Python进行动态字符串比较的方法有多种,包括使用内置函数、正则表达式、第三方库等。核心方法包括:字符串直接比较、正则表达式匹配、Levenshtein距离算法、SequenceMatcher类。在这里,我们将重点讨论如何使用内置函数和正则表达式来进行动态字符串比较。
一、字符串直接比较
Python提供了简单且高效的字符串比较方法,可以直接使用==
运算符进行比较。这种方法适用于比较确定的、完全相同的字符串。
str1 = "hello"
str2 = "hello"
if str1 == str2:
print("The strings are equal.")
else:
print("The strings are not equal.")
这种方法的优点是简单明了,缺点是只能判断字符串是否完全相同,无法处理复杂的比较场景。
二、使用正则表达式进行字符串比较
正则表达式(Regular Expressions,简称regex)是一个强大的工具,用于匹配字符串中的复杂模式。Python的re
模块提供了丰富的正则表达式支持,可以在动态字符串比较中大显身手。
1、基本使用
首先,需要导入re
模块,然后使用re.match
或re.search
函数进行模式匹配。
import re
pattern = r"hello"
text = "hello world"
if re.match(pattern, text):
print("Pattern found in the text.")
else:
print("Pattern not found in the text.")
在上述例子中,re.match
函数用于检查文本是否以指定的模式开头。如果需要在整个文本中查找模式,可以使用re.search
函数。
2、使用正则表达式进行复杂匹配
正则表达式不仅可以匹配简单的字符串,还可以进行更复杂的模式匹配。例如,匹配一个字符串中所有的数字:
import re
pattern = r"\d+"
text = "The price is 100 dollars."
matches = re.findall(pattern, text)
print("Found numbers:", matches)
在这个例子中,\d+
表示匹配一个或多个数字字符,re.findall
函数返回所有匹配的结果。
3、动态生成正则表达式
在实际应用中,可能需要根据不同的条件动态生成正则表达式。可以使用Python的字符串格式化功能来实现这一点:
import re
def generate_pattern(word):
return fr"{word}\d*"
text = "hello123"
pattern = generate_pattern("hello")
if re.match(pattern, text):
print("Pattern found in the text.")
else:
print("Pattern not found in the text.")
通过这种方式,可以根据输入动态生成正则表达式,从而实现更灵活的字符串比较。
三、Levenshtein距离算法
Levenshtein距离(也称为编辑距离)是指两个字符串之间,通过插入、删除或替换单个字符将一个字符串变成另一个字符串所需的最少编辑操作次数。它是一个衡量字符串相似度的重要指标。
1、计算Levenshtein距离
Python中可以使用Levenshtein
库来计算两个字符串的Levenshtein距离。首先需要安装该库:
pip install python-Levenshtein
然后可以使用以下代码进行计算:
import Levenshtein
str1 = "kitten"
str2 = "sitting"
distance = Levenshtein.distance(str1, str2)
print("Levenshtein distance:", distance)
在这个例子中,Levenshtein距离为3,因为需要进行3次编辑操作(替换、插入、插入)才能将kitten
变成sitting
。
2、应用场景
Levenshtein距离广泛应用于拼写检查、DNA序列比对、自然语言处理等领域。例如,在拼写检查中,可以将用户输入的单词与词典中的单词进行比较,找出Levenshtein距离最小的单词作为推荐。
import Levenshtein
def correct_spelling(word, dictionary):
closest_word = min(dictionary, key=lambda w: Levenshtein.distance(word, w))
return closest_word
dictionary = ["apple", "banana", "orange", "grape"]
word = "appl"
corrected_word = correct_spelling(word, dictionary)
print("Did you mean:", corrected_word)
在这个例子中,输入的单词appl
被纠正为apple
。
四、SequenceMatcher类
Python的difflib
模块提供了SequenceMatcher
类,用于比较两个序列(包括字符串)的相似度。它可以找出两个序列的最长公共子序列,并计算相似度比率。
1、基本使用
可以使用SequenceMatcher
类来比较两个字符串的相似度:
from difflib import SequenceMatcher
str1 = "hello world"
str2 = "hello"
matcher = SequenceMatcher(None, str1, str2)
ratio = matcher.ratio()
print("Similarity ratio:", ratio)
在这个例子中,SequenceMatcher
计算两个字符串的相似度比率,结果为0.8333,表示两个字符串有83.33%的相似度。
2、查找最长公共子序列
SequenceMatcher
类还可以用于查找两个字符串的最长公共子序列:
from difflib import SequenceMatcher
str1 = "hello world"
str2 = "world hello"
matcher = SequenceMatcher(None, str1, str2)
match = matcher.find_longest_match(0, len(str1), 0, len(str2))
print("Longest common substring:", str1[match.a: match.a + match.size])
在这个例子中,最长的公共子序列是" "
, 表示两个字符串的最长公共子序列为空格。
3、应用场景
SequenceMatcher
类广泛应用于文本比较、版本控制系统、数据去重等场景。例如,在版本控制系统中,可以使用SequenceMatcher
类来比较两个版本的差异,从而实现增量更新。
from difflib import SequenceMatcher
def get_diff(text1, text2):
matcher = SequenceMatcher(None, text1, text2)
for tag, i1, i2, j1, j2 in matcher.get_opcodes():
if tag == 'replace':
print(f"Replace {text1[i1:i2]} with {text2[j1:j2]}")
elif tag == 'delete':
print(f"Delete {text1[i1:i2]}")
elif tag == 'insert':
print(f"Insert {text2[j1:j2]}")
elif tag == 'equal':
print(f"Equal {text1[i1:i2]}")
text1 = "hello world"
text2 = "hello python world"
get_diff(text1, text2)
在这个例子中,get_diff
函数使用SequenceMatcher
类找出两个文本的差异,并输出详细的差异信息。
通过以上几种方法,Python可以高效地进行动态字符串比较。选择适合的方法取决于具体的应用场景和需求。
相关问答FAQs:
在Python中,如何实现动态字符串比较的功能?
动态字符串比较可以通过使用Python的内置字符串方法和比较运算符来实现。您可以根据需要动态构建字符串,然后使用==
、!=
、<
、>
等运算符进行比较。此外,您还可以使用str()
函数将其他数据类型转换为字符串,以便进行比较。例如,可以通过拼接字符串或使用格式化字符串来创建动态内容,然后进行比较操作。
如何处理字符串比较时的大小写问题?
字符串比较时,大小写可能会影响结果。为了避免这种情况,您可以使用str.lower()
或str.upper()
方法将字符串转换为统一的大小写后再进行比较。这种方式确保了在进行动态字符串比较时,不会因为大小写的不同而导致错误的结果。
在动态比较字符串时,如何提高性能?
为了提高动态字符串比较的性能,可以考虑一些优化策略。使用set
或dict
等数据结构,可以快速查找或比较多个字符串。此外,避免在循环中频繁创建字符串,尽量使用缓存或预先处理好的字符串,以减少内存开销和提高效率。使用join()
方法来连接多个字符串也会比使用+
运算符更高效。
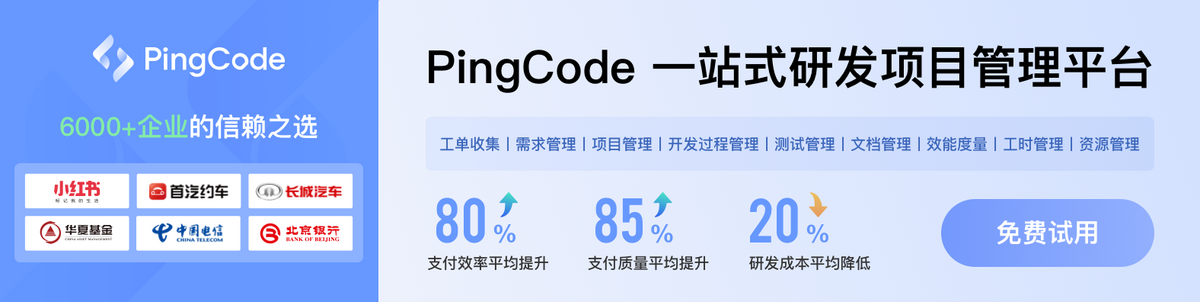