在Python中,一个文件里运行多个代码的方法包括使用函数、类、脚本块、条件语句等。 通过这些方法,可以有效地组织和管理代码,确保其可读性和可维护性。以下详细讨论了这些方法,并提供了实用的示例和最佳实践。
一、使用函数
函数是Python中最常见的代码组织方式之一。将不同的代码块封装在函数中,可以提高代码的重用性和可读性。
1.1 定义与调用函数
函数的定义使用def
关键字,调用函数时使用函数名加括号。
def greet():
print("Hello, World!")
def add(a, b):
return a + b
调用函数
greet()
print(add(3, 4))
1.2 函数的参数与返回值
函数可以接受参数,并返回计算结果。
def multiply(a, b):
return a * b
result = multiply(6, 7)
print(result)
二、使用类
类可以用来封装一组相关的函数和数据,提供一个更高级别的抽象。
2.1 定义类与方法
使用class
关键字定义类,类中的函数称为方法。
class Calculator:
def __init__(self):
self.result = 0
def add(self, a, b):
self.result = a + b
return self.result
def subtract(self, a, b):
self.result = a - b
return self.result
创建类的实例
calc = Calculator()
print(calc.add(10, 5))
print(calc.subtract(10, 5))
2.2 类的属性与实例化
类可以包含属性,实例化类时,属性可以帮助存储和管理数据。
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
创建类的实例
person1 = Person("Alice", 30)
person1.greet()
三、脚本块
在Python文件中,可以使用脚本块直接运行代码。通常,脚本块放在文件的末尾,用于测试或执行主要功能。
3.1 使用if __name__ == "__main__":
这是一个常见的Python惯用法,确保脚本块只有在直接运行文件时才执行。
def main():
print("This is the main function.")
if __name__ == "__main__":
main()
四、条件语句
使用条件语句可以控制代码的执行流程,确保不同代码块在不同条件下运行。
4.1 使用if
, elif
, else
条件语句可以根据不同条件执行不同的代码块。
def check_number(num):
if num > 0:
print("The number is positive.")
elif num < 0:
print("The number is negative.")
else:
print("The number is zero.")
check_number(5)
check_number(-3)
check_number(0)
4.2 嵌套条件语句
条件语句可以嵌套,以处理更复杂的逻辑。
def check_age(age):
if age >= 18:
if age >= 65:
print("You are a senior citizen.")
else:
print("You are an adult.")
else:
print("You are a minor.")
check_age(20)
check_age(70)
check_age(15)
五、模块与包
当代码量较大时,可以将代码拆分成多个模块和包,进一步组织和管理。
5.1 创建与导入模块
模块是一个Python文件,可以包含函数、类和变量。使用import
关键字导入模块。
# 创建一个模块 math_operations.py
def add(a, b):
return a + b
def subtract(a, b):
return a - b
导入并使用模块
import math_operations
print(math_operations.add(5, 3))
print(math_operations.subtract(5, 3))
5.2 创建与导入包
包是包含多个模块的文件夹,文件夹内必须包含一个__init__.py
文件。
# 创建一个包 my_package
文件结构
my_package/
├── __init__.py
├── module1.py
└── module2.py
module1.py
def func1():
print("Function 1 in module 1")
module2.py
def func2():
print("Function 2 in module 2")
导入并使用包
from my_package import module1, module2
module1.func1()
module2.func2()
六、使用外部库
Python有丰富的第三方库,可以帮助实现复杂的功能。使用pip
安装外部库,然后在代码中导入和使用。
6.1 安装与导入外部库
以requests
库为例,展示如何安装和使用外部库。
pip install requests
import requests
response = requests.get("https://api.github.com")
print(response.status_code)
print(response.json())
七、测试与调试
为确保代码的正确性和性能,测试与调试是必不可少的步骤。
7.1 使用unittest
进行单元测试
unittest
是Python自带的测试框架,可以帮助编写和运行测试用例。
import unittest
def add(a, b):
return a + b
class TestMathOperations(unittest.TestCase):
def test_add(self):
self.assertEqual(add(3, 4), 7)
self.assertEqual(add(-1, 1), 0)
if __name__ == "__main__":
unittest.main()
7.2 使用logging
进行调试
logging
模块提供了灵活的日志记录功能,帮助调试和跟踪代码执行。
import logging
logging.basicConfig(level=logging.DEBUG)
def divide(a, b):
try:
result = a / b
logging.debug(f"Result of {a} / {b} is {result}")
return result
except ZeroDivisionError:
logging.error("Division by zero error")
return None
divide(10, 2)
divide(10, 0)
八、性能优化
性能优化是提高代码效率和响应速度的重要手段。
8.1 使用timeit
模块测量性能
timeit
模块可以精确测量代码的执行时间,帮助发现性能瓶颈。
import timeit
def test_function():
total = 0
for i in range(1000):
total += i
return total
execution_time = timeit.timeit(test_function, number=1000)
print(f"Execution time: {execution_time} seconds")
8.2 使用cProfile
进行性能分析
cProfile
模块可以生成详细的性能分析报告,帮助优化代码。
import cProfile
def test_function():
total = 0
for i in range(1000):
total += i
return total
cProfile.run('test_function()')
九、并行与并发
并行与并发可以显著提高代码的执行效率,尤其是处理I/O密集型任务时。
9.1 使用threading
进行多线程编程
threading
模块可以创建和管理多个线程,实现并发执行。
import threading
def print_numbers():
for i in range(5):
print(i)
thread1 = threading.Thread(target=print_numbers)
thread2 = threading.Thread(target=print_numbers)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
9.2 使用multiprocessing
进行多进程编程
multiprocessing
模块可以创建和管理多个进程,实现并行执行。
import multiprocessing
def print_numbers():
for i in range(5):
print(i)
process1 = multiprocessing.Process(target=print_numbers)
process2 = multiprocessing.Process(target=print_numbers)
process1.start()
process2.start()
process1.join()
process2.join()
十、文件与输入输出
文件与输入输出操作是Python编程中常见的任务,可以使用内置的open
函数和其他I/O模块。
10.1 文件读写
使用open
函数可以轻松实现文件的读写操作。
# 写入文件
with open("example.txt", "w") as file:
file.write("Hello, World!")
读取文件
with open("example.txt", "r") as file:
content = file.read()
print(content)
10.2 使用csv
模块处理CSV文件
csv
模块提供了读写CSV文件的简便方法。
import csv
写入CSV文件
with open("data.csv", "w", newline="") as file:
writer = csv.writer(file)
writer.writerow(["Name", "Age", "City"])
writer.writerow(["Alice", 30, "New York"])
writer.writerow(["Bob", 25, "Los Angeles"])
读取CSV文件
with open("data.csv", "r") as file:
reader = csv.reader(file)
for row in reader:
print(row)
十一、异常处理
异常处理可以捕获和处理代码中的错误,确保程序的稳定性和健壮性。
11.1 使用try
, except
, finally
使用try
块来包围可能引发异常的代码,使用except
块捕获并处理异常,finally
块中的代码无论是否发生异常都会执行。
def divide(a, b):
try:
result = a / b
except ZeroDivisionError:
print("Error: Division by zero")
result = None
finally:
print("Execution completed")
return result
print(divide(10, 2))
print(divide(10, 0))
11.2 自定义异常
可以定义自己的异常类,以便在特定条件下引发和处理异常。
class CustomError(Exception):
pass
def check_value(value):
if value < 0:
raise CustomError("Negative value not allowed")
return value
try:
check_value(-1)
except CustomError as e:
print(f"CustomError occurred: {e}")
通过以上多种方法,可以在Python文件中运行多个代码块,并有效地组织和管理代码。无论是使用函数、类、脚本块、条件语句,还是模块与包,都可以提高代码的可读性、可维护性和可扩展性。希望这些示例和最佳实践对你有所帮助。
相关问答FAQs:
如何在一个Python文件中组织和运行多个代码块?
在Python文件中,您可以通过定义多个函数、类或直接编写代码块来组织和运行多个代码。使用函数来封装每个代码块,不仅可以提高代码的可读性,还便于重用。可以在文件末尾调用这些函数来执行代码。
Python文件中可以使用哪些结构来分隔不同的代码?
您可以使用函数、类、模块和注释来分隔不同的代码。通过使用函数,可以将特定功能的代码封装在一起;类则适用于需要维护状态的复杂代码;模块可以将代码逻辑分开,使其更易于管理和重用。注释可以帮助你清晰地说明每个部分的功能。
在一个Python文件中如何管理变量和数据的作用域?
在Python中,变量的作用域由其定义的位置决定。全局变量在文件的任何地方都可以访问,而局部变量仅在函数或类的内部可用。为了避免变量冲突,建议使用局部变量,并尽量在函数内部进行数据处理,这样可以提高代码的可维护性和可读性。
如何在Python文件中调试多个代码块?
调试多个代码块可以通过使用内置的print()
函数来检查变量值,或者使用Python的调试工具,如pdb
模块。通过设置断点,您可以逐步执行代码,查看每个代码块的执行情况,从而找出潜在的问题。此外,使用集成开发环境(IDE)中的调试功能也能大幅提升调试效率。
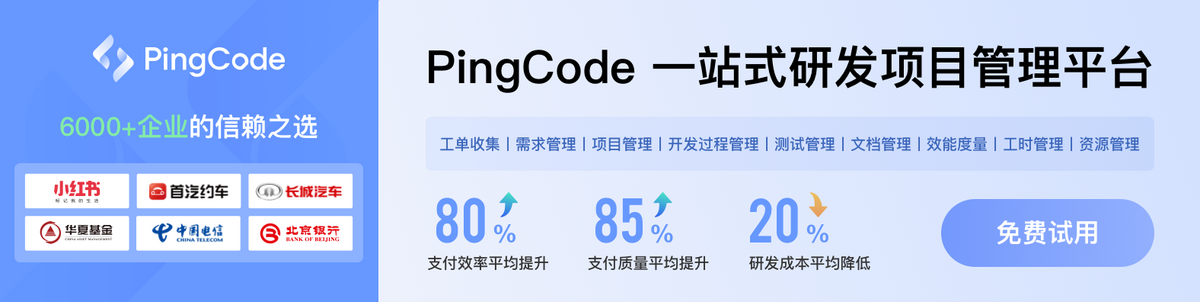