Python在爬取10万级别的数据时,最关键的是合理使用并发处理、优化爬取策略、有效管理数据存储和确保遵守网站的爬取规则。 本文将详细探讨这些关键点,具体包括如何使用Python的多线程和多进程库、如何设计爬虫策略、数据存储优化以及遵守网站爬取规则的重要性。
一、并发处理:多线程与多进程
为了爬取大规模数据,提升爬取速度是关键。Python提供了多线程和多进程两种并发处理方式。
1.1 多线程
多线程适用于I/O密集型任务,比如网络请求。Python的threading
模块可以实现多线程爬取。
import threading
import requests
def fetch_url(url):
response = requests.get(url)
# 处理响应
urls = ['http://example.com/page1', 'http://example.com/page2', ...]
threads = []
for url in urls:
thread = threading.Thread(target=fetch_url, args=(url,))
thread.start()
threads.append(thread)
for thread in threads:
thread.join()
1.2 多进程
多进程适用于CPU密集型任务,比如数据处理。Python的multiprocessing
模块可以实现多进程爬取。
import multiprocessing
import requests
def fetch_url(url):
response = requests.get(url)
# 处理响应
urls = ['http://example.com/page1', 'http://example.com/page2', ...]
with multiprocessing.Pool(processes=4) as pool:
pool.map(fetch_url, urls)
二、设计爬虫策略
2.1 分布式爬虫
对于大规模数据爬取,分布式爬虫是一种有效的解决方案。Scrapy-Redis是一个流行的分布式爬虫框架。
from scrapy_redis.spiders import RedisSpider
class MySpider(RedisSpider):
name = 'myspider'
redis_key = 'myspider:start_urls'
def parse(self, response):
# 解析响应
2.2 爬取间隔与速率限制
为了避免被网站封禁,需合理设置爬取间隔和速率限制。
import time
import requests
def fetch_url(url):
response = requests.get(url)
# 处理响应
time.sleep(1) # 设置爬取间隔
urls = ['http://example.com/page1', 'http://example.com/page2', ...]
for url in urls:
fetch_url(url)
三、数据存储优化
3.1 使用数据库
对于大规模数据,使用数据库存储是必要的。MongoDB、MySQL等数据库都可以用来存储爬取的数据。
import pymongo
client = pymongo.MongoClient('localhost', 27017)
db = client['mydatabase']
collection = db['mycollection']
def store_data(data):
collection.insert_one(data)
3.2 数据清洗与预处理
在存储数据之前,进行数据清洗和预处理是必要的,以保证数据质量。
def clean_data(data):
# 清洗和预处理数据
return data
def fetch_url(url):
response = requests.get(url)
data = response.json()
clean_data = clean_data(data)
store_data(clean_data)
四、遵守网站爬取规则
4.1 Robots.txt
在开始爬取之前,需检查目标网站的robots.txt
文件,确保爬取行为符合网站的规定。
import requests
def check_robots_txt(url):
robots_url = url + '/robots.txt'
response = requests.get(robots_url)
# 解析robots.txt文件
4.2 反爬机制
为了绕过网站的反爬机制,可以使用代理、伪造User-Agent等技术。
import requests
headers = {'User-Agent': 'Mozilla/5.0'}
proxies = {'http': 'http://10.10.1.10:3128'}
response = requests.get('http://example.com', headers=headers, proxies=proxies)
五、错误处理与重试机制
5.1 错误处理
在爬取过程中,可能会遇到各种错误,如网络错误、超时等。需进行错误处理,确保爬虫的稳定性。
import requests
from requests.exceptions import RequestException
def fetch_url(url):
try:
response = requests.get(url)
response.raise_for_status()
except RequestException as e:
print(f"Error fetching {url}: {e}")
5.2 重试机制
对于临时性错误,设置重试机制是必要的,确保数据的完整性。
import requests
from requests.adapters import HTTPAdapter
from requests.packages.urllib3.util.retry import Retry
session = requests.Session()
retry = Retry(total=5, backoff_factor=1)
adapter = HTTPAdapter(max_retries=retry)
session.mount('http://', adapter)
session.mount('https://', adapter)
response = session.get('http://example.com')
六、实战案例:爬取10万级别的数据
6.1 确定目标网站与数据
首先,确定要爬取的网站和数据。例如,爬取某电商网站的商品信息。
6.2 编写爬虫代码
根据前面介绍的多线程、多进程、分布式爬虫等方法,编写爬虫代码。
import requests
import threading
def fetch_product_data(url):
response = requests.get(url)
data = response.json()
# 数据处理与存储
product_urls = ['http://example.com/product1', 'http://example.com/product2', ...]
threads = []
for url in product_urls:
thread = threading.Thread(target=fetch_product_data, args=(url,))
thread.start()
threads.append(thread)
for thread in threads:
thread.join()
6.3 数据存储与管理
将爬取的数据存储到数据库中,进行管理和分析。
import pymongo
client = pymongo.MongoClient('localhost', 27017)
db = client['ecommerce']
collection = db['products']
def store_product_data(data):
collection.insert_one(data)
6.4 优化与扩展
根据实际情况,不断优化爬虫代码,提升效率。同时,可以扩展爬取更多的数据。
总结
爬取10万级别的数据是一项复杂的任务,需要合理使用并发处理、设计高效的爬虫策略、优化数据存储以及遵守网站的爬取规则。通过本文介绍的方法和案例,相信你已经掌握了如何使用Python进行大规模数据爬取的技巧。希望这些内容对你的实际工作有所帮助。
相关问答FAQs:
在使用Python爬取10万级别的数据时,我需要考虑哪些技术和库?
在爬取大量数据时,选择合适的技术和库至关重要。常用的Python库包括Requests和BeautifulSoup用于发起HTTP请求和解析HTML文档,Scrapy框架则是一个功能强大的爬虫工具,适合于大规模数据抓取。对于需要处理JavaScript渲染的网页,可以使用Selenium或Playwright等工具。此外,使用代理服务和设置请求延迟可以帮助规避反爬虫机制。
如何管理和存储爬取到的10万级别数据?
在爬取大量数据后,数据的管理和存储显得尤为重要。可以选择将数据存储在CSV文件中,便于后续分析。如果需要更复杂的查询和数据管理,使用数据库(如MySQL、PostgreSQL或MongoDB)是一个不错的选择。对于大规模数据,考虑使用数据仓库或云存储服务,以便于扩展和管理。
爬取10万级别数据时,如何处理爬虫被封IP的问题?
在大规模爬取数据时,IP被封锁是常见问题。为避免这种情况,可以采用多种策略。使用代理池可以让请求通过不同的IP地址发送,分散请求压力。此外,设置合理的请求间隔和遵循网站的robots.txt协议也非常重要。定期监控爬取的效果,及时调整策略,以确保数据抓取的持续性和有效性。
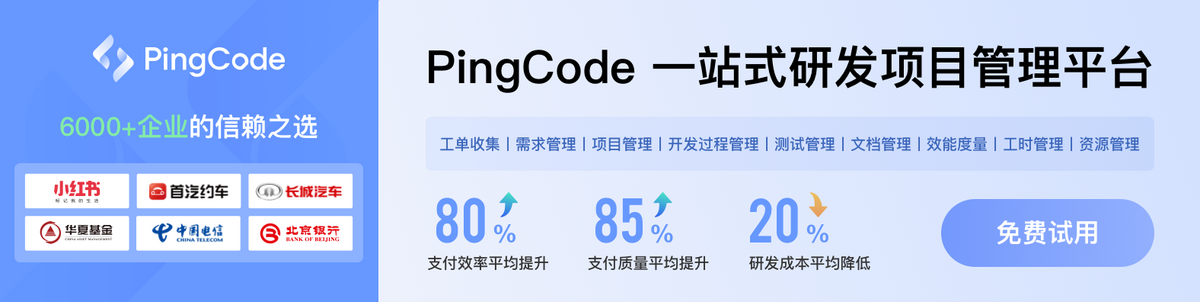