型进行超分辨率重建
ESRGAN(Enhanced Super-Resolution Generative Adversarial Networks)是一个更先进的超分辨率模型,可以生成更高质量的图像。以下是使用预训练的ESRGAN模型进行超分辨率重建的示例代码:
import cv2
import numpy as np
import torch
from torchvision.transforms import ToTensor, ToPILImage
from PIL import Image
def load_esrgan_model(weights_path):
"""
加载预训练的ESRGAN模型
:param weights_path: 模型权重文件路径
:return: 加载的模型
"""
model = torch.load(weights_path)
model.eval()
return model
def preprocess_image(image):
"""
预处理图像
:param image: 输入图像
:return: 预处理后的图像
"""
image = Image.fromarray(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
image = ToTensor()(image).unsqueeze(0)
return image
def super_resolve_image(model, image):
"""
使用ESRGAN模型进行超分辨率重建
:param model: ESRGAN模型
:param image: 输入图像
:return: 超分辨率重建后的图像
"""
with torch.no_grad():
output = model(image)
output = output.squeeze(0).permute(1, 2, 0).numpy()
output = (output * 255.0).clip(0, 255).astype(np.uint8)
output = cv2.cvtColor(output, cv2.COLOR_RGB2BGR)
return output
读取图像
image = cv2.imread('input.jpg')
预处理图像
preprocessed_image = preprocess_image(image)
加载预训练的ESRGAN模型
esrgan_model = load_esrgan_model('esrgan_weights.pth')
使用ESRGAN模型进行超分辨率重建
super_resolved_image = super_resolve_image(esrgan_model, preprocessed_image)
显示图像
cv2.imshow('Super Resolved Image', super_resolved_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
三、调整图像对比度和锐化
除了使用深度学习模型,调整图像的对比度和锐化也是增强图像清晰度的重要方法。
1. 调整图像对比度
调整对比度可以增加图像的动态范围,使其看起来更加清晰。以下是使用Python调整图像对比度的示例代码:
import cv2
import numpy as np
def adjust_contrast(image, alpha):
"""
调整图像对比度
:param image: 输入图像
:param alpha: 对比度系数
:return: 调整后的图像
"""
adjusted = cv2.convertScaleAbs(image, alpha=alpha)
return adjusted
读取图像
image = cv2.imread('input.jpg')
调整对比度
contrast_image = adjust_contrast(image, alpha=1.5)
显示图像
cv2.imshow('Contrast Image', contrast_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
2. 图像锐化
锐化图像可以增强边缘细节,使图像看起来更清晰。以下是使用Python进行图像锐化的示例代码:
import cv2
import numpy as np
def sharpen_image(image):
"""
锐化图像
:param image: 输入图像
:return: 锐化后的图像
"""
kernel = np.array([[0, -1, 0],
[-1, 5, -1],
[0, -1, 0]])
sharpened = cv2.filter2D(image, -1, kernel)
return sharpened
读取图像
image = cv2.imread('input.jpg')
锐化图像
sharpened_image = sharpen_image(image)
显示图像
cv2.imshow('Sharpened Image', sharpened_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
四、综合应用
在实际应用中,可以综合使用上述方法来大幅增强图像的清晰度。以下是一个综合应用的示例代码:
import cv2
import numpy as np
import torch
from torchvision.transforms import ToTensor, ToPILImage
from PIL import Image
from keras.models import load_model
def load_srcnn_model(weights_path):
model = load_model(weights_path)
return model
def load_esrgan_model(weights_path):
model = torch.load(weights_path)
model.eval()
return model
def preprocess_image(image, scale):
height, width, _ = image.shape
image = cv2.resize(image, (width // scale, height // scale), interpolation=cv2.INTER_CUBIC)
image = cv2.resize(image, (width, height), interpolation=cv2.INTER_CUBIC)
image = image.astype(np.float32) / 255.0
return image
def preprocess_image_esrgan(image):
image = Image.fromarray(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
image = ToTensor()(image).unsqueeze(0)
return image
def adjust_contrast_brightness(image, contrast=1.0, brightness=0):
return cv2.convertScaleAbs(image, alpha=contrast, beta=brightness)
def sharpen_image(image):
kernel = np.array([[0, -1, 0],
[-1, 5, -1],
[0, -1, 0]])
return cv2.filter2D(image, -1, kernel)
def super_resolve_image_srcnn(model, image):
image = np.expand_dims(image, axis=0)
output = model.predict(image)
output = output[0] * 255.0
output = np.clip(output, 0, 255)
output = output.astype(np.uint8)
return output
def super_resolve_image_esrgan(model, image):
with torch.no_grad():
output = model(image)
output = output.squeeze(0).permute(1, 2, 0).numpy()
output = (output * 255.0).clip(0, 255).astype(np.uint8)
output = cv2.cvtColor(output, cv2.COLOR_RGB2BGR)
return output
读取图像
image = cv2.imread('input.jpg')
调整对比度和亮度
adjusted_image = adjust_contrast_brightness(image, contrast=1.5, brightness=30)
锐化图像
sharpened_image = sharpen_image(adjusted_image)
预处理图像
preprocessed_image_srcnn = preprocess_image(sharpened_image, scale=2)
preprocessed_image_esrgan = preprocess_image_esrgan(sharpened_image)
加载预训练的模型
srcnn_model = load_srcnn_model('srcnn_weights.h5')
esrgan_model = load_esrgan_model('esrgan_weights.pth')
使用SRCNN模型进行超分辨率重建
super_resolved_image_srcnn = super_resolve_image_srcnn(srcnn_model, preprocessed_image_srcnn)
使用ESRGAN模型进行超分辨率重建
super_resolved_image_esrgan = super_resolve_image_esrgan(esrgan_model, preprocessed_image_esrgan)
显示图像
cv2.imshow('Super Resolved Image SRCNN', super_resolved_image_srcnn)
cv2.imshow('Super Resolved Image ESRGAN', super_resolved_image_esrgan)
cv2.waitKey(0)
cv2.destroyAllWindows()
通过以上综合应用的方法,可以有效地提升图像的清晰度,使其具有更高的视觉质量。希望本文对你了解和实践如何用Python大幅增强图片的清晰度有所帮助。
相关问答FAQs:
如何使用Python库来增强图片的清晰度?
可以使用多个Python库来增强图片的清晰度,其中最常用的是PIL(Pillow)和OpenCV。Pillow提供了简单的图像处理功能,可以使用滤镜如锐化来改善图像的清晰度。OpenCV则提供了更多复杂的图像处理技术,例如拉普拉斯锐化和高通滤波。根据需求选择合适的库,并利用其相关功能进行清晰度增强。
有哪些常用的图像增强技术可以提高图片质量?
常用的图像增强技术包括锐化、对比度增强、亮度调节和去噪声处理。锐化技术通过强调图像边缘来增加清晰度,对比度增强则通过调整颜色强度来提高视觉效果。亮度调节有助于改善图像的整体观感,而去噪声处理可以消除图像中不必要的噪声,从而使图像更加清晰。
增强图片清晰度的过程中,如何避免图像失真?
在增强图片清晰度时,保持图像质量至关重要。建议使用适度的锐化和对比度调整,以避免图像过度处理而导致的失真。使用高级技术,如自适应直方图均衡化,可以帮助改善图像的细节而不会产生明显的失真。此外,进行图像处理时,要定期查看增强效果,以确保最终结果符合预期。
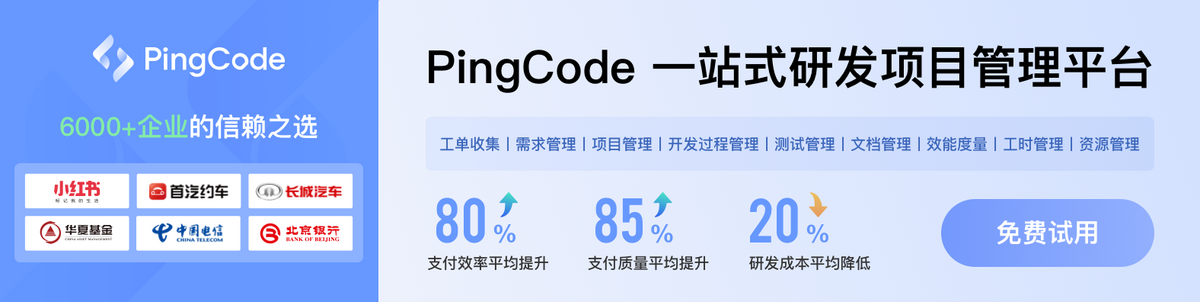