Python读取不同文件夹里的图片,可以通过使用os库遍历文件夹、PIL库或OpenCV库读取图片、glob库匹配文件路径。我们将详细介绍如何使用这些方法来实现这个目标。
一、使用os库遍历文件夹
Python的os库提供了一系列与操作系统交互的功能。我们可以使用os库的os.walk()函数来遍历指定目录及其子目录,并获取其中的所有文件路径。然后,再通过PIL库或OpenCV库读取这些图片文件。
首先,让我们看一下如何使用os库遍历文件夹并获取所有图片文件路径:
import os
def get_image_paths(root_dir, extensions=['.jpg', '.jpeg', '.png', '.bmp']):
image_paths = []
for root, dirs, files in os.walk(root_dir):
for file in files:
if any(file.lower().endswith(ext) for ext in extensions):
image_paths.append(os.path.join(root, file))
return image_paths
root_directory = '/path/to/your/directory'
image_paths = get_image_paths(root_directory)
print(f'Found {len(image_paths)} images')
在这个例子中,我们定义了一个函数get_image_paths()
,它接受一个根目录路径和一个扩展名列表作为参数。这个函数会遍历根目录及其子目录,并将所有符合扩展名的文件路径添加到一个列表中。
二、使用PIL库读取图片
PIL(Python Imaging Library)是一个强大的图像处理库。它可以用于打开、操作和保存许多不同格式的图像文件。我们可以使用PIL库的Image.open()函数来读取图片。
from PIL import Image
def read_images(image_paths):
images = []
for path in image_paths:
try:
img = Image.open(path)
images.append(img)
except Exception as e:
print(f'Error reading {path}: {e}')
return images
images = read_images(image_paths)
print(f'Read {len(images)} images')
在这个例子中,我们定义了一个函数read_images()
,它接受一个图片路径列表作为参数。这个函数会尝试打开每个图片文件,并将成功打开的图片对象添加到一个列表中。如果读取图片时发生错误(例如文件损坏),我们会捕获并打印错误信息。
三、使用OpenCV库读取图片
OpenCV(Open Source Computer Vision Library)是一个开源的计算机视觉和机器学习软件库。它提供了丰富的图像和视频处理功能。我们可以使用OpenCV库的cv2.imread()函数来读取图片。
import cv2
def read_images_opencv(image_paths):
images = []
for path in image_paths:
img = cv2.imread(path)
if img is not None:
images.append(img)
else:
print(f'Error reading {path}')
return images
images_opencv = read_images_opencv(image_paths)
print(f'Read {len(images_opencv)} images with OpenCV')
在这个例子中,我们定义了一个函数read_images_opencv()
,它接受一个图片路径列表作为参数。这个函数会尝试使用OpenCV读取每个图片文件,并将成功读取的图片对象添加到一个列表中。如果读取图片失败,我们会打印错误信息。
四、使用glob库匹配文件路径
glob库提供了一个方便的方法来匹配符合特定模式的文件路径。我们可以使用glob库的glob.glob()函数来获取所有符合指定模式的文件路径。
import glob
def get_image_paths_glob(root_dir, extensions=['.jpg', '.jpeg', '.png', '.bmp']):
image_paths = []
for ext in extensions:
image_paths.extend(glob.glob(os.path.join(root_dir, '', f'*{ext}'), recursive=True))
return image_paths
image_paths_glob = get_image_paths_glob(root_directory)
print(f'Found {len(image_paths_glob)} images with glob')
在这个例子中,我们定义了一个函数get_image_paths_glob()
,它接受一个根目录路径和一个扩展名列表作为参数。这个函数会使用glob.glob()函数匹配符合扩展名的文件路径,并将这些路径添加到一个列表中。
五、综合应用
现在,我们将上述方法综合应用,创建一个完整的解决方案来读取不同文件夹里的图片。我们将使用os库遍历文件夹、PIL库和OpenCV库读取图片、glob库匹配文件路径。
import os
import glob
from PIL import Image
import cv2
def get_image_paths(root_dir, extensions=['.jpg', '.jpeg', '.png', '.bmp']):
image_paths = []
for ext in extensions:
image_paths.extend(glob.glob(os.path.join(root_dir, '', f'*{ext}'), recursive=True))
return image_paths
def read_images_pil(image_paths):
images = []
for path in image_paths:
try:
img = Image.open(path)
images.append(img)
except Exception as e:
print(f'Error reading {path} with PIL: {e}')
return images
def read_images_opencv(image_paths):
images = []
for path in image_paths:
img = cv2.imread(path)
if img is not None:
images.append(img)
else:
print(f'Error reading {path} with OpenCV')
return images
root_directory = '/path/to/your/directory'
image_paths = get_image_paths(root_directory)
print(f'Found {len(image_paths)} images')
images_pil = read_images_pil(image_paths)
print(f'Read {len(images_pil)} images with PIL')
images_opencv = read_images_opencv(image_paths)
print(f'Read {len(images_opencv)} images with OpenCV')
在这个综合应用中,我们首先使用glob库匹配文件路径,然后分别使用PIL库和OpenCV库读取图片。这样,我们可以处理不同文件夹里的图片,并根据需要选择不同的图像处理库。
总结
在这篇文章中,我们详细介绍了如何使用Python读取不同文件夹里的图片。我们首先使用os库遍历文件夹,获取所有图片文件路径。然后,我们分别介绍了如何使用PIL库和OpenCV库读取图片。最后,我们使用glob库匹配文件路径,并将上述方法综合应用,创建一个完整的解决方案。希望这篇文章能帮助您更好地理解和应用Python进行图像处理。
相关问答FAQs:
如何使用Python读取特定文件夹中的所有图片文件?
可以使用Python的os
库结合PIL
(Pillow)库来读取特定文件夹中的所有图片。首先,导入所需的库,使用os.listdir()
函数获取文件夹中的文件列表,然后通过PIL.Image.open()
打开图片文件。示例代码如下:
import os
from PIL import Image
folder_path = '你的文件夹路径'
images = [Image.open(os.path.join(folder_path, file)) for file in os.listdir(folder_path) if file.endswith(('jpg', 'png', 'jpeg'))]
这样就可以读取指定文件夹中的所有图片文件。
Python如何遍历多个文件夹并读取其中的图片?
要遍历多个文件夹,可以使用os.walk()
函数。这将允许你遍历一个目录树,访问所有子文件夹中的文件。结合条件判断,你可以筛选出所需的图片格式。示例代码如下:
import os
from PIL import Image
base_path = '你的根文件夹路径'
for dirpath, dirnames, filenames in os.walk(base_path):
for file in filenames:
if file.endswith(('jpg', 'png', 'jpeg')):
img = Image.open(os.path.join(dirpath, file))
# 处理图片
这种方法可以轻松读取多个文件夹中的图片。
如何处理读取到的图片数据?
读取图片后,可以根据需求进行处理,比如调整大小、转换格式或进行图像增强。使用PIL
库可以实现这些操作。例如,调整图片大小的代码如下:
resized_image = img.resize((width, height))
此外,还可以利用numpy
库对图片进行数值处理,或者使用matplotlib
库进行显示和可视化。这使得读取和处理图片变得非常灵活和强大。
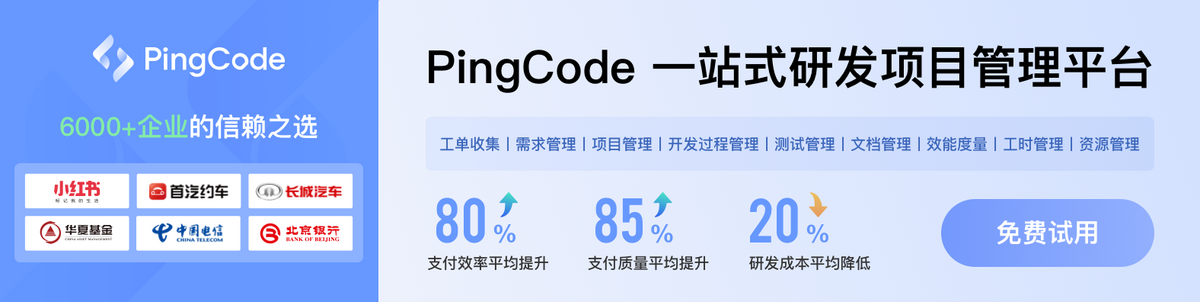