在Python中创建绝对路径的文件涉及以下关键步骤:使用os
和pathlib
模块、确保路径存在、正确处理文件名。使用os
模块、利用pathlib
模块、创建路径、处理文件名是关键点。下面详细描述如何使用pathlib
模块来创建绝对路径的文件。
Python 的 pathlib
模块提供了一个面向对象的路径操作方法。通过使用该模块,可以更方便地处理文件路径。以下是具体步骤:
from pathlib import Path
定义目标路径和文件名
directory = '/absolute/path/to/directory'
filename = 'myfile.txt'
创建目录路径对象
path = Path(directory)
如果目录不存在,创建目录
path.mkdir(parents=True, exist_ok=True)
创建文件路径对象
file_path = path / filename
创建文件并写入内容
file_path.write_text("This is a sample text.")
一、使用os
模块
Python 的 os
模块提供了处理文件和目录的函数,包括创建目录、检查路径是否存在等。以下是使用os
模块创建绝对路径文件的方法:
import os
定义目标路径和文件名
directory = '/absolute/path/to/directory'
filename = 'myfile.txt'
如果目录不存在,创建目录
if not os.path.exists(directory):
os.makedirs(directory)
创建文件路径
file_path = os.path.join(directory, filename)
创建文件并写入内容
with open(file_path, 'w') as file:
file.write("This is a sample text.")
二、使用pathlib
模块
Python 3.4引入的 pathlib
模块提供了面向对象的路径操作方法。以下是使用pathlib
模块创建绝对路径文件的方法:
from pathlib import Path
定义目标路径和文件名
directory = '/absolute/path/to/directory'
filename = 'myfile.txt'
创建目录路径对象
path = Path(directory)
如果目录不存在,创建目录
path.mkdir(parents=True, exist_ok=True)
创建文件路径对象
file_path = path / filename
创建文件并写入内容
file_path.write_text("This is a sample text.")
三、路径处理
在创建绝对路径文件时,处理路径是一个重要步骤。确保路径存在并正确处理文件名是关键。以下是一些路径处理的最佳实践:
1. 确保路径存在
在创建文件前,必须确保目标路径存在。如果路径不存在,可以使用 os.makedirs()
或 pathlib.Path.mkdir()
方法创建路径。
2. 处理文件名
在创建文件时,必须正确处理文件名。可以使用 os.path.join()
或 pathlib.Path
对象来创建文件路径。
3. 检查文件是否存在
在创建文件前,检查文件是否存在是一个好习惯。可以使用 os.path.exists()
或 pathlib.Path.exists()
方法检查文件是否存在。
import os
from pathlib import Path
定义目标路径和文件名
directory = '/absolute/path/to/directory'
filename = 'myfile.txt'
创建目录路径对象
path = Path(directory)
如果目录不存在,创建目录
path.mkdir(parents=True, exist_ok=True)
创建文件路径对象
file_path = path / filename
检查文件是否存在
if not file_path.exists():
# 创建文件并写入内容
file_path.write_text("This is a sample text.")
else:
print("File already exists.")
四、异常处理
在创建文件时,处理异常是一个必要步骤。常见的异常包括路径不存在、权限不足等。可以使用 try...except
语句捕获并处理异常。
import os
from pathlib import Path
定义目标路径和文件名
directory = '/absolute/path/to/directory'
filename = 'myfile.txt'
try:
# 创建目录路径对象
path = Path(directory)
# 如果目录不存在,创建目录
path.mkdir(parents=True, exist_ok=True)
# 创建文件路径对象
file_path = path / filename
# 检查文件是否存在
if not file_path.exists():
# 创建文件并写入内容
file_path.write_text("This is a sample text.")
else:
print("File already exists.")
except Exception as e:
print(f"An error occurred: {e}")
五、跨平台兼容性
在处理文件路径时,跨平台兼容性是一个重要考虑因素。不同操作系统的路径格式不同,因此在处理路径时需要考虑跨平台兼容性。可以使用 os.path
模块的函数来处理路径,以确保跨平台兼容性。
import os
定义目标路径和文件名
directory = os.path.join(os.sep, 'absolute', 'path', 'to', 'directory')
filename = 'myfile.txt'
如果目录不存在,创建目录
if not os.path.exists(directory):
os.makedirs(directory)
创建文件路径
file_path = os.path.join(directory, filename)
创建文件并写入内容
with open(file_path, 'w') as file:
file.write("This is a sample text.")
通过以上方法,可以在Python中轻松创建绝对路径的文件。确保路径存在、正确处理文件名、异常处理和跨平台兼容性是关键步骤。希望这篇文章对你有所帮助。
相关问答FAQs:
如何在Python中获取当前工作目录并创建绝对路径的文件?
在Python中,可以使用os
模块的getcwd()
方法获取当前工作目录。结合os.path.join()
方法,可以轻松地创建一个绝对路径。例如,使用如下代码可以实现:
import os
current_directory = os.getcwd()
file_name = "example.txt"
absolute_path = os.path.join(current_directory, file_name)
with open(absolute_path, 'w') as file:
file.write("Hello, World!")
以上代码会在当前工作目录下创建一个名为example.txt
的文件,其绝对路径为current_directory/example.txt
。
如何在Python中使用绝对路径创建文件而不依赖当前工作目录?
在Python中,可以直接指定绝对路径而不必依赖当前工作目录。只需在打开文件时提供完整的路径,例如:
absolute_path = "/path/to/your/directory/example.txt"
with open(absolute_path, 'w') as file:
file.write("Hello, World!")
确保替换/path/to/your/directory/
为实际的目标路径。这样,您可以在任何指定的目录中创建文件。
在Python中如何处理文件路径以保证跨平台兼容性?
为了确保代码在不同操作系统间的兼容性,建议使用os.path
模块中的join()
和normpath()
方法。通过这些方法,可以避免因路径分隔符不同而导致的错误。例如:
import os
directory = "C:\\Users\\YourUsername\\Documents" # Windows示例
file_name = "example.txt"
absolute_path = os.path.normpath(os.path.join(directory, file_name))
with open(absolute_path, 'w') as file:
file.write("Hello, World!")
使用normpath()
可以处理路径分隔符的问题,确保在Windows和Unix/Linux系统上都能正确运行。
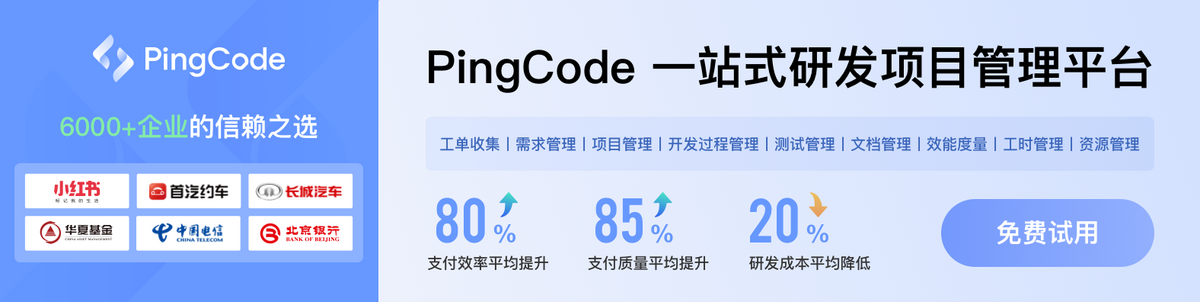