HTML 如何与 Python 连接数据库
在现代Web开发中,HTML、Python 和数据库的结合可以创建动态和交互式网站。首先,你需要使用Python连接数据库,然后通过HTML与Python进行交互。其中一个常用的框架是Flask,它能够让你轻松地将Python代码嵌入到HTML页面中。连接数据库的步骤包括:选择合适的数据库、安装必要的库、编写Python脚本连接数据库、创建API接口、使用HTML与API进行交互。本文将详细介绍这些步骤,并提供相应的代码示例。
一、选择合适的数据库
在选择数据库时,有多种选择,包括关系型数据库(如MySQL、PostgreSQL)和非关系型数据库(如MongoDB)。每种数据库都有其优点和适用场景。对于初学者来说,MySQL和SQLite是很好的选择,因为它们易于使用且有广泛的文档支持。
1.1 关系型数据库
关系型数据库通过表格结构存储数据,常见的有MySQL、PostgreSQL、SQLite等。MySQL和PostgreSQL适合大型项目,SQLite适合小型项目和开发阶段。
1.2 非关系型数据库
非关系型数据库通过键-值对、文档、图等结构存储数据,常见的有MongoDB、Redis等。MongoDB适合需要高扩展性的应用,而Redis通常用于缓存和实时数据处理。
二、安装必要的库
无论选择哪种数据库,都需要安装相应的库来连接数据库。对于MySQL,可以使用mysql-connector-python库;对于SQLite,可以直接使用Python内置的sqlite3库;对于MongoDB,可以使用pymongo库。
2.1 安装MySQL连接库
pip install mysql-connector-python
2.2 安装SQLite连接库
SQLite连接库是Python内置的,无需安装。
2.3 安装MongoDB连接库
pip install pymongo
三、编写Python脚本连接数据库
编写Python脚本以连接数据库并执行基本操作。以下以MySQL和SQLite为例。
3.1 使用MySQL连接数据库
import mysql.connector
def connect_mysql():
try:
connection = mysql.connector.connect(
host='localhost',
database='your_database',
user='your_username',
password='your_password'
)
if connection.is_connected():
print("Connected to MySQL database")
return connection
except mysql.connector.Error as err:
print(f"Error: {err}")
return None
Example usage
connection = connect_mysql()
if connection:
connection.close()
3.2 使用SQLite连接数据库
import sqlite3
def connect_sqlite():
try:
connection = sqlite3.connect('your_database.db')
print("Connected to SQLite database")
return connection
except sqlite3.Error as err:
print(f"Error: {err}")
return None
Example usage
connection = connect_sqlite()
if connection:
connection.close()
四、创建API接口
使用Flask框架创建API接口,使HTML页面可以与Python脚本通信。Flask是一个轻量级的Web框架,非常适合创建API接口。
4.1 安装Flask
pip install flask
4.2 编写Flask应用
以下示例展示如何创建一个简单的Flask应用,并通过API接口与数据库进行交互。
from flask import Flask, request, jsonify
import mysql.connector
app = Flask(__name__)
def connect_mysql():
try:
connection = mysql.connector.connect(
host='localhost',
database='your_database',
user='your_username',
password='your_password'
)
if connection.is_connected():
print("Connected to MySQL database")
return connection
except mysql.connector.Error as err:
print(f"Error: {err}")
return None
@app.route('/data', methods=['GET'])
def get_data():
connection = connect_mysql()
if connection:
cursor = connection.cursor(dictionary=True)
cursor.execute("SELECT * FROM your_table")
rows = cursor.fetchall()
connection.close()
return jsonify(rows)
else:
return jsonify({"error": "Failed to connect to database"}), 500
if __name__ == '__main__':
app.run(debug=True)
五、使用HTML与API进行交互
在HTML页面中使用JavaScript进行API调用,以实现与Python脚本的交互。
5.1 创建HTML页面
以下示例展示如何在HTML页面中使用JavaScript调用Flask API,并显示数据库数据。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Database Connection Example</title>
</head>
<body>
<h1>Database Data</h1>
<div id="data-container"></div>
<script>
fetch('/data')
.then(response => response.json())
.then(data => {
const dataContainer = document.getElementById('data-container');
data.forEach(row => {
const div = document.createElement('div');
div.textContent = JSON.stringify(row);
dataContainer.appendChild(div);
});
})
.catch(error => console.error('Error fetching data:', error));
</script>
</body>
</html>
通过以上步骤,你可以实现HTML与Python连接数据库的基础功能。以下是更详细的内容,涵盖了数据库操作、API设计和前端交互的更多细节。
六、数据库操作的更多细节
在实际应用中,除了简单的查询操作,你还可能需要进行插入、更新和删除操作。
6.1 插入数据
@app.route('/data', methods=['POST'])
def insert_data():
data = request.json
connection = connect_mysql()
if connection:
cursor = connection.cursor()
cursor.execute("INSERT INTO your_table (column1, column2) VALUES (%s, %s)", (data['column1'], data['column2']))
connection.commit()
connection.close()
return jsonify({"message": "Data inserted successfully"}), 201
else:
return jsonify({"error": "Failed to connect to database"}), 500
6.2 更新数据
@app.route('/data/<int:id>', methods=['PUT'])
def update_data(id):
data = request.json
connection = connect_mysql()
if connection:
cursor = connection.cursor()
cursor.execute("UPDATE your_table SET column1 = %s, column2 = %s WHERE id = %s", (data['column1'], data['column2'], id))
connection.commit()
connection.close()
return jsonify({"message": "Data updated successfully"}), 200
else:
return jsonify({"error": "Failed to connect to database"}), 500
6.3 删除数据
@app.route('/data/<int:id>', methods=['DELETE'])
def delete_data(id):
connection = connect_mysql()
if connection:
cursor = connection.cursor()
cursor.execute("DELETE FROM your_table WHERE id = %s", (id,))
connection.commit()
connection.close()
return jsonify({"message": "Data deleted successfully"}), 200
else:
return jsonify({"error": "Failed to connect to database"}), 500
七、API设计的更多细节
API设计不仅仅是实现基本的CRUD操作,还需要考虑安全性、性能和可扩展性。
7.1 安全性
在处理用户输入时,必须进行输入验证和参数化查询,以防止SQL注入和其他安全漏洞。
@app.route('/data', methods=['POST'])
def insert_data():
data = request.json
if 'column1' not in data or 'column2' not in data:
return jsonify({"error": "Invalid input"}), 400
connection = connect_mysql()
if connection:
cursor = connection.cursor()
cursor.execute("INSERT INTO your_table (column1, column2) VALUES (%s, %s)", (data['column1'], data['column2']))
connection.commit()
connection.close()
return jsonify({"message": "Data inserted successfully"}), 201
else:
return jsonify({"error": "Failed to connect to database"}), 500
7.2 性能
为了提高API的性能,可以使用连接池、缓存和索引。
from mysql.connector.pooling import MySQLConnectionPool
pool = MySQLConnectionPool(
pool_name="mypool",
pool_size=10,
host='localhost',
database='your_database',
user='your_username',
password='your_password'
)
def connect_mysql():
return pool.get_connection()
@app.route('/data', methods=['GET'])
def get_data():
connection = connect_mysql()
if connection:
cursor = connection.cursor(dictionary=True)
cursor.execute("SELECT * FROM your_table")
rows = cursor.fetchall()
connection.close()
return jsonify(rows)
else:
return jsonify({"error": "Failed to connect to database"}), 500
7.3 可扩展性
为了提高API的可扩展性,可以使用蓝图和模块化设计。
from flask import Blueprint
data_bp = Blueprint('data', __name__)
@data_bp.route('/data', methods=['GET'])
def get_data():
connection = connect_mysql()
if connection:
cursor = connection.cursor(dictionary=True)
cursor.execute("SELECT * FROM your_table")
rows = cursor.fetchall()
connection.close()
return jsonify(rows)
else:
return jsonify({"error": "Failed to connect to database"}), 500
app.register_blueprint(data_bp)
八、前端交互的更多细节
在前端页面中,可以使用更多的JavaScript库和框架,如jQuery、React 或 Vue.js,以提高交互性和用户体验。
8.1 使用jQuery进行API调用
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Database Connection Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<h1>Database Data</h1>
<div id="data-container"></div>
<script>
$(document).ready(function() {
$.ajax({
url: '/data',
method: 'GET',
success: function(data) {
const dataContainer = $('#data-container');
data.forEach(row => {
const div = $('<div></div>').text(JSON.stringify(row));
dataContainer.append(div);
});
},
error: function(error) {
console.error('Error fetching data:', error);
}
});
});
</script>
</body>
</html>
8.2 使用React进行API调用
import React, { useEffect, useState } from 'react';
import axios from 'axios';
function App() {
const [data, setData] = useState([]);
useEffect(() => {
axios.get('/data')
.then(response => {
setData(response.data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
}, []);
return (
<div>
<h1>Database Data</h1>
<div id="data-container">
{data.map((row, index) => (
<div key={index}>{JSON.stringify(row)}</div>
))}
</div>
</div>
);
}
export default App;
通过上述步骤和示例代码,你可以实现HTML、Python和数据库的连接和交互。在实际开发中,根据项目需求和技术栈选择合适的工具和方法,可以提高开发效率和系统性能。
相关问答FAQs:
如何使用Python与HTML进行数据库交互?
在网页开发中,Python通常作为后端语言与HTML前端结合。常见的做法是使用Flask或Django等框架,将Python代码处理数据库操作,然后将结果通过HTML模板渲染到网页上。您需要在Python中设置数据库连接,执行查询,并将结果传递给HTML模板引擎进行展示。
Python连接数据库时需要哪些库?
为了实现Python与数据库的连接,您可以使用多种库,具体取决于您选择的数据库类型。对于MySQL,可以使用mysql-connector-python
或PyMySQL
;对于PostgreSQL,推荐使用psycopg2
;SQLite则可以直接使用Python自带的sqlite3
模块。这些库提供了丰富的功能来执行SQL命令和处理结果。
如何在HTML中展示来自数据库的数据?
在HTML中展示数据库数据的过程通常涉及到几个步骤。首先,使用Python从数据库中查询所需的数据,并将结果存储在一个变量中。接着,使用模板引擎(如Jinja2)将数据传递到HTML文件中。您可以使用循环结构在HTML中动态生成表格或列表,以展示数据库中的信息,确保页面能够实时反映数据库的更新。
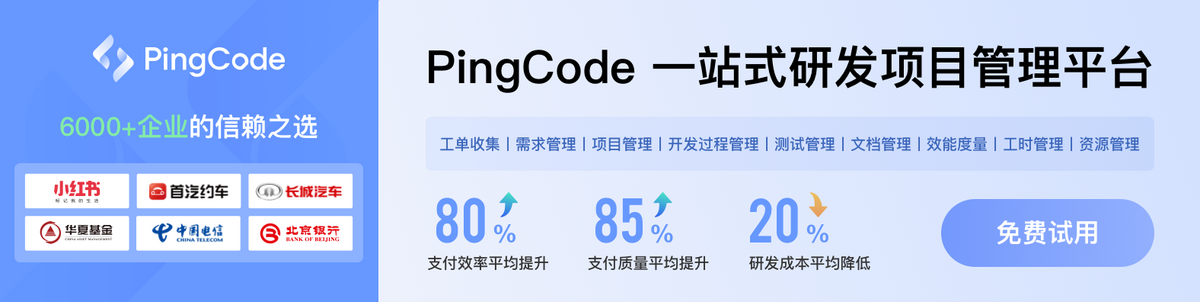