使用Python对文档中的名词进行统计的方法包括:自然语言处理、词性标注、分词、停用词过滤。 其中,自然语言处理是最为核心的一点,它通过计算机算法理解和处理人类语言文本。具体操作包括文本预处理、词性标注、分词和过滤停用词。本文将详细描述如何使用Python的自然语言处理库,如NLTK和spaCy,来实现这一目标。
一、自然语言处理
自然语言处理(NLP)是计算机科学、人工智能和语言学交叉的领域。它的目标是让计算机能够理解和处理人类语言。在用Python对文档中的名词进行统计时,NLP可以帮助我们处理和分析文本数据,使得我们能够从中提取有价值的信息。
NLP的核心步骤包括:文本预处理、词性标注、命名实体识别和情感分析等。我们将在后面的部分详细讨论这些步骤,并展示如何使用Python库来实现它们。
二、文本预处理
文本预处理是NLP的重要步骤,主要包括:去除标点符号、转化为小写、去除停用词和分词等。通过这些操作,我们可以将原始文本转化为更易于处理的形式。
1. 去除标点符号
在处理文本时,标点符号往往不是我们关注的重点,因此需要去除。我们可以使用正则表达式(regex)来实现这一目标。
import re
def remove_punctuation(text):
return re.sub(r'[^\w\s]', '', text)
2. 转化为小写
转化为小写可以帮助我们统一文本格式,避免因大小写不同而导致的重复计算。
def to_lowercase(text):
return text.lower()
3. 去除停用词
停用词是指在文本中频繁出现但没有实际意义的词汇,如“的”、“是”、“了”等。我们可以使用NLTK库中的停用词列表来去除这些词。
from nltk.corpus import stopwords
def remove_stopwords(text):
stop_words = set(stopwords.words('english'))
return ' '.join([word for word in text.split() if word not in stop_words])
4. 分词
分词是将文本拆分为单词的过程。在英文中,分词相对简单,可以直接使用空格进行拆分;在中文中,则需要使用专门的分词工具,如jieba库。
def tokenize(text):
return text.split()
三、词性标注
词性标注是指为每个单词分配一个词性标签,如名词、动词、形容词等。在Python中,我们可以使用NLTK和spaCy库进行词性标注。
1. 使用NLTK进行词性标注
NLTK(Natural Language Toolkit)是一个强大的Python库,提供了丰富的自然语言处理工具。我们可以使用NLTK的pos_tag函数进行词性标注。
import nltk
def pos_tagging(text):
tokens = nltk.word_tokenize(text)
return nltk.pos_tag(tokens)
2. 使用spaCy进行词性标注
spaCy是另一个流行的NLP库,它的性能更高,适用于处理大规模数据。我们可以使用spaCy的模型进行词性标注。
import spacy
def spacy_pos_tagging(text):
nlp = spacy.load('en_core_web_sm')
doc = nlp(text)
return [(token.text, token.pos_) for token in doc]
四、名词提取
在完成词性标注后,我们可以根据词性标签提取名词。在NLTK中,名词的标签通常是NN、NNS、NNP和NNPS;在spaCy中,名词的标签是NOUN和PROPN。
def extract_nouns(tagged_text):
return [word for word, pos in tagged_text if pos in ('NN', 'NNS', 'NNP', 'NNPS')]
五、名词统计
最后,我们可以统计名词的频次。在Python中,我们可以使用collections.Counter类来实现这一目标。
from collections import Counter
def noun_frequency(nouns):
return Counter(nouns)
六、完整示例
下面是一个完整的示例,展示如何使用上述步骤对文档中的名词进行统计。
import re
import nltk
import spacy
from nltk.corpus import stopwords
from collections import Counter
下载NLTK数据
nltk.download('punkt')
nltk.download('averaged_perceptron_tagger')
nltk.download('stopwords')
def remove_punctuation(text):
return re.sub(r'[^\w\s]', '', text)
def to_lowercase(text):
return text.lower()
def remove_stopwords(text):
stop_words = set(stopwords.words('english'))
return ' '.join([word for word in text.split() if word not in stop_words])
def preprocess_text(text):
text = remove_punctuation(text)
text = to_lowercase(text)
text = remove_stopwords(text)
return text
def pos_tagging(text):
tokens = nltk.word_tokenize(text)
return nltk.pos_tag(tokens)
def extract_nouns(tagged_text):
return [word for word, pos in tagged_text if pos in ('NN', 'NNS', 'NNP', 'NNPS')]
def noun_frequency(nouns):
return Counter(nouns)
def main(text):
preprocessed_text = preprocess_text(text)
tagged_text = pos_tagging(preprocessed_text)
nouns = extract_nouns(tagged_text)
freq = noun_frequency(nouns)
return freq
示例文本
text = "Python is a popular programming language. It is widely used in data science, machine learning, and web development."
统计名词频次
freq = main(text)
print(freq)
七、使用spaCy进行优化
虽然NLTK功能强大,但spaCy在处理大规模数据时表现更佳。我们可以使用spaCy替代NLTK,重写上述示例。
import re
import spacy
from nltk.corpus import stopwords
from collections import Counter
加载spaCy模型
nlp = spacy.load('en_core_web_sm')
def remove_punctuation(text):
return re.sub(r'[^\w\s]', '', text)
def to_lowercase(text):
return text.lower()
def remove_stopwords(text):
stop_words = set(stopwords.words('english'))
return ' '.join([word for word in text.split() if word not in stop_words])
def preprocess_text(text):
text = remove_punctuation(text)
text = to_lowercase(text)
text = remove_stopwords(text)
return text
def spacy_pos_tagging(text):
doc = nlp(text)
return [(token.text, token.pos_) for token in doc]
def extract_nouns(tagged_text):
return [word for word, pos in tagged_text if pos in ('NOUN', 'PROPN')]
def noun_frequency(nouns):
return Counter(nouns)
def main(text):
preprocessed_text = preprocess_text(text)
tagged_text = spacy_pos_tagging(preprocessed_text)
nouns = extract_nouns(tagged_text)
freq = noun_frequency(nouns)
return freq
示例文本
text = "Python is a popular programming language. It is widely used in data science, machine learning, and web development."
统计名词频次
freq = main(text)
print(freq)
八、进一步优化和应用
1. 处理多语言文本
如果需要处理非英文文本,我们可以使用对应语言的模型。例如,spaCy提供了多种语言的模型,可以处理中文、法语、德语等文本。
# 加载中文模型
nlp = spacy.load('zh_core_web_sm')
def main(text):
preprocessed_text = preprocess_text(text)
tagged_text = spacy_pos_tagging(preprocessed_text)
nouns = extract_nouns(tagged_text)
freq = noun_frequency(nouns)
return freq
示例中文文本
text = "Python是一种流行的编程语言。它广泛应用于数据科学、机器学习和Web开发。"
统计名词频次
freq = main(text)
print(freq)
2. 处理大规模文本数据
在处理大规模文本数据时,我们可以使用分布式计算框架,如Apache Spark,来提高处理效率。PySpark是Spark的Python API,可以帮助我们实现这一目标。
from pyspark.sql import SparkSession
from pyspark.sql.functions import col, explode, split
初始化SparkSession
spark = SparkSession.builder.appName('NounFrequency').getOrCreate()
def preprocess_text(text):
text = remove_punctuation(text)
text = to_lowercase(text)
text = remove_stopwords(text)
return text
def main(texts):
# 转化为DataFrame
df = spark.createDataFrame([(text,) for text in texts], ['text'])
# 预处理文本
preprocess_udf = spark.udf.register('preprocess_text', preprocess_text)
df = df.withColumn('preprocessed_text', preprocess_udf(col('text')))
# 分词
df = df.withColumn('tokens', split(col('preprocessed_text'), ' '))
# 词性标注和名词提取
tagged_udf = spark.udf.register('spacy_pos_tagging', spacy_pos_tagging)
df = df.withColumn('tagged', tagged_udf(col('preprocessed_text')))
extract_udf = spark.udf.register('extract_nouns', extract_nouns)
df = df.withColumn('nouns', extract_udf(col('tagged')))
# 统计名词频次
df = df.withColumn('noun', explode(col('nouns')))
freq_df = df.groupBy('noun').count().orderBy('count', ascending=False)
return freq_df
示例文本
texts = [
"Python is a popular programming language.",
"It is widely used in data science, machine learning, and web development."
]
统计名词频次
freq_df = main(texts)
freq_df.show()
九、总结
使用Python对文档中的名词进行统计是一个复杂但有趣的任务。本文详细介绍了从文本预处理、词性标注到名词提取和统计的完整流程。我们使用了NLTK和spaCy两种流行的NLP库,并展示了如何优化和应用这些方法。通过这些步骤,我们可以轻松地从文本数据中提取有价值的信息。希望本文能为您在自然语言处理领域的研究和应用提供一些帮助。
相关问答FAQs:
如何使用Python提取文档中的名词?
要提取文档中的名词,可以使用自然语言处理库,如NLTK或spaCy。这些库提供了强大的工具,可以对文本进行分词和词性标注。通过识别词性为名词的单词,就可以完成名词的提取。
使用Python统计名词时,有哪些常用的库和工具?
常用的库包括NLTK、spaCy和TextBlob。NLTK提供了基本的文本处理功能,spaCy则在速度和准确性上表现更佳,特别是在处理大型文本时。TextBlob则是一个简单易用的库,适合快速开发和测试。
如何优化名词统计的结果,以便于分析?
对名词进行统计后,可以使用数据可视化库如Matplotlib或Seaborn,生成柱状图或词云,帮助直观展示名词的频率。此外,可以对名词进行去重、分类和排序,以便更好地理解文本内容。
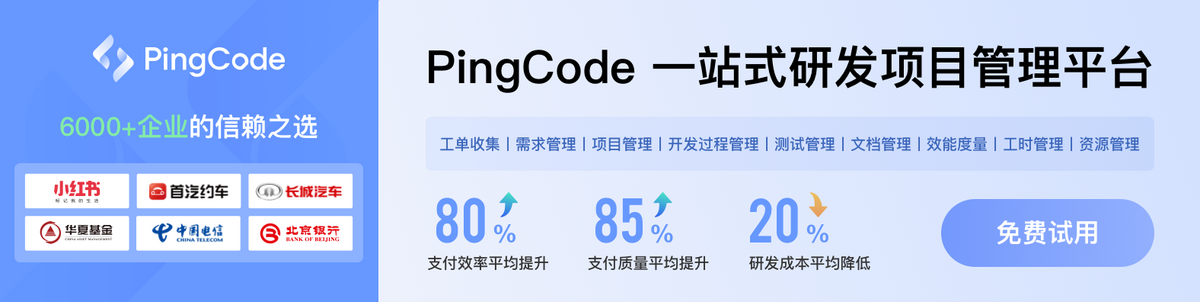