要用Python爬虫爬取一个网页,你需要掌握以下几个核心步骤:选择合适的工具和库、发送HTTP请求、解析网页内容、处理数据、遵守法律和道德规范。其中,选择合适的工具和库是至关重要的一步,因为不同的库有不同的优势和适用场景。下面我将详细介绍这些步骤。
一、选择合适的工具和库
Python 提供了丰富的库来支持网页爬取,其中最常用的是 Requests 和 BeautifulSoup。Requests 是一个用于发送HTTP请求的库,使用起来非常简单和直观;BeautifulSoup 是一个用于解析HTML和XML文档的库,可以轻松地从网页中提取数据。
Requests库
Requests 是一个非常流行且易于使用的HTTP库,能够处理复杂的HTTP请求并返回响应。它的主要优点在于其简洁的API和强大的功能。
import requests
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
print('Request successful!')
print(response.text)
else:
print(f'Failed to retrieve the webpage. Status code: {response.status_code}')
BeautifulSoup库
BeautifulSoup 是一个用于解析HTML和XML文档的库,可以轻松地从网页中提取数据。它支持多种解析器,其中最常用的是 html.parser 和 lxml。
from bs4 import BeautifulSoup
html_doc = """
<html><head><title>The Dormouse's story</title></head>
<body><p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
"""
soup = BeautifulSoup(html_doc, 'html.parser')
print(soup.prettify())
二、发送HTTP请求
发送HTTP请求是爬取网页的第一步。你需要通过HTTP请求获取网页的HTML内容。常见的请求方法包括GET和POST,GET方法用于请求数据,POST方法用于提交数据。
发送GET请求
使用 Requests 库发送GET请求非常简单,只需要指定目标URL即可。
import requests
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
print('Request successful!')
print(response.text)
else:
print(f'Failed to retrieve the webpage. Status code: {response.status_code}')
发送POST请求
有时你需要通过POST请求提交数据,例如登录表单。使用 Requests 库发送POST请求同样很简单,只需要将数据作为字典传递给 requests.post() 方法。
import requests
url = 'http://example.com/login'
data = {'username': 'myusername', 'password': 'mypassword'}
response = requests.post(url, data=data)
if response.status_code == 200:
print('Login successful!')
print(response.text)
else:
print(f'Failed to login. Status code: {response.status_code}')
三、解析网页内容
解析网页内容是爬取网页的核心步骤。你需要从HTML文档中提取所需的数据。BeautifulSoup 是一个非常强大的工具,可以轻松地从HTML文档中提取数据。
解析HTML文档
使用 BeautifulSoup 解析HTML文档非常简单,只需要将HTML文档传递给 BeautifulSoup 构造函数即可。
from bs4 import BeautifulSoup
html_doc = """
<html><head><title>The Dormouse's story</title></head>
<body><p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
"""
soup = BeautifulSoup(html_doc, 'html.parser')
print(soup.prettify())
查找元素
BeautifulSoup 提供了多种方法来查找HTML文档中的元素,例如 find() 和 find_all() 方法。
# 查找单个元素
title_tag = soup.find('title')
print(title_tag.string)
查找所有元素
links = soup.find_all('a')
for link in links:
print(link.get('href'))
四、处理数据
处理数据是爬取网页的最终目的。你需要将提取的数据保存到数据库或文件中,以便后续分析和处理。Python 提供了丰富的库来支持数据处理和存储,例如 pandas 和 sqlite3。
保存数据到CSV文件
使用 pandas 库可以轻松地将数据保存到CSV文件中。
import pandas as pd
data = {'name': ['Elsie', 'Lacie', 'Tillie'], 'age': [25, 30, 35]}
df = pd.DataFrame(data)
df.to_csv('data.csv', index=False)
保存数据到SQLite数据库
使用 sqlite3 库可以轻松地将数据保存到SQLite数据库中。
import sqlite3
conn = sqlite3.connect('data.db')
c = conn.cursor()
创建表
c.execute('''CREATE TABLE IF NOT EXISTS users
(name TEXT, age INTEGER)''')
插入数据
users = [('Elsie', 25), ('Lacie', 30), ('Tillie', 35)]
c.executemany('INSERT INTO users VALUES (?, ?)', users)
提交事务
conn.commit()
查询数据
c.execute('SELECT * FROM users')
print(c.fetchall())
关闭连接
conn.close()
五、遵守法律和道德规范
在爬取网页时,遵守法律和道德规范是非常重要的。未经授权的爬虫行为可能会违反网站的服务条款,甚至触犯法律。以下是一些遵守法律和道德规范的建议:
遵守网站的Robots.txt文件
Robots.txt 文件是网站用于向爬虫说明哪些页面可以访问,哪些页面不能访问的协议。在爬取网页前,应该先检查网站的 Robots.txt 文件,并遵守其中的规则。
import requests
url = 'http://example.com/robots.txt'
response = requests.get(url)
if response.status_code == 200:
print('Robots.txt content:')
print(response.text)
else:
print(f'Failed to retrieve Robots.txt. Status code: {response.status_code}')
设置适当的请求间隔
为了避免给目标网站带来过大的负担,应该设置适当的请求间隔。使用 time.sleep() 方法可以轻松地实现请求间隔。
import time
import requests
urls = ['http://example.com/page1', 'http://example.com/page2', 'http://example.com/page3']
for url in urls:
response = requests.get(url)
if response.status_code == 200:
print(f'Successfully retrieved {url}')
else:
print(f'Failed to retrieve {url}. Status code: {response.status_code}')
# 设置请求间隔
time.sleep(5)
识别并处理反爬虫机制
许多网站都有反爬虫机制,例如IP封禁和验证码。为了避免被封禁,可以使用代理池和模拟浏览器行为。
import requests
from fake_useragent import UserAgent
使用代理池
proxies = {
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080'
}
模拟浏览器行为
ua = UserAgent()
headers = {'User-Agent': ua.random}
response = requests.get('http://example.com', headers=headers, proxies=proxies)
if response.status_code == 200:
print('Request successful!')
print(response.text)
else:
print(f'Failed to retrieve the webpage. Status code: {response.status_code}')
总结
通过选择合适的工具和库、发送HTTP请求、解析网页内容、处理数据以及遵守法律和道德规范,你可以使用Python爬虫高效地爬取网页。希望这篇文章对你有所帮助,祝你在爬虫的学习和应用中取得成功。
相关问答FAQs:
如何选择合适的Python库来进行网页爬取?
在Python中,有多个库可供选择,像BeautifulSoup、Requests和Scrapy等。Requests库非常适合发送HTTP请求并获取网页内容,而BeautifulSoup则可以帮助解析HTML文档并提取所需的信息。Scrapy是一个功能强大的框架,适合需要处理大量数据的复杂项目。选择合适的库主要取决于项目的需求和复杂性。
在爬取网页时应该注意哪些法律和道德规范?
进行网页爬取时,遵守网站的robots.txt文件规则是非常重要的,这个文件通常会规定哪些内容可以被爬取,哪些内容是禁止的。此外,避免对网站造成过大压力,尽量控制请求频率,使用适当的延迟,确保不会对服务器造成负担。同时,尊重版权和数据隐私,确保爬取的数据不会被用于不当用途。
如何处理爬取到的网页数据?
一旦成功爬取到网页内容,数据处理就成为一个重要步骤。可以使用Pandas库将数据转化为DataFrame格式,便于进一步分析和处理。同时,使用正则表达式或BeautifulSoup提取所需信息后,可以将清洗后的数据存储到CSV文件、数据库或其他格式,方便后续使用和分析。
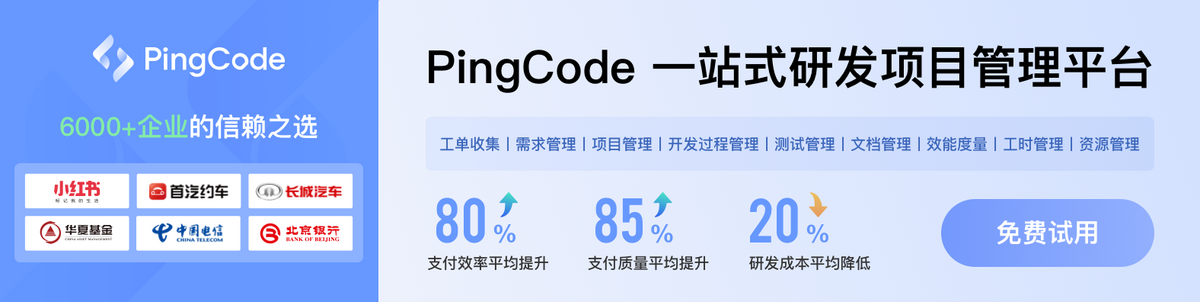