如何用Python做一个贾维斯
利用Python构建一个贾维斯(Jarvis)人工智能助手涉及到多个技术领域和工具,包括语音识别、自然语言处理、文本到语音转换、任务自动化等。关键步骤包括:语音识别、自然语言处理、执行任务、文本到语音转换。其中,语音识别是整个流程的基础,可以使用Google Speech Recognition API来实现。
构建贾维斯的过程可以分为几个主要部分:获取用户输入、解析输入、执行任务、提供反馈。以下是详细的步骤和实现方法。
一、获取用户输入
获取用户输入是贾维斯的第一步,通常通过麦克风获取语音输入。Python的speech_recognition
库可以帮助我们实现这一点。
安装和配置
首先,需要安装speech_recognition
库:
pip install SpeechRecognition
还需要安装pyaudio
库来访问麦克风:
pip install pyaudio
然后,我们可以编写一个简单的代码来捕获语音输入并将其转换为文本:
import speech_recognition as sr
def get_audio():
recognizer = sr.Recognizer()
with sr.Microphone() as source:
print("Listening...")
audio = recognizer.listen(source)
try:
print("Recognizing...")
text = recognizer.recognize_google(audio)
print(f"User said: {text}")
except Exception as e:
print("Sorry, could not recognize what you said.")
return None
return text
user_input = get_audio()
在这个代码段中,我们使用了speech_recognition
库来监听麦克风输入,并使用Google的语音识别服务将语音转换为文本。
二、自然语言处理
获得用户输入后,下一步是解析用户的请求。自然语言处理(NLP)是处理和理解用户意图的关键步骤。可以使用nltk
、spaCy
等库来处理和理解自然语言。
安装和配置
首先,需要安装nltk
库:
pip install nltk
或者安装spaCy
库:
pip install spacy
python -m spacy download en_core_web_sm
处理用户请求
使用nltk
处理用户请求的一个简单示例:
import nltk
from nltk.tokenize import word_tokenize
def process_text(text):
tokens = word_tokenize(text)
print(tokens)
user_input = "What is the weather today?"
process_text(user_input)
或者使用spaCy
来解析用户请求:
import spacy
nlp = spacy.load("en_core_web_sm")
def process_text(text):
doc = nlp(text)
for token in doc:
print(token.text, token.pos_, token.dep_)
user_input = "What is the weather today?"
process_text(user_input)
通过这些步骤,我们可以将用户输入的文本进行分词、词性标注等处理,从而更好地理解用户的意图。
三、执行任务
根据用户的请求执行相应的任务。例如,如果用户询问天气,我们可以使用某些API来获取天气信息;如果用户想要打开某个程序,我们可以使用系统命令来实现。
获取天气信息
使用requests
库和一个天气API来获取天气信息:
import requests
def get_weather(city):
api_key = "your_api_key"
base_url = "http://api.openweathermap.org/data/2.5/weather?"
complete_url = base_url + "appid=" + api_key + "&q=" + city
response = requests.get(complete_url)
data = response.json()
if data["cod"] != "404":
main = data["main"]
weather = data["weather"]
temperature = main["temp"]
weather_description = weather[0]["description"]
return f"Temperature: {temperature}\nDescription: {weather_description}"
else:
return "City Not Found"
city = "London"
print(get_weather(city))
打开程序
使用os
库来执行系统命令:
import os
def open_program(program_name):
if program_name == "notepad":
os.system("notepad")
elif program_name == "calculator":
os.system("calc")
else:
print("Program not recognized")
open_program("notepad")
通过这些步骤,我们可以根据用户的请求执行相应的任务。
四、文本到语音转换
最后一步是将结果反馈给用户。我们可以使用pyttsx3
库将文本转换为语音。
安装和配置
首先,需要安装pyttsx3
库:
pip install pyttsx3
转换文本到语音
使用pyttsx3
库将文本转换为语音:
import pyttsx3
def speak(text):
engine = pyttsx3.init()
engine.say(text)
engine.runAndWait()
speak("Hello, I am Jarvis. How can I assist you today?")
通过这些步骤,我们可以将文本转换为语音并反馈给用户。
五、整合所有部分
将所有部分整合到一起,构建一个完整的贾维斯助手。以下是一个完整的示例代码:
import speech_recognition as sr
import pyttsx3
import requests
import os
def get_audio():
recognizer = sr.Recognizer()
with sr.Microphone() as source:
print("Listening...")
audio = recognizer.listen(source)
try:
print("Recognizing...")
text = recognizer.recognize_google(audio)
print(f"User said: {text}")
except Exception as e:
print("Sorry, could not recognize what you said.")
return None
return text
def process_text(text):
if "weather" in text:
city = text.split("in")[-1].strip()
return get_weather(city)
elif "open" in text:
program_name = text.split("open")[-1].strip()
open_program(program_name)
return f"Opening {program_name}"
else:
return "I am not sure how to help with that."
def get_weather(city):
api_key = "your_api_key"
base_url = "http://api.openweathermap.org/data/2.5/weather?"
complete_url = base_url + "appid=" + api_key + "&q=" + city
response = requests.get(complete_url)
data = response.json()
if data["cod"] != "404":
main = data["main"]
weather = data["weather"]
temperature = main["temp"]
weather_description = weather[0]["description"]
return f"Temperature: {temperature}\nDescription: {weather_description}"
else:
return "City Not Found"
def open_program(program_name):
if program_name == "notepad":
os.system("notepad")
elif program_name == "calculator":
os.system("calc")
else:
print("Program not recognized")
def speak(text):
engine = pyttsx3.init()
engine.say(text)
engine.runAndWait()
if __name__ == "__main__":
while True:
user_input = get_audio()
if user_input:
response = process_text(user_input)
speak(response)
在这个代码中,我们整合了语音识别、自然语言处理、任务执行和文本到语音转换的所有部分,构建了一个基本的贾维斯助手。
六、进一步优化和扩展
创建一个基本的贾维斯只是第一步,可以通过以下方式进一步优化和扩展:
增加更多功能
可以增加更多的功能,例如设置提醒、发送邮件、播放音乐等。通过集成更多的API和库,可以让贾维斯变得更加智能和实用。
语音识别和处理优化
可以使用更高级的语音识别和处理技术,例如使用深度学习模型来提高准确性。可以使用DeepSpeech
等开源项目来替代Google Speech Recognition API。
自然语言理解
可以使用更高级的自然语言理解技术,例如使用BERT
等预训练模型来更好地理解用户意图。
用户界面
可以为贾维斯增加一个用户界面,例如使用Tkinter
或PyQt
来创建一个图形用户界面,使其更易于使用。
通过不断优化和扩展,可以让贾维斯变得更加智能和强大。构建一个贾维斯不仅可以提高生产力,还可以提升编程技能,是一个非常有趣和有挑战性的项目。
相关问答FAQs:
用Python制作贾维斯的基本步骤是什么?
制作贾维斯的第一步是确定功能需求。可以考虑实现语音识别、自然语言处理和任务自动化等功能。使用像SpeechRecognition库进行语音输入,结合NLTK或spaCy进行语言处理。接下来,选择合适的API来扩展功能,例如天气查询、日历管理等。最后,整合这些功能,通过一个统一的接口来进行交互。
哪些Python库可以帮助我实现贾维斯的功能?
有许多Python库可以帮助您构建贾维斯。例如,SpeechRecognition库可以实现语音识别,Pyttsx3可以用于文本转语音,OpenCV可以处理图像和视频,Flask或Django可以搭建Web接口。对于自然语言处理,使用NLTK或spaCy是个不错的选择。结合这些工具,您可以实现一个功能丰富的虚拟助手。
如何训练贾维斯以提高它的响应能力?
训练贾维斯的关键在于不断收集和分析用户的反馈。可以使用机器学习技术来分析用户的命令和问题,从而改进语音识别和自然语言处理的准确性。通过使用Python的Scikit-learn库,您可以训练模型来识别用户的偏好和习惯。持续更新和优化模型,使得贾维斯能适应用户的需求,提升响应能力。
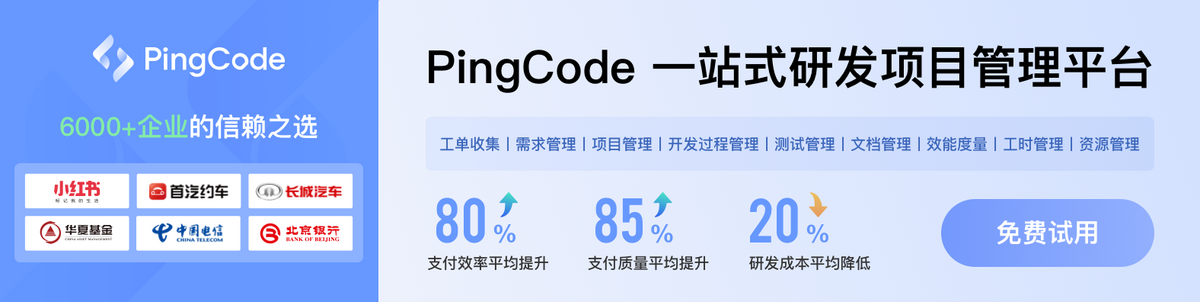