删除数据库中的第一列通常是一个需要谨慎处理的操作,主要步骤包括:连接数据库、获取表信息、删除列、更新表结构。 其中,获取表信息是最关键的一步,因为需要确保不会破坏表的完整性和数据一致性。下面将详细介绍如何使用Python删除数据库的第一列。
一、连接数据库
在开始删除列之前,首先需要连接到数据库。Python中最常用的数据库连接库是sqlite3
、MySQL Connector
和psycopg2
等。以下示例展示了如何使用这些库连接到数据库。
1.1 使用 sqlite3 连接 SQLite 数据库
import sqlite3
def connect_to_sqlite(db_name):
try:
conn = sqlite3.connect(db_name)
return conn
except sqlite3.Error as e:
print(f"Error connecting to database: {e}")
return None
1.2 使用 MySQL Connector 连接 MySQL 数据库
import mysql.connector
def connect_to_mysql(user, password, host, database):
try:
conn = mysql.connector.connect(
user=user,
password=password,
host=host,
database=database
)
return conn
except mysql.connector.Error as err:
print(f"Error: {err}")
return None
1.3 使用 psycopg2 连接 PostgreSQL 数据库
import psycopg2
def connect_to_postgresql(user, password, host, database):
try:
conn = psycopg2.connect(
user=user,
password=password,
host=host,
database=database
)
return conn
except psycopg2.Error as e:
print(f"Error: {e}")
return None
二、获取表信息
在删除列之前,获取表的结构信息是非常重要的,这样可以确保删除操作不会破坏表的完整性。
2.1 获取 SQLite 数据库表结构
def get_table_info_sqlite(conn, table_name):
cursor = conn.cursor()
cursor.execute(f"PRAGMA table_info({table_name})")
columns = cursor.fetchall()
return columns
2.2 获取 MySQL 数据库表结构
def get_table_info_mysql(conn, table_name):
cursor = conn.cursor()
cursor.execute(f"DESCRIBE {table_name}")
columns = cursor.fetchall()
return columns
2.3 获取 PostgreSQL 数据库表结构
def get_table_info_postgresql(conn, table_name):
cursor = conn.cursor()
cursor.execute(f"""
SELECT column_name
FROM information_schema.columns
WHERE table_name = '{table_name}'
""")
columns = cursor.fetchall()
return columns
三、删除列
删除列的方式因数据库类型而异。通常需要创建一个临时表,然后将数据复制到新表中,最后重命名新表。
3.1 删除 SQLite 数据库中的列
SQLite 不支持直接删除列,需要手动创建新表。
def remove_first_column_sqlite(conn, table_name):
cursor = conn.cursor()
columns = get_table_info_sqlite(conn, table_name)
if not columns:
print("Table not found")
return
column_names = [col[1] for col in columns[1:]]
columns_string = ", ".join(column_names)
cursor.execute(f"CREATE TABLE {table_name}_temp AS SELECT {columns_string} FROM {table_name}")
cursor.execute(f"DROP TABLE {table_name}")
cursor.execute(f"ALTER TABLE {table_name}_temp RENAME TO {table_name}")
conn.commit()
3.2 删除 MySQL 数据库中的列
MySQL 支持使用 ALTER TABLE
语句删除列。
def remove_first_column_mysql(conn, table_name):
cursor = conn.cursor()
columns = get_table_info_mysql(conn, table_name)
if not columns:
print("Table not found")
return
first_column = columns[0][0]
cursor.execute(f"ALTER TABLE {table_name} DROP COLUMN {first_column}")
conn.commit()
3.3 删除 PostgreSQL 数据库中的列
PostgreSQL 也支持使用 ALTER TABLE
语句删除列。
def remove_first_column_postgresql(conn, table_name):
cursor = conn.cursor()
columns = get_table_info_postgresql(conn, table_name)
if not columns:
print("Table not found")
return
first_column = columns[0][0]
cursor.execute(f"ALTER TABLE {table_name} DROP COLUMN {first_column}")
conn.commit()
四、更新表结构
删除列后,需要确保表的结构更新正确。通常情况下,删除列后无需额外操作,但如果有外键约束或索引,可能需要进一步处理。
4.1 更新 SQLite 表结构
在 SQLite 中,更新表结构通常意味着确保临时表和原表一致。
def update_table_structure_sqlite(conn, table_name):
cursor = conn.cursor()
cursor.execute(f"PRAGMA table_info({table_name})")
columns = cursor.fetchall()
print(f"Updated table structure for {table_name}:")
for col in columns:
print(col)
4.2 更新 MySQL 表结构
在 MySQL 中,可以使用 DESCRIBE
命令检查表结构。
def update_table_structure_mysql(conn, table_name):
cursor = conn.cursor()
cursor.execute(f"DESCRIBE {table_name}")
columns = cursor.fetchall()
print(f"Updated table structure for {table_name}:")
for col in columns:
print(col)
4.3 更新 PostgreSQL 表结构
在 PostgreSQL 中,可以查询 information_schema
来检查表结构。
def update_table_structure_postgresql(conn, table_name):
cursor = conn.cursor()
cursor.execute(f"""
SELECT column_name
FROM information_schema.columns
WHERE table_name = '{table_name}'
""")
columns = cursor.fetchall()
print(f"Updated table structure for {table_name}:")
for col in columns:
print(col)
五、示例应用
为了更清晰地展示上述步骤,下面提供了一个完整的示例应用。
5.1 SQLite 示例应用
db_name = 'example.db'
table_name = 'example_table'
conn = connect_to_sqlite(db_name)
if conn:
remove_first_column_sqlite(conn, table_name)
update_table_structure_sqlite(conn, table_name)
conn.close()
5.2 MySQL 示例应用
user = 'user'
password = 'password'
host = 'localhost'
database = 'example_db'
table_name = 'example_table'
conn = connect_to_mysql(user, password, host, database)
if conn:
remove_first_column_mysql(conn, table_name)
update_table_structure_mysql(conn, table_name)
conn.close()
5.3 PostgreSQL 示例应用
user = 'user'
password = 'password'
host = 'localhost'
database = 'example_db'
table_name = 'example_table'
conn = connect_to_postgresql(user, password, host, database)
if conn:
remove_first_column_postgresql(conn, table_name)
update_table_structure_postgresql(conn, table_name)
conn.close()
以上步骤详细介绍了如何使用Python删除数据库中的第一列,包括连接数据库、获取表信息、删除列和更新表结构。不同数据库有不同的处理方法,但核心思想是一致的。希望这篇文章能帮助你更好地理解和操作数据库列的删除。
相关问答FAQs:
如何在Python中连接数据库以进行操作?
在Python中,可以使用多种库连接数据库,如SQLite、MySQL、PostgreSQL等。对于SQLite,可以使用内置的sqlite3库。首先,需要导入sqlite3模块,然后使用connect()
方法连接到数据库,接着可以创建一个游标对象来执行SQL语句。
删除数据库中的一列会影响其他列吗?
删除一列通常不会直接影响其他列的数据,但需要注意的是,相关的约束条件可能会受到影响。如果存在外键约束或触发器,删除该列可能会导致错误。因此,确保在进行操作前备份数据,并检查数据库结构。
在删除列之前,如何检查数据库表的结构?
可以使用SQL语句PRAGMA table_info(table_name);
(对于SQLite)或DESCRIBE table_name;
(对于MySQL)来查看表结构。这些命令将列出表中的所有列及其数据类型和约束条件,有助于了解删除操作的潜在影响。
删除列后,如何验证操作是否成功?
在删除列之后,可以再次使用查看表结构的SQL命令来确认该列已被成功删除。此外,通过执行简单的查询来确保其他数据的完整性和准确性,确保没有影响到其他列的数据。
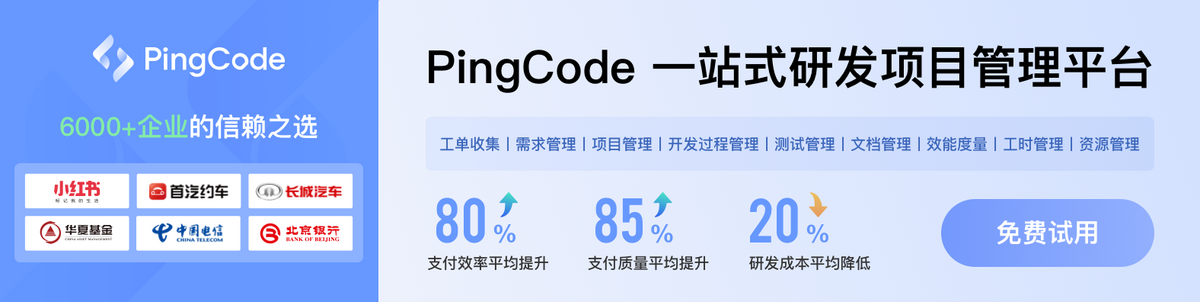