Python3可以通过多种方式下载和保存网络地址图片,使用requests库、使用urllib库、使用Pillow库处理图像。使用requests库是最常见和简便的方法,只需几行代码即可完成图片的下载和保存。具体来说,可以通过以下步骤来实现:首先,导入requests库;其次,使用requests.get()方法获取图片的二进制数据;最后,将获取到的数据写入文件中并保存。下面将详细介绍这些方法及其实现步骤。
一、使用requests库下载和保存图片
requests库是Python中一个非常强大且简单易用的HTTP库。它不仅可以轻松地发送HTTP请求,还可以处理响应,包括下载文件。下面是使用requests库下载和保存图片的详细步骤:
1.1 安装requests库
在使用requests库之前,需要先安装它。可以通过以下命令安装:
pip install requests
1.2 下载图片并保存
以下是使用requests库下载并保存图片的代码示例:
import requests
url = 'https://example.com/image.jpg'
response = requests.get(url)
with open('image.jpg', 'wb') as file:
file.write(response.content)
核心步骤包括:
- 使用requests.get()方法发送HTTP GET请求,获取图片数据。
- 使用
response.content
获取响应内容,即图片的二进制数据。 - 使用
open()
函数以写入二进制模式('wb')打开一个文件。 - 使用
file.write()
方法将图片数据写入文件。
1.3 处理异常情况
在实际应用中,需要处理可能出现的异常情况,例如网络错误、URL无效等。以下是一个更健壮的代码示例:
import requests
def download_image(url, save_path):
try:
response = requests.get(url, timeout=10)
response.raise_for_status() # 检查请求是否成功
with open(save_path, 'wb') as file:
file.write(response.content)
print(f'Image successfully downloaded: {save_path}')
except requests.RequestException as e:
print(f'Failed to download image: {e}')
url = 'https://example.com/image.jpg'
download_image(url, 'image.jpg')
在这个示例中,使用了response.raise_for_status()
方法来检查请求是否成功,并使用了requests.RequestException
来捕获所有可能的请求异常。
二、使用urllib库下载和保存图片
urllib库是Python内置的一个用于处理URL的模块,它提供了一些用于打开和读取URL的函数。尽管urllib库的使用稍显复杂,但它不需要额外安装第三方库。
2.1 使用urllib.request模块
以下是使用urllib库下载并保存图片的代码示例:
import urllib.request
url = 'https://example.com/image.jpg'
save_path = 'image.jpg'
urllib.request.urlretrieve(url, save_path)
print(f'Image successfully downloaded: {save_path}')
核心步骤包括:
- 使用
urllib.request.urlretrieve()
函数下载图片。 - 指定URL和保存路径。
2.2 使用urllib.request模块的高级用法
可以使用更高级的用法来处理下载过程中的异常情况:
import urllib.request
def download_image(url, save_path):
try:
with urllib.request.urlopen(url) as response:
with open(save_path, 'wb') as file:
file.write(response.read())
print(f'Image successfully downloaded: {save_path}')
except urllib.error.URLError as e:
print(f'Failed to download image: {e}')
url = 'https://example.com/image.jpg'
download_image(url, 'image.jpg')
在这个示例中,使用了urllib.request.urlopen()
函数打开URL,并使用response.read()
方法读取图片数据,同时使用了urllib.error.URLError
来捕获URL相关的异常。
三、使用Pillow库处理图像
Pillow库是Python中的一个强大的图像处理库,它不仅可以处理图像的打开、保存和转换,还可以进行复杂的图像操作。
3.1 安装Pillow库
在使用Pillow库之前,需要先安装它。可以通过以下命令安装:
pip install pillow
3.2 下载并保存图片
以下是使用Pillow库下载并保存图片的代码示例:
import requests
from PIL import Image
from io import BytesIO
url = 'https://example.com/image.jpg'
response = requests.get(url)
image = Image.open(BytesIO(response.content))
image.save('image.jpg')
print('Image successfully downloaded and saved.')
核心步骤包括:
- 使用requests库下载图片数据。
- 使用
BytesIO
将图片数据转换为字节流。 - 使用
Image.open()
方法打开图片。 - 使用
image.save()
方法保存图片。
3.3 处理不同格式的图像
Pillow库支持多种图像格式,可以轻松进行格式转换。例如,将下载的图片保存为PNG格式:
import requests
from PIL import Image
from io import BytesIO
url = 'https://example.com/image.jpg'
response = requests.get(url)
image = Image.open(BytesIO(response.content))
image.save('image.png')
print('Image successfully downloaded and saved as PNG.')
四、综合使用多种库进行高级操作
在实际项目中,可能需要综合使用多种库来完成更为复杂的任务。例如,下载图片后对其进行处理并保存。下面是一个综合使用requests库和Pillow库的示例:
4.1 下载图片并转换为灰度图像
import requests
from PIL import Image, ImageFilter
from io import BytesIO
def download_and_process_image(url, save_path):
try:
response = requests.get(url, timeout=10)
response.raise_for_status()
image = Image.open(BytesIO(response.content))
gray_image = image.convert('L') # 转换为灰度图像
gray_image.save(save_path)
print(f'Image successfully downloaded and processed: {save_path}')
except requests.RequestException as e:
print(f'Failed to download image: {e}')
url = 'https://example.com/image.jpg'
download_and_process_image(url, 'gray_image.jpg')
在这个示例中,下载图片后将其转换为灰度图像并保存。
4.2 下载图片并应用滤镜效果
import requests
from PIL import Image, ImageFilter
from io import BytesIO
def download_and_apply_filter(url, save_path):
try:
response = requests.get(url, timeout=10)
response.raise_for_status()
image = Image.open(BytesIO(response.content))
blurred_image = image.filter(ImageFilter.BLUR) # 应用模糊滤镜
blurred_image.save(save_path)
print(f'Image successfully downloaded and blurred: {save_path}')
except requests.RequestException as e:
print(f'Failed to download image: {e}')
url = 'https://example.com/image.jpg'
download_and_apply_filter(url, 'blurred_image.jpg')
在这个示例中,下载图片后应用模糊滤镜效果并保存。
五、总结
通过以上几种方法,我们可以看到Python3中下载和保存网络地址图片的多种实现方式。requests库以其简便性和强大功能广受欢迎;urllib库作为Python内置库,适合不希望依赖第三方库的场景;Pillow库则提供了丰富的图像处理功能,可以满足更多高级需求。
在实际应用中,可以根据具体需求选择合适的方法。如果只是简单地下载图片,requests库无疑是最佳选择;如果需要进行复杂的图像处理,Pillow库则是不可或缺的工具。无论选择哪种方法,都需要注意处理异常情况,以提高代码的鲁棒性和健壮性。
相关问答FAQs:
如何在Python3中下载网络上的图片?
在Python3中下载网络上的图片可以使用requests
库。首先,确保安装了该库,可以通过命令pip install requests
进行安装。下载图片的代码示例如下:
import requests
url = '图片的URL'
response = requests.get(url)
if response.status_code == 200:
with open('保存的文件名.jpg', 'wb') as file:
file.write(response.content)
else:
print("下载失败,状态码:", response.status_code)
使用Python3处理网络图片时,有哪些库可以选择?
除了requests
库,Python3还提供了其他一些库来处理网络图片。例如,urllib
是一个内置库,可以用来下载图片;Pillow
库则可以用于图像处理,支持打开、修改和保存图像格式。选择合适的库取决于具体需求,比如是否需要进行图像处理或简单的下载。
如果下载的图片无法打开,可能是什么原因?
下载的图片无法打开可能有多种原因。例如,下载的内容不是有效的图片格式,或者在下载过程中出现了网络问题。检查HTTP响应状态码,如果不是200,说明请求未成功。此外,确认URL指向的是一个有效的图片文件,确保所保存的文件名和格式与原始图片一致。
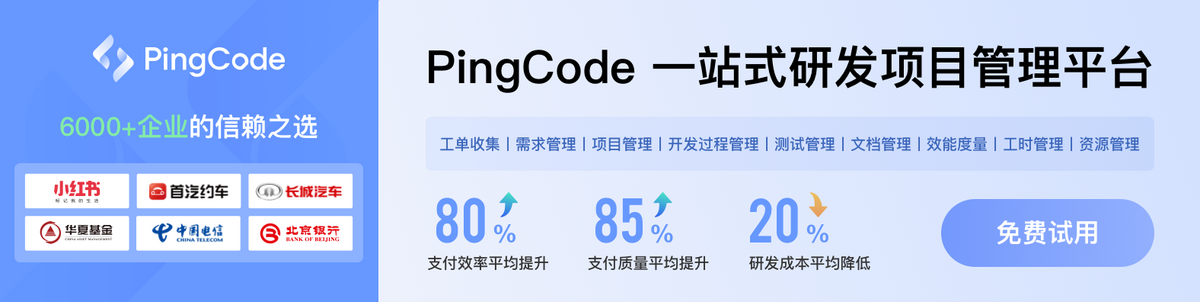